Merge branch 'master' into docking
# Conflicts: # examples/imgui_examples.sln # imgui.cpp # imgui.h # imgui_demo.cpp
This commit is contained in:
commit
3af9ac320d
@ -594,7 +594,7 @@ void ImGui_ImplVulkan_RenderDrawData(ImDrawData* draw_data, VkCommandBuffer comm
|
||||
// Note: at this point both vkCmdSetViewport() and vkCmdSetScissor() have been called.
|
||||
// Our last values will leak into user/application rendering IF:
|
||||
// - Your app uses a pipeline with VK_DYNAMIC_STATE_VIEWPORT or VK_DYNAMIC_STATE_SCISSOR dynamic state
|
||||
// - And you forgot to call vkCmdSetViewport() and vkCmdSetScissor() yourself to explicitely set that state.
|
||||
// - And you forgot to call vkCmdSetViewport() and vkCmdSetScissor() yourself to explicitly set that state.
|
||||
// If you use VK_DYNAMIC_STATE_VIEWPORT or VK_DYNAMIC_STATE_SCISSOR you are responsible for setting the values before rendering.
|
||||
// In theory we should aim to backup/restore those values but I am not sure this is possible.
|
||||
// We perform a call to vkCmdSetScissor() to set back a full viewport which is likely to fix things for 99% users but technically this is not perfect. (See github #4644)
|
||||
|
@ -5,22 +5,22 @@ _(You may browse this at https://github.com/ocornut/imgui/blob/master/docs/BACKE
|
||||
**The backends/ folder contains backends for popular platforms/graphics API, which you can use in
|
||||
your application or engine to easily integrate Dear ImGui.** Each backend is typically self-contained in a pair of files: imgui_impl_XXXX.cpp + imgui_impl_XXXX.h.
|
||||
|
||||
- The 'Platform' backends are in charge of: mouse/keyboard/gamepad inputs, cursor shape, timing, windowing.<BR>
|
||||
- The 'Platform' backends are in charge of: mouse/keyboard/gamepad inputs, cursor shape, timing, and windowing.<BR>
|
||||
e.g. Windows ([imgui_impl_win32.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_win32.cpp)), GLFW ([imgui_impl_glfw.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_glfw.cpp)), SDL2 ([imgui_impl_sdl.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_sdl.cpp)), etc.
|
||||
|
||||
- The 'Renderer' backends are in charge of: creating atlas texture, rendering imgui draw data.<BR>
|
||||
- The 'Renderer' backends are in charge of: creating atlas texture, and rendering imgui draw data.<BR>
|
||||
e.g. DirectX11 ([imgui_impl_dx11.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_dx11.cpp)), OpenGL/WebGL ([imgui_impl_opengl3.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_opengl3.cpp)), Vulkan ([imgui_impl_vulkan.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_vulkan.cpp)), etc.
|
||||
|
||||
- For some high-level frameworks, a single backend usually handle both 'Platform' and 'Renderer' parts.<BR>
|
||||
- For some high-level frameworks, a single backend usually handles both 'Platform' and 'Renderer' parts.<BR>
|
||||
e.g. Allegro 5 ([imgui_impl_allegro5.cpp](https://github.com/ocornut/imgui/blob/master/backends/imgui_impl_allegro5.cpp)). If you end up creating a custom backend for your engine, you may want to do the same.
|
||||
|
||||
An application usually combines 1 Platform backend + 1 Renderer backend + main Dear ImGui sources.
|
||||
An application usually combines one Platform backend + one Renderer backend + main Dear ImGui sources.
|
||||
For example, the [example_win32_directx11](https://github.com/ocornut/imgui/tree/master/examples/example_win32_directx11) application combines imgui_impl_win32.cpp + imgui_impl_dx11.cpp. There are 20+ examples in the [examples/](https://github.com/ocornut/imgui/blob/master/examples/) folder. See [EXAMPLES.MD](https://github.com/ocornut/imgui/blob/master/docs/EXAMPLES.md) for details.
|
||||
|
||||
**Once Dear ImGui is setup and running, run and refer to `ImGui::ShowDemoWindow()` in imgui_demo.cpp for usage of the end-user API.**
|
||||
|
||||
|
||||
### What are backends
|
||||
### What are backends?
|
||||
|
||||
Dear ImGui is highly portable and only requires a few things to run and render, typically:
|
||||
|
||||
@ -41,7 +41,7 @@ Dear ImGui is highly portable and only requires a few things to run and render,
|
||||
This is essentially what each backend is doing + obligatory portability cruft. Using default backends ensure you can get all those features including the ones that would be harder to implement on your side (e.g. multi-viewports support).
|
||||
|
||||
It is important to understand the difference between the core Dear ImGui library (files in the root folder)
|
||||
and backends which we are describing here (backends/ folder).
|
||||
and the backends which we are describing here (backends/ folder).
|
||||
|
||||
- Some issues may only be backend or platform specific.
|
||||
- You should be able to write backends for pretty much any platform and any 3D graphics API.
|
||||
@ -109,19 +109,19 @@ Think twice!
|
||||
If you are new to Dear ImGui, first try using the existing backends as-is.
|
||||
You will save lots of time integrating the library.
|
||||
You can LATER decide to rewrite yourself a custom backend if you really need to.
|
||||
In most situations, custom backends have less features and more bugs than the standard backends we provide.
|
||||
In most situations, custom backends have fewer features and more bugs than the standard backends we provide.
|
||||
If you want portability, you can use multiple backends and choose between them either at compile time
|
||||
or at runtime.
|
||||
|
||||
**Example A**: your engine is built over Windows + DirectX11 but you have your own high-level rendering
|
||||
system layered over DirectX11.<BR>
|
||||
Suggestion: try using imgui_impl_win32.cpp + imgui_impl_dx11.cpp first.
|
||||
Once it works, if you really need it you can replace the imgui_impl_dx11.cpp code with a
|
||||
Once it works, if you really need it, you can replace the imgui_impl_dx11.cpp code with a
|
||||
custom renderer using your own rendering functions, and keep using the standard Win32 code etc.
|
||||
|
||||
**Example B**: your engine runs on Windows, Mac, Linux and uses DirectX11, Metal, Vulkan respectively.<BR>
|
||||
**Example B**: your engine runs on Windows, Mac, Linux and uses DirectX11, Metal, and Vulkan respectively.<BR>
|
||||
Suggestion: use multiple generic backends!
|
||||
Once it works, if you really need it you can replace parts of backends with your own abstractions.
|
||||
Once it works, if you really need it, you can replace parts of backends with your own abstractions.
|
||||
|
||||
**Example C**: your engine runs on platforms we can't provide public backends for (e.g. PS4/PS5, Switch),
|
||||
and you have high-level systems everywhere.<BR>
|
||||
|
@ -127,7 +127,9 @@ Breaking changes:
|
||||
- requires an explicit identifier. You may still use e.g. PushID() calls and then pass an empty identifier.
|
||||
- always uses style.FramePadding for padding, to be consistent with other buttons. You may use PushStyleVar() to alter this.
|
||||
- As always we are keeping a redirection function available (will obsolete later).
|
||||
- Commented out redirecting functions/enums names that were marked obsolete in 1.77 and 1.78 (June 2020): (#3361)
|
||||
- Removed the bizarre legacy default argument for 'TreePush(const void* ptr = NULL)'. (#1057)
|
||||
Must always pass a pointer value explicitly, NULL is ok.
|
||||
- Commented out redirecting functions/enums names that were marked obsolete in 1.77 and 1.78 (June 2020): (#3361)
|
||||
- DragScalar(), DragScalarN(), DragFloat(), DragFloat2(), DragFloat3(), DragFloat4()
|
||||
- SliderScalar(), SliderScalarN(), SliderFloat(), SliderFloat2(), SliderFloat3(), SliderFloat4()
|
||||
- For old signatures ending with (..., const char* format, float power = 1.0f) -> use (..., format ImGuiSliderFlags_Logarithmic) if power != 1.0f.
|
||||
@ -149,6 +151,9 @@ Breaking changes:
|
||||
|
||||
Other Changes:
|
||||
|
||||
- Popups & Modals: fixed nested Begin() being erroneously input-inhibited. While it is
|
||||
unusual, you can nest a Begin() inside a popup or modal, it is occasionally useful to
|
||||
achieve certains things (e.g. some ways to implement suggestion popup #718, #4461).
|
||||
- InputText: added experimental io.ConfigInputTextEnterKeepActive feature to make pressing
|
||||
Enter keep the input active and select all text.
|
||||
- InputText: numerical fields automatically accept full-width characters (U+FF01..U+FF5E)
|
||||
@ -186,8 +191,10 @@ Other Changes:
|
||||
- Debug Tools: Debug Log: Added 'IO' and 'Clipper' events logging.
|
||||
- Debug Tools: Item Picker: Mouse button can be changed by holding Ctrl+Shift, making it easier
|
||||
to use the Item Picker in e.g. menus. (#2673)
|
||||
- Docs: Fixed various typos in comments and documentations. (#5649, #5675, #5679) [@tocic, @lessigsx]
|
||||
- Demo: Improved "Constrained-resizing window" example, more clearly showcase aspect-ratio. (#5627)
|
||||
- Demo: Added more explicit "Center window" mode to "Overlay example". (#5618)
|
||||
- Examples: Added all SDL examples to default VS solution.
|
||||
- Backends: GLFW: Honor GLFW_CURSOR_DISABLED by not setting mouse position. (#5625) [@scorpion-26]
|
||||
- Backends: Metal: Use __bridge for ARC based systems. (#5403) [@stack]
|
||||
- Backends: Metal: Add dispatch synchronization. (#5447) [@luigifcruz]
|
||||
|
@ -14,7 +14,7 @@
|
||||
- Please browse the [Wiki](https://github.com/ocornut/imgui/wiki) to find code snippets, links and other resources (e.g. [Useful extensions](https://github.com/ocornut/imgui/wiki/Useful-Extensions)).
|
||||
- Please read [docs/FAQ.md](https://github.com/ocornut/imgui/blob/master/docs/FAQ.md).
|
||||
- Please read [docs/FONTS.md](https://github.com/ocornut/imgui/blob/master/docs/FONTS.md) if your question relates to fonts or text.
|
||||
- Please read one of the [examples/](https://github.com/ocornut/imgui/tree/master/examples) application if your question relates to setting up dear imgui.
|
||||
- Please read one of the [examples/](https://github.com/ocornut/imgui/tree/master/examples) application if your question relates to setting up Dear ImGui.
|
||||
- Please run `ImGui::ShowDemoWindow()` to explore the demo and its sources.
|
||||
- Please use the search function of your IDE to search in for comments related to your situation.
|
||||
- Please use the search function of GitHub to look for similar issues. You may [browse issues by Labels](https://github.com/ocornut/imgui/labels).
|
||||
@ -24,49 +24,49 @@
|
||||
|
||||
## Issues vs Discussions
|
||||
|
||||
If:
|
||||
- You cannot Build or Link examples.
|
||||
- You cannot Build or Link or Run Dear ImGui in your application or custom engine.
|
||||
- You are failing to load a font.
|
||||
If you:
|
||||
- Cannot BUILD or LINK examples.
|
||||
- Cannot BUILD, or LINK, or RUN Dear ImGui in your application or custom engine.
|
||||
- Cannot LOAD a font.
|
||||
|
||||
Then please [use the Discussions forums](https://github.com/ocornut/imgui/discussions) instead of opening an Issue.
|
||||
Then please [use the Discussions forums](https://github.com/ocornut/imgui/discussions) instead of opening an issue.
|
||||
|
||||
If Dear ImGui is successfully showing in your app and you have used Dear ImGui before, you can open an Issue. Any form of discussions is welcome as a nw Issue.
|
||||
If Dear ImGui is successfully showing in your app and you have used Dear ImGui before, you can open an issue. Any form of discussions is welcome as a new issue.
|
||||
|
||||
## How to open an Issue
|
||||
## How to open an issue
|
||||
|
||||
You may use the Issue Tracker to submit bug reports, feature requests or suggestions. You may ask for help or advice as well. But **PLEASE CAREFULLY READ THIS WALL OF TEXT. ISSUES IGNORING THOSE GUIDELINES MAY BE CLOSED. USERS IGNORING THOSE GUIDELINES MIGHT BE BLOCKED.**
|
||||
|
||||
Please do your best to clarify your request. The amount of incomplete or ambiguous requests due to people not following those guidelines is often overwhelming. Issues created without the requested information may be closed prematurely. Exceptionally entitled, impolite or lazy requests may lead to bans.
|
||||
Please do your best to clarify your request. The amount of incomplete or ambiguous requests due to people not following those guidelines is often overwhelming. Issues created without the requested information may be closed prematurely. Exceptionally entitled, impolite, or lazy requests may lead to bans.
|
||||
|
||||
**PLEASE UNDERSTAND THAT OPEN-SOURCE SOFTWARE LIVES OR DIES BY THE AMOUNT OF ENERGY MAINTAINERS CAN SPARE. WE HAVE LOTS OF STUFF TO DO. THIS IS AN ATTENTION ECONOMY AND MANY LAZY OR MINOR ISSUES ARE HOGGING OUR ATTENTION AND DRAINING ENERGY, TAKING US AWAY FROM MORE IMPORTANT WORK.**
|
||||
|
||||
Steps:
|
||||
|
||||
- Article: [How To Ask Good Questions](https://bit.ly/3nwRnx1).
|
||||
- **PLEASE DO FILL THE REQUESTED NEW ISSUE TEMPLATE.** Including dear imgui version number, branch name, platform/renderer back-ends (imgui_impl_XXX files), operating system.
|
||||
- **Try to be explicit with your Goals, your Expectations and what you have Tried**. Be mindful of [The XY Problem](http://xyproblem.info/). What you have in mind or in your code is not obvious to other people. People frequently discuss problems and suggest incorrect solutions without first clarifying their goal. When requesting a new feature, please describe the usage context (how you intend to use it, why you need it, etc..). If you tried something and it failed, show us what you tried.
|
||||
- **Attach screenshots (or gif/video) to clarify the context**. They often convey useful information that are omitted by the description. You can drag pictures/files in the message edit box. Avoid using 3rd party image hosting services, prefer the long term longevity of GitHub attachments (you can drag pictures into your post). On Windows you can use [ScreenToGif](https://www.screentogif.com/) to easily capture .gif files.
|
||||
- **PLEASE DO FILL THE REQUESTED NEW ISSUE TEMPLATE.** Including Dear ImGui version number, branch name, platform/renderer back-ends (imgui_impl_XXX files), operating system.
|
||||
- **Try to be explicit with your GOALS, your EXPECTATIONS and what you have tried**. Be mindful of [The XY Problem](http://xyproblem.info/). What you have in mind or in your code is not obvious to other people. People frequently discuss problems and suggest incorrect solutions without first clarifying their goals. When requesting a new feature, please describe the usage context (how you intend to use it, why you need it, etc.). If you tried something and it failed, show us what you tried.
|
||||
- **Attach screenshots (or GIF/video) to clarify the context**. They often convey useful information that is omitted by the description. You can drag pictures/files in the message edit box. Avoid using 3rd party image hosting services, prefer the long-term longevity of GitHub attachments (you can drag pictures into your post). On Windows, you can use [ScreenToGif](https://www.screentogif.com/) to easily capture .gif files.
|
||||
- **If you are discussing an assert or a crash, please provide a debugger callstack**. Never state "it crashes" without additional information. If you don't know how to use a debugger and retrieve a callstack, learning about it will be useful.
|
||||
- **Please make sure that your project have asserts enabled.** Calls to IM_ASSERT() are scattered in the code to help catch common issues. When an assert is triggered read the comments around it. By default IM_ASSERT() calls the standard assert() function. To verify that your asserts are enabled, add the line `IM_ASSERT(false);` in your main() function. Your application should display an error message and abort. If your application doesn't report an error, your asserts are disabled.
|
||||
- **Please provide a Minimal, Complete and Verifiable Example ([MCVE](https://stackoverflow.com/help/mcve)) to demonstrate your problem**. An ideal submission includes a small piece of code that anyone can paste in one of the examples/ application (e.g. in main.cpp or imgui_demo.cpp) to understand and reproduce it. Narrowing your problem to its shortest and purest form is the easiest way to understand it. Please test your shortened code to ensure it actually exhibit the problem. **Often while creating the MCVE you will end up solving the problem!** Many questions that are missing a standalone verifiable example are missing the actual cause of their issue in the description, which ends up wasting everyone's time.
|
||||
- Please state if you have made substantial modifications to your copy of imgui or the back-end.
|
||||
- If you are not calling dear imgui directly from C++, please provide information about your Language and the wrapper/binding you are using.
|
||||
- Be mindful that messages are being sent to the mailbox of "Watching" users. Try to proof-read your messages before sending them. Edits are not seen by those users, unless they browse the site.
|
||||
- **Please make sure that your project has asserts enabled.** Calls to IM_ASSERT() are scattered in the code to help catch common issues. When an assert is triggered read the comments around it. By default IM_ASSERT() calls the standard assert() function. To verify that your asserts are enabled, add the line `IM_ASSERT(false);` in your main() function. Your application should display an error message and abort. If your application doesn't report an error, your asserts are disabled.
|
||||
- **Please provide a Minimal, Complete, and Verifiable Example ([MCVE](https://stackoverflow.com/help/mcve)) to demonstrate your problem**. An ideal submission includes a small piece of code that anyone can paste into one of the examples applications (examples/../main.cpp) or demo (imgui_demo.cpp) to understand and reproduce it. Narrowing your problem to its shortest and purest form is the easiest way to understand it. Please test your shortened code to ensure it exhibits the problem. **Often while creating the MCVE you will end up solving the problem!** Many questions that are missing a standalone verifiable example are missing the actual cause of their issue in the description, which ends up wasting everyone's time.
|
||||
- Please state if you have made substantial modifications to your copy of Dear ImGui or the back-end.
|
||||
- If you are not calling Dear ImGui directly from C++, please provide information about your Language and the wrapper/binding you are using.
|
||||
- Be mindful that messages are being sent to the mailbox of "Watching" users. Try to proofread your messages before sending them. Edits are not seen by those users unless they browse the site.
|
||||
|
||||
**Some unfortunate words of warning**
|
||||
- If you are involved in cheating schemes (e.g. DLL injection) for competitive online multi-player games, please don't try posting here. We won't answer and you will be blocked. It doesn't matter if your question relates to said project. We've had too many of you and need to project our time and sanity.
|
||||
- Due to frequent abuse of this service from aforementioned users, if your GitHub account is anonymous and was created five minutes ago please understand that your post will receive more scrutiny and incomplete questions will be harshly dismissed.
|
||||
- If you are involved in cheating schemes (e.g. DLL injection) for competitive online multiplayer games, please don't try posting here. We won't answer and you will be blocked. It doesn't matter if your question relates to said project. We've had too many of you and need to project our time and sanity.
|
||||
- Due to frequent abuse of this service from the aforementioned users, if your GitHub account is anonymous and was created five minutes ago please understand that your post will receive more scrutiny and incomplete questions will be harshly dismissed.
|
||||
|
||||
If you have been using dear imgui for a while or have been using C/C++ for several years or have demonstrated good behavior here, it is ok to not fulfill every item to the letter. Those are guidelines and experienced users or members of the community will know which information is useful in a given context.
|
||||
If you have been using Dear ImGui for a while or have been using C/C++ for several years or have demonstrated good behavior here, it is ok to not fulfill every item to the letter. Those are guidelines and experienced users or members of the community will know which information is useful in a given context.
|
||||
|
||||
## How to open a Pull Request
|
||||
|
||||
- **Please understand that by submitting a PR you are also submitting a request for the maintainer to review your code and then take over its maintenance.** PR should be crafted both in the interest in the end-users and also to ease the maintainer into understanding and accepting it.
|
||||
- Many PR are useful to demonstrate a need and a possible solution but aren't adequate for merging (causing other issues, not seeing other aspects of the big picture, etc.). In doubt, don't hesitate to push a PR because that is always the first step toward finding the mergeable solution! Even if a PR stays unmerged for a long time, its presence can be useful for other users and helps toward finding a general solution.
|
||||
- **Please understand that by submitting a PR you are also submitting a request for the maintainer to review your code and then take over its maintenance.** PR should be crafted both in the interest of the end-users and also to ease the maintainer into understanding and accepting it.
|
||||
- Many PRs are useful to demonstrate a need and a possible solution but aren't adequate for merging (causing other issues, not seeing other aspects of the big picture, etc.). In doubt, don't hesitate to push a PR because that is always the first step toward finding the mergeable solution! Even if a PR stays unmerged for a long time, its presence can be useful for other users and helps toward finding a general solution.
|
||||
- **When adding a feature,** please describe the usage context (how you intend to use it, why you need it, etc.). Be mindful of [The XY Problem](http://xyproblem.info/).
|
||||
- **When fixing a warning or compilation problem,** please post the compiler log and specify the compiler version and platform you are using.
|
||||
- **Attach screenshots (or gif/video) to clarify the context and demonstrate the feature at a glance.** You can drag pictures/files in the message edit box. Prefer the long term longevity of GitHub attachments over 3rd party hosting (you can drag pictures into your post).
|
||||
- **Attach screenshots (or GIF/video) to clarify the context and demonstrate the feature at a glance.** You can drag pictures/files in the message edit box. Prefer the long-term longevity of GitHub attachments over 3rd party hosting (you can drag pictures into your post).
|
||||
- **Make sure your code follows the coding style already used in the codebase:** 4 spaces indentations (no tabs), `local_variable`, `FunctionName()`, `MemberName`, `// Text Comment`, `//CodeComment();`, C-style casts, etc.. We don't use modern C++ idioms and tend to use only a minimum of C++11 features. The applications under examples/ are generally less consistent because they sometimes try to mimic the coding style often adopted by a certain ecosystem (e.g. DirectX-related code tend to use the style of their sample).
|
||||
- **Make sure you create a branch dedicated to the pull request**. In Git, 1 PR is associated to 1 branch. If you keep pushing to the same branch after you submitted the PR, your new commits will appear in the PR (we can still cherry-pick individual commits).
|
||||
|
||||
@ -74,7 +74,7 @@ Thank you for reading!
|
||||
|
||||
## Copyright / Contributor License Agreement
|
||||
|
||||
Any code you submit will become part of the repository and be distributed under the [Dear ImGui license](https://github.com/ocornut/imgui/blob/master/LICENSE.txt). By submitting code to the project you agree that the code is your own work and that you have the ability to give it to the project.
|
||||
Any code you submit will become part of the repository and be distributed under the [Dear ImGui license](https://github.com/ocornut/imgui/blob/master/LICENSE.txt). By submitting code to the project you agree that the code is your work and that you can give it to the project.
|
||||
|
||||
You also agree by submitting your code that you grant all transferrable rights to the code to the project maintainer, including for example re-licensing the code, modifying the code, distributing in source or binary forms. Specifically this includes a requirement that you assign copyright to the project maintainer. For this reason, do not modify any copyright statements in files in any PRs.
|
||||
You also agree by submitting your code that you grant all transferrable rights to the code to the project maintainer, including for example re-licensing the code, modifying the code, and distributing it in source or binary forms. Specifically, this includes a requirement that you assign copyright to the project maintainer. For this reason, do not modify any copyright statements in files in any PRs.
|
||||
|
||||
|
94
docs/FAQ.md
94
docs/FAQ.md
@ -177,7 +177,7 @@ Each draw command needs the triangle rendered using the clipping rectangle provi
|
||||
Rectangles provided by Dear ImGui are defined as
|
||||
`(x1=left,y1=top,x2=right,y2=bottom)`
|
||||
and **NOT** as
|
||||
`(x1,y1,width,height)`
|
||||
`(x1,y1,width,height)`.
|
||||
Refer to rendering backends in the [examples/](https://github.com/ocornut/imgui/tree/master/examples) folder for references of how to handle the `ClipRect` field.
|
||||
|
||||
##### [Return to Index](#index)
|
||||
@ -198,10 +198,10 @@ Dear ImGui internally needs to uniquely identify UI elements.
|
||||
Elements that are typically not clickable (such as calls to the Text functions) don't need an ID.
|
||||
Interactive widgets (such as calls to Button buttons) need a unique ID.
|
||||
|
||||
**Unique ID are used internally to track active widgets and occasionally associate state to widgets.<BR>
|
||||
Unique ID are implicitly built from the hash of multiple elements that identify the "path" to the UI element.**
|
||||
**Unique IDs are used internally to track active widgets and occasionally associate state to widgets.<BR>
|
||||
Unique IDs are implicitly built from the hash of multiple elements that identify the "path" to the UI element.**
|
||||
|
||||
Since Dear ImGui 1.85 you can use `Demo>Tools>Stack Tool` or call `ImGui::ShowStackToolWindow()`. The tool display intermediate values leading to the creation of a unique ID, making things easier to debug and understand.
|
||||
Since Dear ImGui 1.85, you can use `Demo>Tools>Stack Tool` or call `ImGui::ShowStackToolWindow()`. The tool display intermediate values leading to the creation of a unique ID, making things easier to debug and understand.
|
||||
|
||||
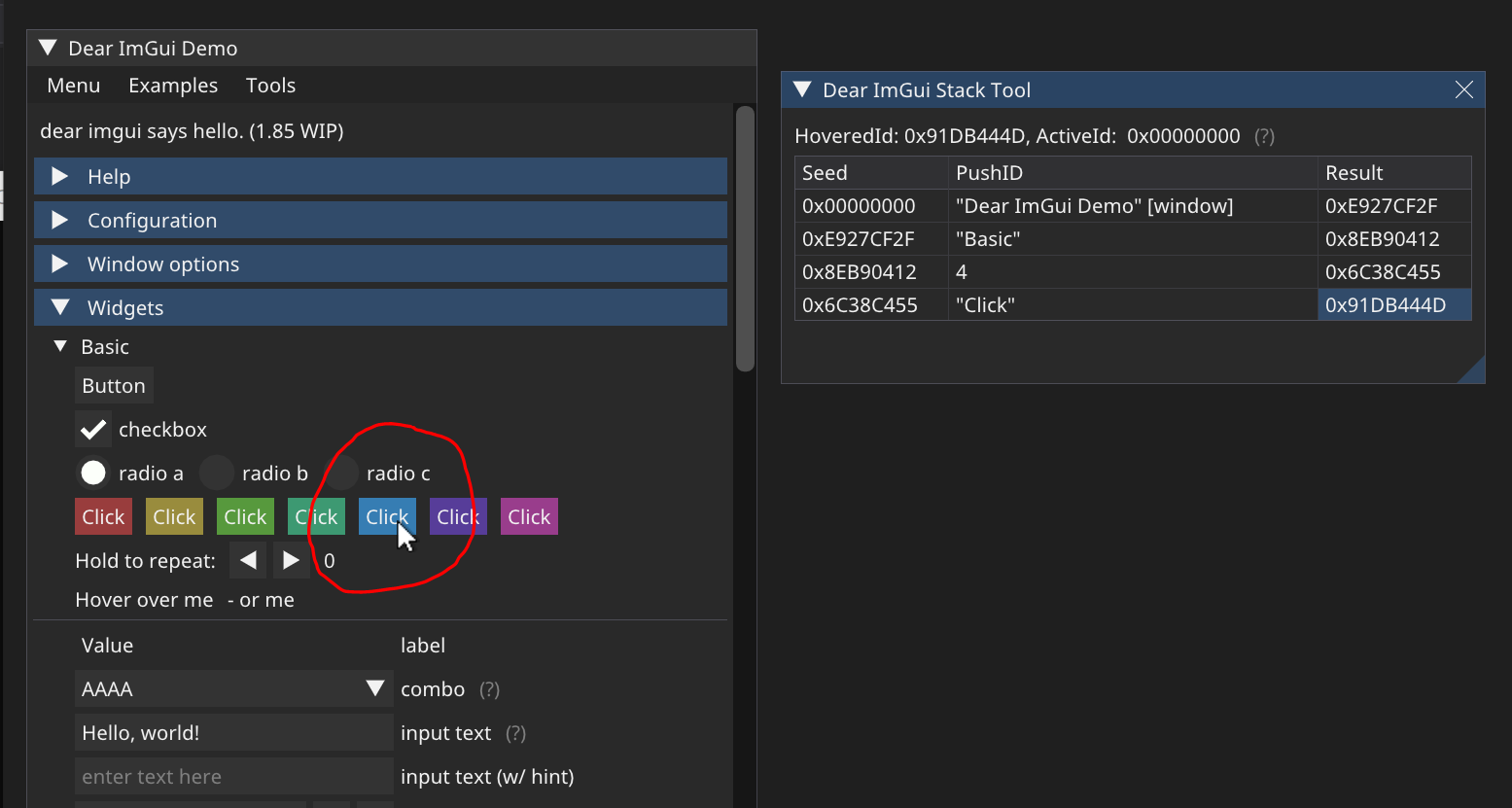
|
||||
|
||||
@ -243,9 +243,9 @@ End();
|
||||
Fear not! This is easy to solve and there are many ways to solve it!
|
||||
|
||||
- Solving ID conflict in a simple/local context:
|
||||
When passing a label you can optionally specify extra ID information within string itself.
|
||||
When passing a label you can optionally specify extra ID information within the string itself.
|
||||
Use "##" to pass a complement to the ID that won't be visible to the end-user.
|
||||
This helps solving the simple collision cases when you know e.g. at compilation time which items
|
||||
This helps solve the simple collision cases when you know e.g. at compilation time which items
|
||||
are going to be created:
|
||||
```cpp
|
||||
Begin("MyWindow");
|
||||
@ -260,7 +260,7 @@ End();
|
||||
Checkbox("##On", &b); // Label = "", ID = hash of (..., "##On") // No visible label, just a checkbox!
|
||||
```
|
||||
- Occasionally/rarely you might want to change a label while preserving a constant ID. This allows
|
||||
you to animate labels. For example you may want to include varying information in a window title bar,
|
||||
you to animate labels. For example, you may want to include varying information in a window title bar,
|
||||
but windows are uniquely identified by their ID. Use "###" to pass a label that isn't part of ID:
|
||||
```cpp
|
||||
Button("Hello###ID"); // Label = "Hello", ID = hash of (..., "###ID")
|
||||
@ -273,9 +273,9 @@ Begin(buf); // Variable title, ID = hash of "MyGame"
|
||||
Use `PushID()` / `PopID()` to create scopes and manipulate the ID stack, as to avoid ID conflicts
|
||||
within the same window. This is the most convenient way of distinguishing ID when iterating and
|
||||
creating many UI elements programmatically.
|
||||
You can push a pointer, a string or an integer value into the ID stack.
|
||||
Remember that ID are formed from the concatenation of _everything_ pushed into the ID stack.
|
||||
At each level of the stack we store the seed used for items at this level of the ID stack.
|
||||
You can push a pointer, a string, or an integer value into the ID stack.
|
||||
Remember that IDs are formed from the concatenation of _everything_ pushed into the ID stack.
|
||||
At each level of the stack, we store the seed used for items at this level of the ID stack.
|
||||
```cpp
|
||||
Begin("Window");
|
||||
for (int i = 0; i < 100; i++)
|
||||
@ -320,8 +320,8 @@ if (TreeNode("node")) // <-- this function call will do a PushID() for you (unl
|
||||
}
|
||||
```
|
||||
|
||||
When working with trees, ID are used to preserve the open/close state of each tree node.
|
||||
Depending on your use cases you may want to use strings, indices or pointers as ID.
|
||||
When working with trees, IDs are used to preserve the open/close state of each tree node.
|
||||
Depending on your use cases you may want to use strings, indices, or pointers as ID.
|
||||
- e.g. when following a single pointer that may change over time, using a static string as ID
|
||||
will preserve your node open/closed state when the targeted object change.
|
||||
- e.g. when displaying a list of objects, using indices or pointers as ID will preserve the
|
||||
@ -342,10 +342,10 @@ Short explanation:
|
||||
**Please read documentations or tutorials on your graphics API to understand how to display textures on the screen before moving onward.**
|
||||
|
||||
Long explanation:
|
||||
- Dear ImGui's job is to create "meshes", defined in a renderer-agnostic format made of draw commands and vertices. At the end of the frame those meshes (ImDrawList) will be displayed by your rendering function. They are made up of textured polygons and the code to render them is generally fairly short (a few dozen lines). In the examples/ folder we provide functions for popular graphics API (OpenGL, DirectX, etc.).
|
||||
- Dear ImGui's job is to create "meshes", defined in a renderer-agnostic format made of draw commands and vertices. At the end of the frame, those meshes (ImDrawList) will be displayed by your rendering function. They are made up of textured polygons and the code to render them is generally fairly short (a few dozen lines). In the examples/ folder, we provide functions for popular graphics APIs (OpenGL, DirectX, etc.).
|
||||
- Each rendering function decides on a data type to represent "textures". The concept of what is a "texture" is entirely tied to your underlying engine/graphics API.
|
||||
We carry the information to identify a "texture" in the ImTextureID type.
|
||||
ImTextureID is nothing more that a void*, aka 4/8 bytes worth of data: just enough to store 1 pointer or 1 integer of your choice.
|
||||
ImTextureID is nothing more than a void*, aka 4/8 bytes worth of data: just enough to store one pointer or integer of your choice.
|
||||
Dear ImGui doesn't know or understand what you are storing in ImTextureID, it merely passes ImTextureID values until they reach your rendering function.
|
||||
- In the [examples/](https://github.com/ocornut/imgui/tree/master/examples) backends, for each graphics API we decided on a type that is likely to be a good representation for specifying an image from the end-user perspective. This is what the _examples_ rendering functions are using:
|
||||
```cpp
|
||||
@ -371,9 +371,9 @@ DirectX12:
|
||||
For example, in the OpenGL example backend we store raw OpenGL texture identifier (GLuint) inside ImTextureID.
|
||||
Whereas in the DirectX11 example backend we store a pointer to ID3D11ShaderResourceView inside ImTextureID, which is a higher-level structure tying together both the texture and information about its format and how to read it.
|
||||
|
||||
- If you have a custom engine built over e.g. OpenGL, instead of passing GLuint around you may decide to use a high-level data type to carry information about the texture as well as how to display it (shaders, etc.). The decision of what to use as ImTextureID can always be made better knowing how your codebase is designed. If your engine has high-level data types for "textures" and "material" then you may want to use them.
|
||||
- If you have a custom engine built over e.g. OpenGL, instead of passing GLuint around you may decide to use a high-level data type to carry information about the texture as well as how to display it (shaders, etc.). The decision of what to use as ImTextureID can always be made better by knowing how your codebase is designed. If your engine has high-level data types for "textures" and "material" then you may want to use them.
|
||||
If you are starting with OpenGL or DirectX or Vulkan and haven't built much of a rendering engine over them, keeping the default ImTextureID representation suggested by the example backends is probably the best choice.
|
||||
(Advanced users may also decide to keep a low-level type in ImTextureID, and use ImDrawList callback and pass information to their renderer)
|
||||
(Advanced users may also decide to keep a low-level type in ImTextureID, use ImDrawList callback and pass information to their renderer)
|
||||
|
||||
User code may do:
|
||||
```cpp
|
||||
@ -387,15 +387,15 @@ The renderer function called after ImGui::Render() will receive that same value
|
||||
MyTexture* texture = (MyTexture*)pcmd->GetTexID();
|
||||
MyEngineBindTexture2D(texture);
|
||||
```
|
||||
Once you understand this design you will understand that loading image files and turning them into displayable textures is not within the scope of Dear ImGui.
|
||||
This is by design and is actually a good thing, because it means your code has full control over your data types and how you display them.
|
||||
If you want to display an image file (e.g. PNG file) into the screen, please refer to documentation and tutorials for the graphics API you are using.
|
||||
Once you understand this design, you will understand that loading image files and turning them into displayable textures is not within the scope of Dear ImGui.
|
||||
This is by design and is a good thing because it means your code has full control over your data types and how you display them.
|
||||
If you want to display an image file (e.g. PNG file) on the screen, please refer to documentation and tutorials for the graphics API you are using.
|
||||
|
||||
Refer to [Image Loading and Displaying Examples](https://github.com/ocornut/imgui/wiki/Image-Loading-and-Displaying-Examples) on the [Wiki](https://github.com/ocornut/imgui/wiki) to find simplified examples for loading textures with OpenGL, DirectX9 and DirectX11.
|
||||
|
||||
C/C++ tip: a void* is pointer-sized storage. You may safely store any pointer or integer into it by casting your value to ImTextureID / void*, and vice-versa.
|
||||
Because both end-points (user code and rendering function) are under your control, you know exactly what is stored inside the ImTextureID / void*.
|
||||
Examples:
|
||||
Here are some examples:
|
||||
```cpp
|
||||
GLuint my_tex = XXX;
|
||||
void* my_void_ptr;
|
||||
@ -423,7 +423,7 @@ This way you'll be able to use your own types everywhere, e.g. passing `MyVector
|
||||
---
|
||||
|
||||
### Q: How can I interact with standard C++ types (such as std::string and std::vector)?
|
||||
- Being highly portable (backends/bindings for several languages, frameworks, programming style, obscure or older platforms/compilers), and aiming for compatibility & performance suitable for every modern real-time game engines, dear imgui does not use any of std C++ types. We use raw types (e.g. char* instead of std::string) because they adapt to more use cases.
|
||||
- Being highly portable (backends/bindings for several languages, frameworks, programming styles, obscure or older platforms/compilers), and aiming for compatibility & performance suitable for every modern real-time game engine, Dear ImGui does not use any of std C++ types. We use raw types (e.g. char* instead of std::string) because they adapt to more use cases.
|
||||
- To use ImGui::InputText() with a std::string or any resizable string class, see [misc/cpp/imgui_stdlib.h](https://github.com/ocornut/imgui/blob/master/misc/cpp/imgui_stdlib.h).
|
||||
- To use combo boxes and list boxes with `std::vector` or any other data structure: the `BeginCombo()/EndCombo()` API
|
||||
lets you iterate and submit items yourself, so does the `ListBoxHeader()/ListBoxFooter()` API.
|
||||
@ -432,12 +432,12 @@ Prefer using them over the old and awkward `Combo()/ListBox()` api.
|
||||
You may write your own one-liner wrappers to facilitate user code (tip: add new functions in ImGui:: namespace from your code).
|
||||
- Dear ImGui applications often need to make intensive use of strings. It is expected that many of the strings you will pass
|
||||
to the API are raw literals (free in C/C++) or allocated in a manner that won't incur a large cost on your application.
|
||||
Please bear in mind that using `std::string` on applications with large amount of UI may incur unsatisfactory performances.
|
||||
Please bear in mind that using `std::string` on applications with a large amount of UI may incur unsatisfactory performances.
|
||||
Modern implementations of `std::string` often include small-string optimization (which is often a local buffer) but those
|
||||
are not configurable and not the same across implementations.
|
||||
- If you are finding your UI traversal cost to be too large, make sure your string usage is not leading to excessive amount
|
||||
of heap allocations. Consider using literals, statically sized buffers and your own helper functions. A common pattern
|
||||
is that you will need to build lots of strings on the fly, and their maximum length can be easily be scoped ahead.
|
||||
- If you are finding your UI traversal cost to be too large, make sure your string usage is not leading to an excessive amount
|
||||
of heap allocations. Consider using literals, statically sized buffers, and your own helper functions. A common pattern
|
||||
is that you will need to build lots of strings on the fly, and their maximum length can be easily scoped ahead.
|
||||
One possible implementation of a helper to facilitate printf-style building of strings: https://github.com/ocornut/Str
|
||||
This is a small helper where you can instance strings with configurable local buffers length. Many game engines will
|
||||
provide similar or better string helpers.
|
||||
@ -473,7 +473,7 @@ ImGui::End();
|
||||
- Refer to "Demo > Examples > Custom Rendering" in the demo window and read the code of `ShowExampleAppCustomRendering()` in `imgui_demo.cpp` from more examples.
|
||||
- To generate colors: you can use the macro `IM_COL32(255,255,255,255)` to generate them at compile time, or use `ImGui::GetColorU32(IM_COL32(255,255,255,255))` or `ImGui::GetColorU32(ImVec4(1.0f,1.0f,1.0f,1.0f))` to generate a color that is multiplied by the current value of `style.Alpha`.
|
||||
- Math operators: if you have setup `IM_VEC2_CLASS_EXTRA` in `imconfig.h` to bind your own math types, you can use your own math types and their natural operators instead of ImVec2. ImVec2 by default doesn't export any math operators in the public API. You may use `#define IMGUI_DEFINE_MATH_OPERATORS` `#include "imgui_internal.h"` to use the internally defined math operators, but instead prefer using your own math library and set it up in `imconfig.h`.
|
||||
- You can use `ImGui::GetBackgroundDrawList()` or `ImGui::GetForegroundDrawList()` to access draw lists which will be displayed behind and over every other dear imgui windows (one bg/fg drawlist per viewport). This is very convenient if you need to quickly display something on the screen that is not associated to a dear imgui window.
|
||||
- You can use `ImGui::GetBackgroundDrawList()` or `ImGui::GetForegroundDrawList()` to access draw lists which will be displayed behind and over every other Dear ImGui window (one bg/fg drawlist per viewport). This is very convenient if you need to quickly display something on the screen that is not associated with a Dear ImGui window.
|
||||
- You can also create your own empty window and draw inside it. Call Begin() with the NoBackground | NoDecoration | NoSavedSettings | NoInputs flags (The `ImGuiWindowFlags_NoDecoration` flag itself is a shortcut for NoTitleBar | NoResize | NoScrollbar | NoCollapse). Then you can retrieve the ImDrawList* via `GetWindowDrawList()` and draw to it in any way you like.
|
||||
- You can create your own ImDrawList instance. You'll need to initialize them with `ImGui::GetDrawListSharedData()`, or create your own instancing `ImDrawListSharedData`, and then call your renderer function with your own ImDrawList or ImDrawData data.
|
||||
- Looking for fun? The [ImDrawList coding party 2020](https://github.com/ocornut/imgui/issues/3606) thread is full of "don't do this at home" extreme uses of the ImDrawList API.
|
||||
@ -486,7 +486,7 @@ ImGui::End();
|
||||
|
||||
### Q: How should I handle DPI in my application?
|
||||
|
||||
The short answer is: obtain the desired DPI scale, load your fonts resized with that scale (always round down fonts size to nearest integer), and scale your Style structure accordingly using `style.ScaleAllSizes()`.
|
||||
The short answer is: obtain the desired DPI scale, load your fonts resized with that scale (always round down fonts size to the nearest integer), and scale your Style structure accordingly using `style.ScaleAllSizes()`.
|
||||
|
||||
Your application may want to detect DPI change and reload the fonts and reset style between frames.
|
||||
|
||||
@ -496,16 +496,16 @@ Down the line Dear ImGui will provide a variety of standardized reference values
|
||||
|
||||
Applications in the `examples/` folder are not DPI aware partly because they are unable to load a custom font from the file-system (may change that in the future).
|
||||
|
||||
The reason DPI is not auto-magically solved in stock examples is that we don't yet have a satisfying solution for the "multi-dpi" problem (using the `docking` branch: when multiple viewport windows are over multiple monitors using different DPI scale). The current way to handle this on the application side is:
|
||||
The reason DPI is not auto-magically solved in stock examples is that we don't yet have a satisfying solution for the "multi-dpi" problem (using the `docking` branch: when multiple viewport windows are over multiple monitors using different DPI scales). The current way to handle this on the application side is:
|
||||
- Create and maintain one font atlas per active DPI scale (e.g. by iterating `platform_io.Monitors[]` before `NewFrame()`).
|
||||
- Hook `platform_io.OnChangedViewport()` to detect when a `Begin()` call makes a Dear ImGui window change monitor (and therefore DPI).
|
||||
- In the hook: swap atlas, swap style with correctly sized one, remap the current font from one atlas to the other (may need to maintain a remapping table of your fonts at varying DPI scale).
|
||||
- In the hook: swap atlas, swap style with correctly sized one, and remap the current font from one atlas to the other (you may need to maintain a remapping table of your fonts at varying DPI scales).
|
||||
|
||||
This approach is relatively easy and functional but come with two issues:
|
||||
This approach is relatively easy and functional but comes with two issues:
|
||||
- It's not possibly to reliably size or position a window ahead of `Begin()` without knowing on which monitor it'll land.
|
||||
- Style override may be lost during the `Begin()` call crossing monitor boundaries. You may need to do some custom scaling mumbo-jumbo if you want your `OnChangedViewport()` handler to preserve style overrides.
|
||||
|
||||
Please note that if you are not using multi-viewports with multi-monitors using different DPI scale, you can ignore all of this and use the simpler technique recommended at the top.
|
||||
Please note that if you are not using multi-viewports with multi-monitors using different DPI scales, you can ignore that and use the simpler technique recommended at the top.
|
||||
|
||||
### Q: How can I load a different font than the default?
|
||||
Use the font atlas to load the TTF/OTF file you want:
|
||||
@ -536,11 +536,11 @@ io.Fonts->AddFontFromFileTTF("MyFolder/MyFont.ttf", size); // ALSO CORRECT
|
||||
---
|
||||
|
||||
### Q: How can I easily use icons in my application?
|
||||
The most convenient and practical way is to merge an icon font such as FontAwesome inside you
|
||||
The most convenient and practical way is to merge an icon font such as FontAwesome inside your
|
||||
main font. Then you can refer to icons within your strings.
|
||||
You may want to see `ImFontConfig::GlyphMinAdvanceX` to make your icon look monospace to facilitate alignment.
|
||||
(Read the [docs/FONTS.md](https://github.com/ocornut/imgui/blob/master/docs/FONTS.md) file for more details about icons font loading.)
|
||||
With some extra effort, you may use colorful icon by registering custom rectangle space inside the font atlas,
|
||||
With some extra effort, you may use colorful icons by registering custom rectangle space inside the font atlas,
|
||||
and copying your own graphics data into it. See docs/FONTS.md about using the AddCustomRectFontGlyph API.
|
||||
|
||||
##### [Return to Index](#index)
|
||||
@ -564,7 +564,7 @@ io.Fonts->GetTexDataAsRGBA32() or GetTexDataAsAlpha8()
|
||||
ImFontConfig config;
|
||||
config.OversampleH = 2;
|
||||
config.OversampleV = 1;
|
||||
config.GlyphOffset.y -= 1.0f; // Move everything by 1 pixels up
|
||||
config.GlyphOffset.y -= 1.0f; // Move everything by 1 pixel up
|
||||
config.GlyphExtraSpacing.x = 1.0f; // Increase spacing between characters
|
||||
io.Fonts->AddFontFromFileTTF("myfontfile.ttf", size_pixels, &config);
|
||||
|
||||
@ -598,17 +598,17 @@ builder.BuildRanges(&ranges); // Build the final result
|
||||
io.Fonts->AddFontFromFileTTF("myfontfile.ttf", 16.0f, NULL, ranges.Data);
|
||||
```
|
||||
|
||||
All your strings needs to use UTF-8 encoding. In C++11 you can encode a string literal in UTF-8
|
||||
All your strings need to use UTF-8 encoding. In C++11 you can encode a string literal in UTF-8
|
||||
by using the u8"hello" syntax. Specifying literal in your source code using a local code page
|
||||
(such as CP-923 for Japanese or CP-1251 for Cyrillic) will NOT work!
|
||||
Otherwise you can convert yourself to UTF-8 or load text data from file already saved as UTF-8.
|
||||
Otherwise, you can convert yourself to UTF-8 or load text data from a file already saved as UTF-8.
|
||||
|
||||
Text input: it is up to your application to pass the right character code by calling `io.AddInputCharacter()`.
|
||||
The applications in examples/ are doing that.
|
||||
Windows: you can use the WM_CHAR or WM_UNICHAR or WM_IME_CHAR message (depending if your app is built using Unicode or MultiByte mode).
|
||||
You may also use MultiByteToWideChar() or ToUnicode() to retrieve Unicode codepoints from MultiByte characters or keyboard state.
|
||||
You may also use `MultiByteToWideChar()` or `ToUnicode()` to retrieve Unicode codepoints from MultiByte characters or keyboard state.
|
||||
Windows: if your language is relying on an Input Method Editor (IME), you can write your HWND to ImGui::GetMainViewport()->PlatformHandleRaw
|
||||
in order for the default the default implementation of io.SetPlatformImeDataFn() to set your Microsoft IME position correctly.
|
||||
for the default implementation of io.SetPlatformImeDataFn() to set your Microsoft IME position correctly.
|
||||
|
||||
##### [Return to Index](#index)
|
||||
|
||||
@ -631,9 +631,9 @@ You may take a look at:
|
||||
|
||||
### Q: Can you create elaborate/serious tools with Dear ImGui?
|
||||
|
||||
Yes. People have written game editors, data browsers, debuggers, profilers and all sort of non-trivial tools with the library. In my experience the simplicity of the API is very empowering. Your UI runs close to your live data. Make the tools always-on and everybody in the team will be inclined to create new tools (as opposed to more "offline" UI toolkits where only a fraction of your team effectively creates tools). The list of sponsors below is also an indicator that serious game teams have been using the library.
|
||||
Yes. People have written game editors, data browsers, debuggers, profilers, and all sorts of non-trivial tools with the library. In my experience, the simplicity of the API is very empowering. Your UI runs close to your live data. Make the tools always-on and everybody in the team will be inclined to create new tools (as opposed to more "offline" UI toolkits where only a fraction of your team effectively creates tools). The list of sponsors below is also an indicator that serious game teams have been using the library.
|
||||
|
||||
Dear ImGui is very programmer centric and the immediate-mode GUI paradigm might require you to readjust some habits before you can realize its full potential. Dear ImGui is about making things that are simple, efficient and powerful.
|
||||
Dear ImGui is very programmer centric and the immediate-mode GUI paradigm might require you to readjust some habits before you can realize its full potential. Dear ImGui is about making things that are simple, efficient, and powerful.
|
||||
|
||||
Dear ImGui is built to be efficient and scalable toward the needs for AAA-quality applications running all day. The IMGUI paradigm offers different opportunities for optimization that the more typical RMGUI paradigm.
|
||||
|
||||
@ -643,9 +643,9 @@ Dear ImGui is built to be efficient and scalable toward the needs for AAA-qualit
|
||||
|
||||
### Q: Can you reskin the look of Dear ImGui?
|
||||
|
||||
Somehow. You can alter the look of the interface to some degree: changing colors, sizes, padding, rounding, fonts. However, as Dear ImGui is designed and optimized to create debug tools, the amount of skinning you can apply is limited. There is only so much you can stray away from the default look and feel of the interface. Dear ImGui is NOT designed to create user interface for games, although with ingenious use of the low-level API you can do it.
|
||||
Somehow. You can alter the look of the interface to some degree: changing colors, sizes, padding, rounding, and fonts. However, as Dear ImGui is designed and optimized to create debug tools, the amount of skinning you can apply is limited. There is only so much you can stray away from the default look and feel of the interface. Dear ImGui is NOT designed to create a user interface for games, although with ingenious use of the low-level API you can do it.
|
||||
|
||||
A reasonably skinned application may look like (screenshot from [#2529](https://github.com/ocornut/imgui/issues/2529#issuecomment-524281119))
|
||||
A reasonably skinned application may look like (screenshot from [#2529](https://github.com/ocornut/imgui/issues/2529#issuecomment-524281119)):
|
||||
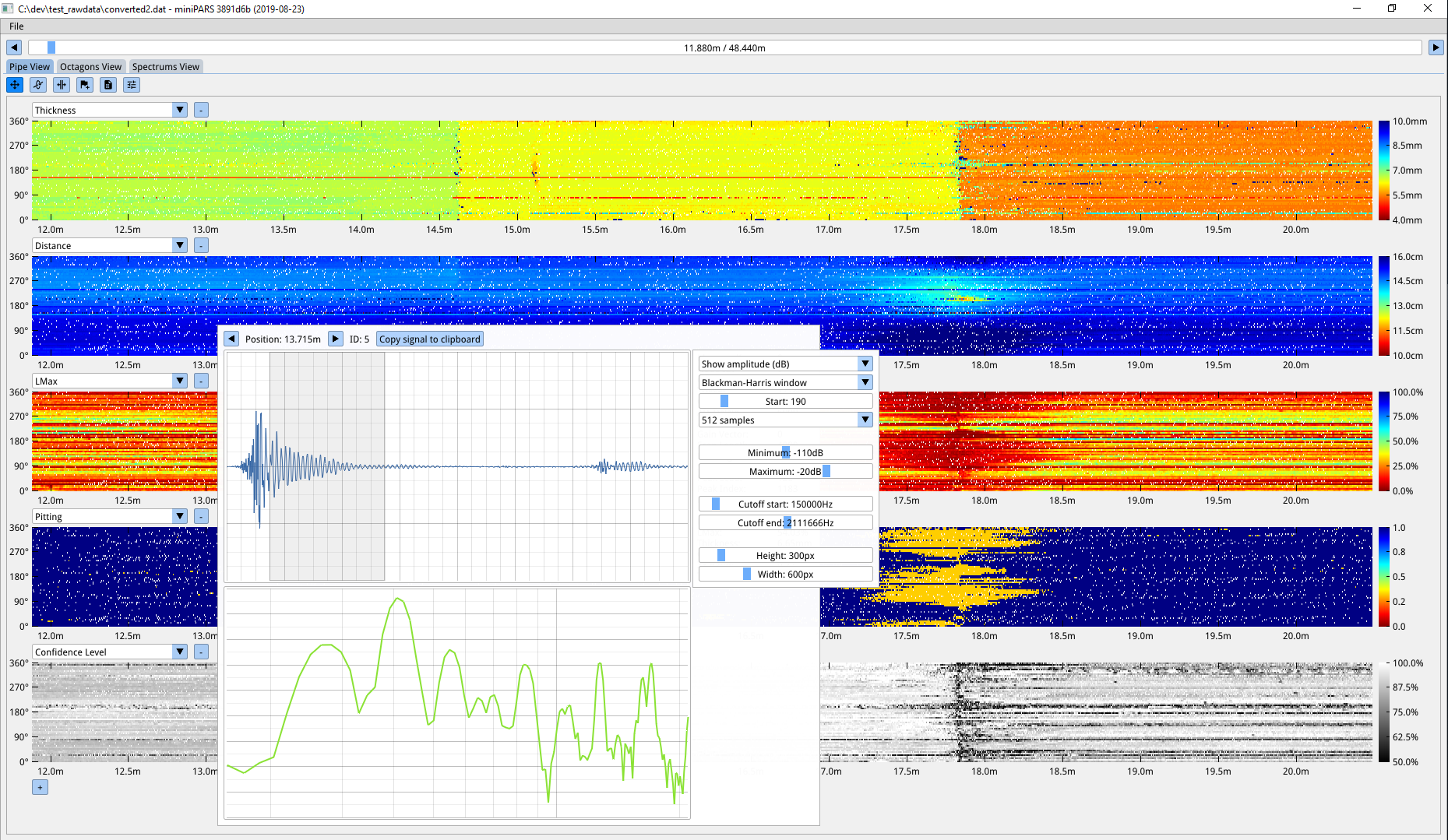
|
||||
|
||||
##### [Return to Index](#index)
|
||||
@ -654,7 +654,7 @@ A reasonably skinned application may look like (screenshot from [#2529](https://
|
||||
|
||||
### Q: Why using C++ (as opposed to C)?
|
||||
|
||||
Dear ImGui takes advantage of a few C++ languages features for convenience but nothing anywhere Boost insanity/quagmire. Dear ImGui doesn't use any C++ header file. Dear ImGui uses a very small subset of C++11 features. In particular, function overloading and default parameters are used to make the API easier to use and code more terse. Doing so I believe the API is sitting on a sweet spot and giving up on those features would make the API more cumbersome. Other features such as namespace, constructors and templates (in the case of the ImVector<> class) are also relied on as a convenience.
|
||||
Dear ImGui takes advantage of a few C++ language features for convenience but nothing anywhere Boost insanity/quagmire. Dear ImGui doesn't use any C++ header file. Dear ImGui uses a very small subset of C++11 features. In particular, function overloading and default parameters are used to make the API easier to use and code terser. Doing so I believe the API is sitting on a sweet spot and giving up on those features would make the API more cumbersome. Other features such as namespace, constructors, and templates (in the case of the ImVector<> class) are also relied on as a convenience.
|
||||
|
||||
There is an auto-generated [c-api for Dear ImGui (cimgui)](https://github.com/cimgui/cimgui) by Sonoro1234 and Stephan Dilly. It is designed for creating bindings to other languages. If possible, I would suggest using your target language functionalities to try replicating the function overloading and default parameters used in C++ else the API may be harder to use. Also see [Bindings](https://github.com/ocornut/imgui/wiki/Bindings) for various third-party bindings.
|
||||
|
||||
@ -665,11 +665,11 @@ There is an auto-generated [c-api for Dear ImGui (cimgui)](https://github.com/ci
|
||||
# Q&A: Community
|
||||
|
||||
### Q: How can I help?
|
||||
- Businesses: please reach out to `contact AT dearimgui.com` if you work in a place using Dear ImGui! We can discuss ways for your company to fund development via invoiced technical support, maintenance or sponsoring contacts. This is among the most useful thing you can do for Dear ImGui. With increased funding, we can hire more people working on this project.
|
||||
- Businesses: please reach out to `contact AT dearimgui.com` if you work in a place using Dear ImGui! We can discuss ways for your company to fund development via invoiced technical support, maintenance, or sponsoring contacts. This is among the most useful thing you can do for Dear ImGui. With increased funding, we can hire more people to work on this project.
|
||||
- Individuals: you can support continued maintenance and development via PayPal donations. See [README](https://github.com/ocornut/imgui/blob/master/docs/README.md).
|
||||
- If you are experienced with Dear ImGui and C++, look at [GitHub Issues](https://github.com/ocornut/imgui/issues), [GitHub Discussions](https://github.com/ocornut/imgui/discussions), the [Wiki](https://github.com/ocornut/imgui/wiki), read [docs/TODO.txt](https://github.com/ocornut/imgui/blob/master/docs/TODO.txt) and see how you want to help and can help!
|
||||
- Disclose your usage of Dear ImGui via a dev blog post, a tweet, a screenshot, a mention somewhere etc.
|
||||
You may post screenshot or links in the [gallery threads](https://github.com/ocornut/imgui/issues/5243). Visuals are ideal as they inspire other programmers. Disclosing your use of Dear ImGui helps the library grow credibility, and help other teams and programmers with taking decisions.
|
||||
- If you are experienced with Dear ImGui and C++, look at [GitHub Issues](https://github.com/ocornut/imgui/issues), [GitHub Discussions](https://github.com/ocornut/imgui/discussions), the [Wiki](https://github.com/ocornut/imgui/wiki), read [docs/TODO.txt](https://github.com/ocornut/imgui/blob/master/docs/TODO.txt), and see how you want to help and can help!
|
||||
- Disclose your usage of Dear ImGui via a dev blog post, a tweet, a screenshot, a mention somewhere, etc.
|
||||
You may post screenshots or links in the [gallery threads](https://github.com/ocornut/imgui/issues/5243). Visuals are ideal as they inspire other programmers. Disclosing your use of Dear ImGui helps the library grow credibility, and helps other teams and programmers with taking decisions.
|
||||
- If you have issues or if you need to hack into the library, even if you don't expect any support it is useful that you share your issues or sometimes incomplete PR.
|
||||
|
||||
##### [Return to Index](#index)
|
||||
|
@ -11,25 +11,25 @@ Dear ImGui
|
||||
|
||||
Businesses: support continued development and maintenance via invoiced technical support, maintenance, sponsoring contracts:
|
||||
<br> _E-mail: contact @ dearimgui dot com_
|
||||
|
||||
Individuals: support continued development and maintenance [here](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=WGHNC6MBFLZ2S). Also see [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors) page.
|
||||
<br>Individuals: support continued development and maintenance [here](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=WGHNC6MBFLZ2S). Also see [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors) page.
|
||||
|
||||
| [The Pitch](#the-pitch) - [Usage](#usage) - [How it works](#how-it-works) - [Releases & Changelogs](#releases--changelogs) - [Demo](#demo) - [Integration](#integration) |
|
||||
:----------------------------------------------------------: |
|
||||
| [Upcoming changes](#upcoming-changes) - [Gallery](#gallery) - [Support, FAQ](#support-frequently-asked-questions-faq) - [How to help](#how-to-help) - [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors) - [Credits](#credits) - [License](#license) |
|
||||
| [Gallery](#gallery) - [Support, FAQ](#support-frequently-asked-questions-faq) - [How to help](#how-to-help) - [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors) - [Credits](#credits) - [License](#license) |
|
||||
| [Wiki](https://github.com/ocornut/imgui/wiki) - [Languages & frameworks backends/bindings](https://github.com/ocornut/imgui/wiki/Bindings) - [Software using Dear ImGui](https://github.com/ocornut/imgui/wiki/Software-using-dear-imgui) - [User quotes](https://github.com/ocornut/imgui/wiki/Quotes) |
|
||||
|
||||
### The Pitch
|
||||
|
||||
Dear ImGui is a **bloat-free graphical user interface library for C++**. It outputs optimized vertex buffers that you can render anytime in your 3D-pipeline enabled application. It is fast, portable, renderer agnostic and self-contained (no external dependencies).
|
||||
Dear ImGui is a **bloat-free graphical user interface library for C++**. It outputs optimized vertex buffers that you can render anytime in your 3D-pipeline-enabled application. It is fast, portable, renderer agnostic, and self-contained (no external dependencies).
|
||||
|
||||
Dear ImGui is designed to **enable fast iterations** and to **empower programmers** to create **content creation tools and visualization / debug tools** (as opposed to UI for the average end-user). It favors simplicity and productivity toward this goal, and lacks certain features normally found in more high-level libraries.
|
||||
Dear ImGui is designed to **enable fast iterations** and to **empower programmers** to create **content creation tools and visualization / debug tools** (as opposed to UI for the average end-user). It favors simplicity and productivity toward this goal and lacks certain features commonly found in more high-level libraries.
|
||||
|
||||
Dear ImGui is particularly suited to integration in games engine (for tooling), real-time 3D applications, fullscreen applications, embedded applications, or any applications on consoles platforms where operating system features are non-standard.
|
||||
Dear ImGui is particularly suited to integration in game engines (for tooling), real-time 3D applications, fullscreen applications, embedded applications, or any applications on console platforms where operating system features are non-standard.
|
||||
|
||||
- Minimize state synchronization.
|
||||
- Minimize state storage on user side.
|
||||
- Minimize setup and maintenance.
|
||||
- Easy to use to create dynamic UI which are the reflection of a dynamic data set.
|
||||
- Easy to use to create code-driven and data-driven tools.
|
||||
- Easy to use to create ad hoc short-lived tools and long-lived, more elaborate tools.
|
||||
- Easy to hack and improve.
|
||||
@ -41,13 +41,11 @@ Dear ImGui is particularly suited to integration in games engine (for tooling),
|
||||
|
||||
**The core of Dear ImGui is self-contained within a few platform-agnostic files** which you can easily compile in your application/engine. They are all the files in the root folder of the repository (imgui*.cpp, imgui*.h).
|
||||
|
||||
**No specific build process is required**. You can add the .cpp files to your existing project.
|
||||
**No specific build process is required**. You can add the .cpp files into your existing project.
|
||||
|
||||
You will need a backend to integrate Dear ImGui in your app. The backend passes mouse/keyboard/gamepad inputs and variety of settings to Dear ImGui, and is in charge of rendering the resulting vertices. **Backends for a variety of graphics api and rendering platforms** are provided in the [backends/](https://github.com/ocornut/imgui/tree/master/backends) folder, along with example applications in the [examples/](https://github.com/ocornut/imgui/tree/master/examples) folder. See the [Integration](#integration) section of this document for details. You may also create your own backend. Anywhere where you can render textured triangles, you can render Dear ImGui.
|
||||
**Backends for a variety of graphics API and rendering platforms** are provided in the [backends/](https://github.com/ocornut/imgui/tree/master/backends) folder, along with example applications in the [examples/](https://github.com/ocornut/imgui/tree/master/examples) folder. See the [Integration](#integration) section of this document for details. You may also create your own backend. Anywhere where you can render textured triangles, you can render Dear ImGui.
|
||||
|
||||
After Dear ImGui is setup in your application, you can use it from \_anywhere\_ in your program loop:
|
||||
|
||||
Code:
|
||||
After Dear ImGui is set up in your application, you can use it from \_anywhere\_ in your program loop:
|
||||
```cpp
|
||||
ImGui::Text("Hello, world %d", 123);
|
||||
if (ImGui::Button("Save"))
|
||||
@ -92,37 +90,35 @@ ImGui::End();
|
||||
Result:
|
||||
<br>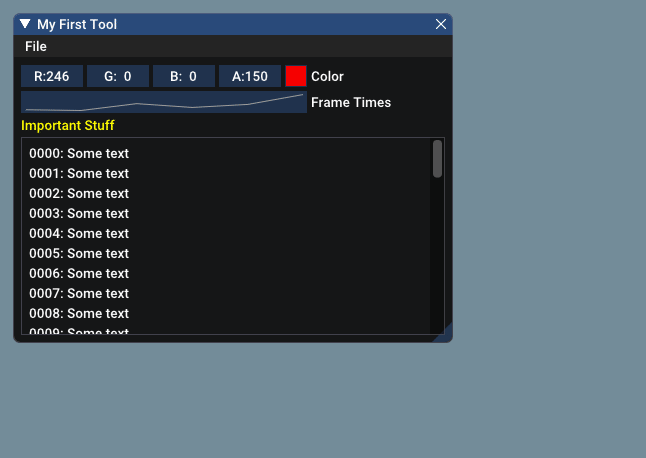
|
||||
|
||||
Dear ImGui allows you to **create elaborate tools** as well as very short-lived ones. On the extreme side of short-livedness: using the Edit&Continue (hot code reload) feature of modern compilers you can add a few widgets to tweaks variables while your application is running, and remove the code a minute later! Dear ImGui is not just for tweaking values. You can use it to trace a running algorithm by just emitting text commands. You can use it along with your own reflection data to browse your dataset live. You can use it to expose the internals of a subsystem in your engine, to create a logger, an inspection tool, a profiler, a debugger, an entire game making editor/framework, etc.
|
||||
Dear ImGui allows you to **create elaborate tools** as well as very short-lived ones. On the extreme side of short-livedness: using the Edit&Continue (hot code reload) feature of modern compilers you can add a few widgets to tweak variables while your application is running, and remove the code a minute later! Dear ImGui is not just for tweaking values. You can use it to trace a running algorithm by just emitting text commands. You can use it along with your own reflection data to browse your dataset live. You can use it to expose the internals of a subsystem in your engine, to create a logger, an inspection tool, a profiler, a debugger, an entire game-making editor/framework, etc.
|
||||
|
||||
### How it works
|
||||
|
||||
Check out the Wiki's [About the IMGUI paradigm](https://github.com/ocornut/imgui/wiki#about-the-imgui-paradigm) section if you want to understand the core principles behind the IMGUI paradigm. An IMGUI tries to minimize superfluous state duplication, state synchronization and state retention from the user's point of view. It is less error-prone (less code and less bugs) than traditional retained-mode interfaces, and lends itself to create dynamic user interfaces.
|
||||
Check out the Wiki's [About the IMGUI paradigm](https://github.com/ocornut/imgui/wiki#about-the-imgui-paradigm) section if you want to understand the core principles behind the IMGUI paradigm. An IMGUI tries to minimize superfluous state duplication, state synchronization, and state retention from the user's point of view. It is less error-prone (less code and fewer bugs) than traditional retained-mode interfaces, and lends itself to creating dynamic user interfaces.
|
||||
|
||||
Dear ImGui outputs vertex buffers and command lists that you can easily render in your application. The number of draw calls and state changes required to render them is fairly small. Because Dear ImGui doesn't know or touch graphics state directly, you can call its functions anywhere in your code (e.g. in the middle of a running algorithm, or in the middle of your own rendering process). Refer to the sample applications in the examples/ folder for instructions on how to integrate Dear ImGui with your existing codebase.
|
||||
|
||||
_A common misunderstanding is to mistake immediate mode gui for immediate mode rendering, which usually implies hammering your driver/GPU with a bunch of inefficient draw calls and state changes as the gui functions are called. This is NOT what Dear ImGui does. Dear ImGui outputs vertex buffers and a small list of draw calls batches. It never touches your GPU directly. The draw call batches are decently optimal and you can render them later, in your app or even remotely._
|
||||
_A common misunderstanding is to mistake immediate mode GUI for immediate mode rendering, which usually implies hammering your driver/GPU with a bunch of inefficient draw calls and state changes as the GUI functions are called. This is NOT what Dear ImGui does. Dear ImGui outputs vertex buffers and a small list of draw calls batches. It never touches your GPU directly. The draw call batches are decently optimal and you can render them later, in your app or even remotely._
|
||||
|
||||
### Releases & Changelogs
|
||||
|
||||
See [Releases](https://github.com/ocornut/imgui/releases) page.
|
||||
See [Releases](https://github.com/ocornut/imgui/releases) page for decorated Changelogs.
|
||||
Reading the changelogs is a good way to keep up to date with the things Dear ImGui has to offer, and maybe will give you ideas of some features that you've been ignoring until now!
|
||||
|
||||
### Demo
|
||||
|
||||
Calling the `ImGui::ShowDemoWindow()` function will create a demo window showcasing variety of features and examples. The code is always available for reference in `imgui_demo.cpp`.
|
||||
|
||||
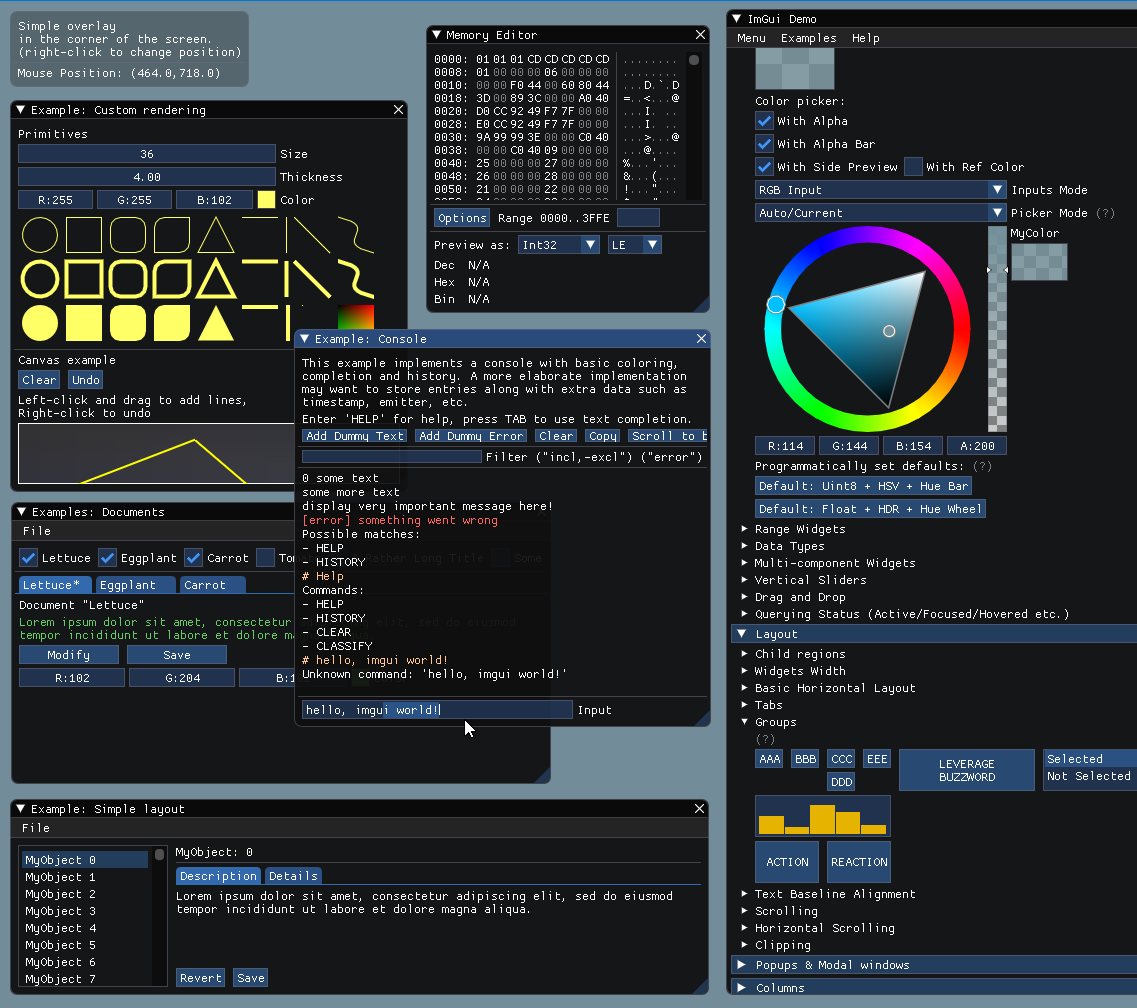
|
||||
Calling the `ImGui::ShowDemoWindow()` function will create a demo window showcasing a variety of features and examples. The code is always available for reference in `imgui_demo.cpp`. [Here's how te demo look](https://raw.githubusercontent.com/wiki/ocornut/imgui/web/v167/v167-misc.png).
|
||||
|
||||
You should be able to build the examples from sources (tested on Windows/Mac/Linux). If you don't, let us know! If you want to have a quick look at some Dear ImGui features, you can download Windows binaries of the demo app here:
|
||||
- [imgui-demo-binaries-20220504.zip](https://www.dearimgui.org/binaries/imgui-demo-binaries-20220504.zip) (Windows, 1.88 WIP, built 2022/05/04, master branch) or [older demo binaries](https://www.dearimgui.org/binaries).
|
||||
- [imgui-demo-binaries-20220504.zip](https://www.dearimgui.org/binaries/imgui-demo-binaries-20220504.zip) (Windows, 1.88 WIP, built 2022/05/04, master) or [older binaries](https://www.dearimgui.org/binaries).
|
||||
|
||||
The demo applications are not DPI aware so expect some blurriness on a 4K screen. For DPI awareness in your application, you can load/reload your font at different scale, and scale your style with `style.ScaleAllSizes()` (see [FAQ](https://www.dearimgui.org/faq)).
|
||||
The demo applications are not DPI aware so expect some blurriness on a 4K screen. For DPI awareness in your application, you can load/reload your font at a different scale and scale your style with `style.ScaleAllSizes()` (see [FAQ](https://www.dearimgui.org/faq)).
|
||||
|
||||
### Integration
|
||||
|
||||
On most platforms and when using C++, **you should be able to use a combination of the [imgui_impl_xxxx](https://github.com/ocornut/imgui/tree/master/backends) backends without modification** (e.g. `imgui_impl_win32.cpp` + `imgui_impl_dx11.cpp`). If your engine supports multiple platforms, consider using more of the imgui_impl_xxxx files instead of rewriting them: this will be less work for you and you can get Dear ImGui running immediately. You can _later_ decide to rewrite a custom backend using your custom engine functions if you wish so.
|
||||
On most platforms and when using C++, **you should be able to use a combination of the [imgui_impl_xxxx](https://github.com/ocornut/imgui/tree/master/backends) backends without modification** (e.g. `imgui_impl_win32.cpp` + `imgui_impl_dx11.cpp`). If your engine supports multiple platforms, consider using more imgui_impl_xxxx files instead of rewriting them: this will be less work for you, and you can get Dear ImGui running immediately. You can _later_ decide to rewrite a custom backend using your custom engine functions if you wish so.
|
||||
|
||||
Integrating Dear ImGui within your custom engine is a matter of 1) wiring mouse/keyboard/gamepad inputs 2) uploading one texture to your GPU/render engine 3) providing a render function that can bind textures and render textured triangles. The [examples/](https://github.com/ocornut/imgui/tree/master/examples) folder is populated with applications doing just that. If you are an experienced programmer at ease with those concepts, it should take you less than two hours to integrate Dear ImGui in your custom engine. **Make sure to spend time reading the [FAQ](https://www.dearimgui.org/faq), comments, and some of the examples/ application!**
|
||||
Integrating Dear ImGui within your custom engine is a matter of 1) wiring mouse/keyboard/gamepad inputs 2) uploading one texture to your GPU/render engine 3) providing a render function that can bind textures and render textured triangles. The [examples/](https://github.com/ocornut/imgui/tree/master/examples) folder is populated with applications doing just that. If you are an experienced programmer at ease with those concepts, it should take you less than two hours to integrate Dear ImGui into your custom engine. **Make sure to spend time reading the [FAQ](https://www.dearimgui.org/faq), comments, and the examples applications!**
|
||||
|
||||
Officially maintained backends/bindings (in repository):
|
||||
- Renderers: DirectX9, DirectX10, DirectX11, DirectX12, Metal, OpenGL/ES/ES2, SDL_Renderer, Vulkan, WebGPU.
|
||||
@ -135,36 +131,20 @@ Officially maintained backends/bindings (in repository):
|
||||
- Note that C bindings ([cimgui](https://github.com/cimgui/cimgui)) are auto-generated, you can use its json/lua output to generate bindings for other languages.
|
||||
|
||||
[Useful Extensions/Widgets](https://github.com/ocornut/imgui/wiki/Useful-Extensions) wiki page:
|
||||
- Text editors, node editors, timeline editors, plotting, software renderers, remote network access, memory editors, gizmos etc.
|
||||
- Text editors, node editors, timeline editors, plotting, software renderers, remote network access, memory editors, gizmos, etc.
|
||||
|
||||
Also see [Wiki](https://github.com/ocornut/imgui/wiki) for more links and ideas.
|
||||
|
||||
### Upcoming Changes
|
||||
|
||||
Some of the goals for 2022 are:
|
||||
- Work on Docking (see [#2109](https://github.com/ocornut/imgui/issues/2109), in public [docking](https://github.com/ocornut/imgui/tree/docking) branch)
|
||||
- Work on Multi-Viewport / Multiple OS windows. (see [#1542](https://github.com/ocornut/imgui/issues/1542), in public [docking](https://github.com/ocornut/imgui/tree/docking) branch looking for feedback)
|
||||
- Work on gamepad/keyboard controls. (see [#787](https://github.com/ocornut/imgui/issues/787))
|
||||
- Work on automation and testing system, both to test the library and end-user apps. (see [#435](https://github.com/ocornut/imgui/issues/435))
|
||||
- Make the examples look better, improve styles, improve font support, make the examples hi-DPI and multi-DPI aware.
|
||||
|
||||
### Gallery
|
||||
|
||||
For more user-submitted screenshots of projects using Dear ImGui, check out the [Gallery Threads](https://github.com/ocornut/imgui/issues/5243)!
|
||||
|
||||
For a list of third-party widgets and extensions, check out the [Useful Extensions/Widgets](https://github.com/ocornut/imgui/wiki/Useful-Extensions) wiki page.
|
||||
|
||||
Custom engine [ehre](https://github.com/tksuoran/erhe) (docking branch)
|
||||
[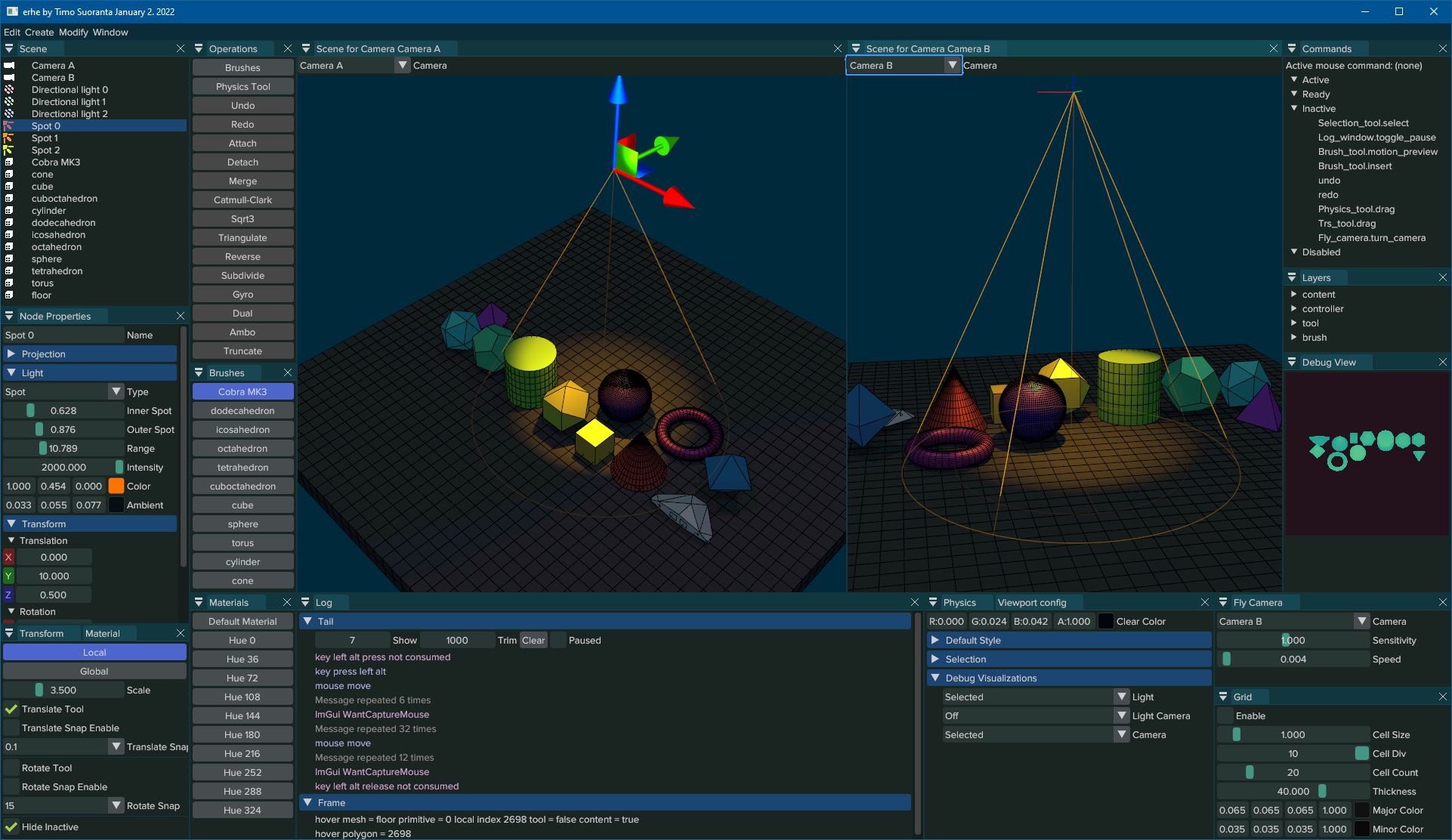](https://user-images.githubusercontent.com/994606/147875067-a848991e-2ad2-4fd3-bf71-4aeb8a547bcf.png)
|
||||
|
||||
Custom engine for [Wonder Boy: The Dragon's Trap](http://www.TheDragonsTrap.com) (2017)
|
||||
[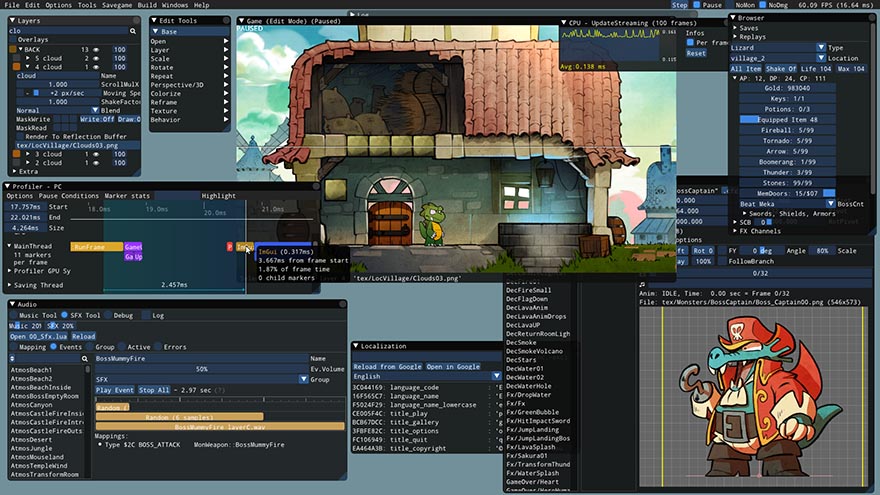](https://cloud.githubusercontent.com/assets/8225057/20628927/33e14cac-b329-11e6-80f6-9524e93b048a.png)
|
||||
|
||||
Custom engine (untitled)
|
||||
[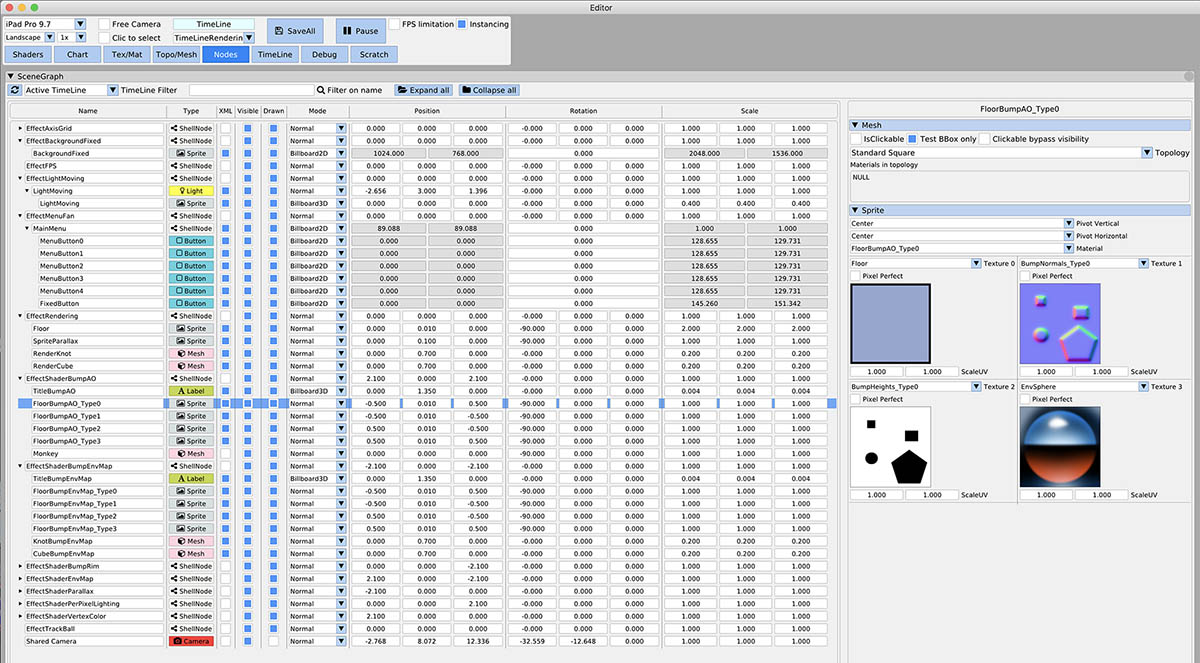](https://raw.githubusercontent.com/wiki/ocornut/imgui/web/v160/editor_white.png)
|
||||
|
||||
[Tracy Profiler](https://github.com/wolfpld/tracy)
|
||||

|
||||
| | |
|
||||
|--|--|
|
||||
| Custom engine [ehre](https://github.com/tksuoran/erhe) (docking branch)<BR>[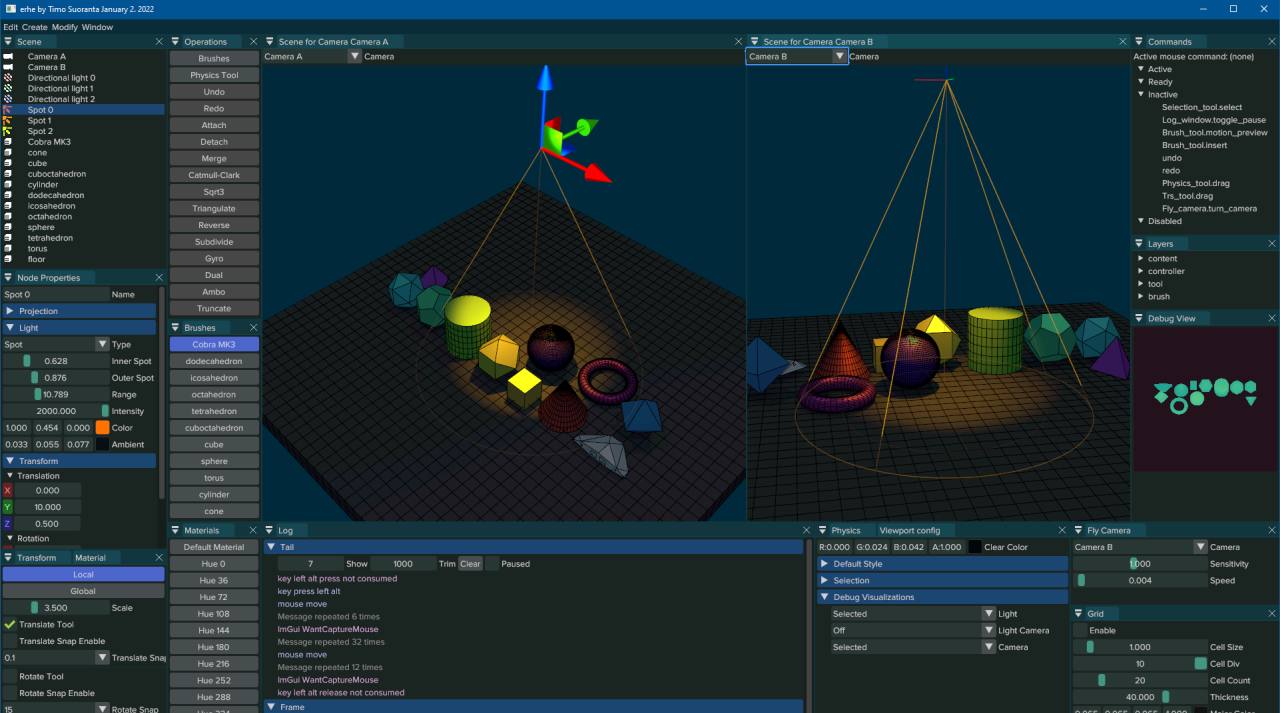](https://user-images.githubusercontent.com/994606/147875067-a848991e-2ad2-4fd3-bf71-4aeb8a547bcf.png) | Custom engine for [Wonder Boy: The Dragon's Trap](http://www.TheDragonsTrap.com) (2017)<BR>[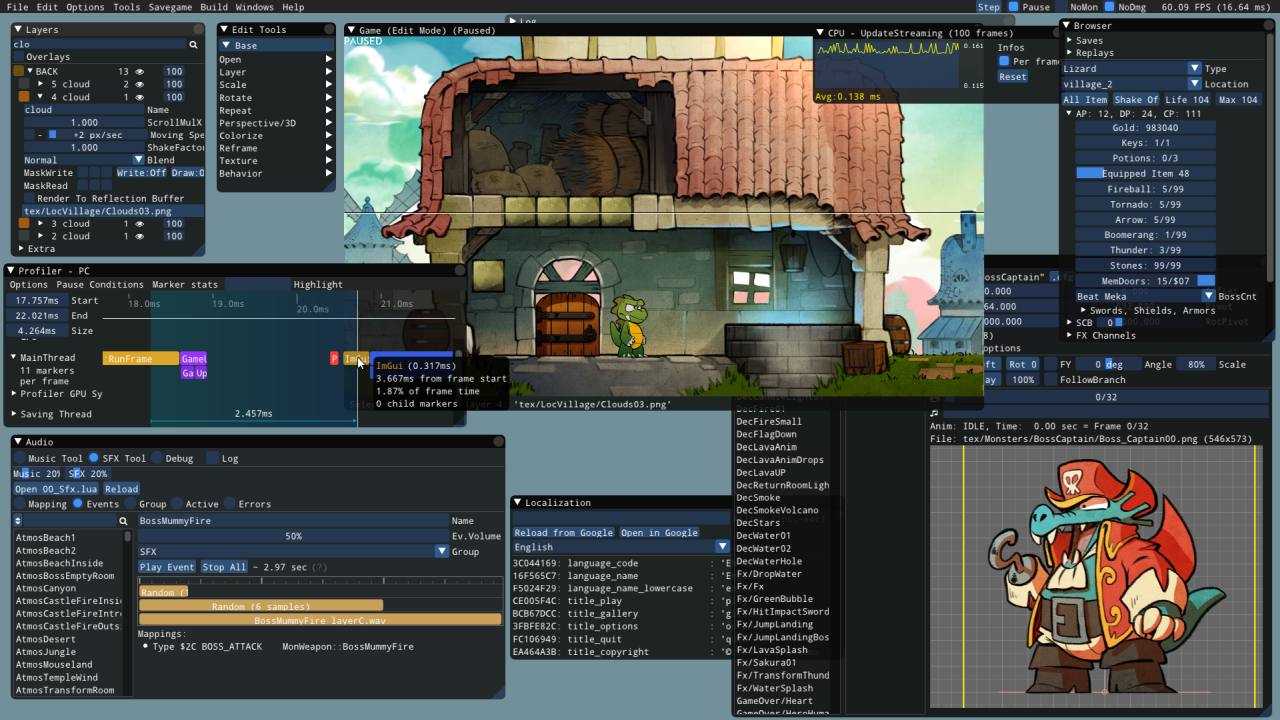](https://cloud.githubusercontent.com/assets/8225057/20628927/33e14cac-b329-11e6-80f6-9524e93b048a.png) |
|
||||
| Custom engine (untitled)<BR>[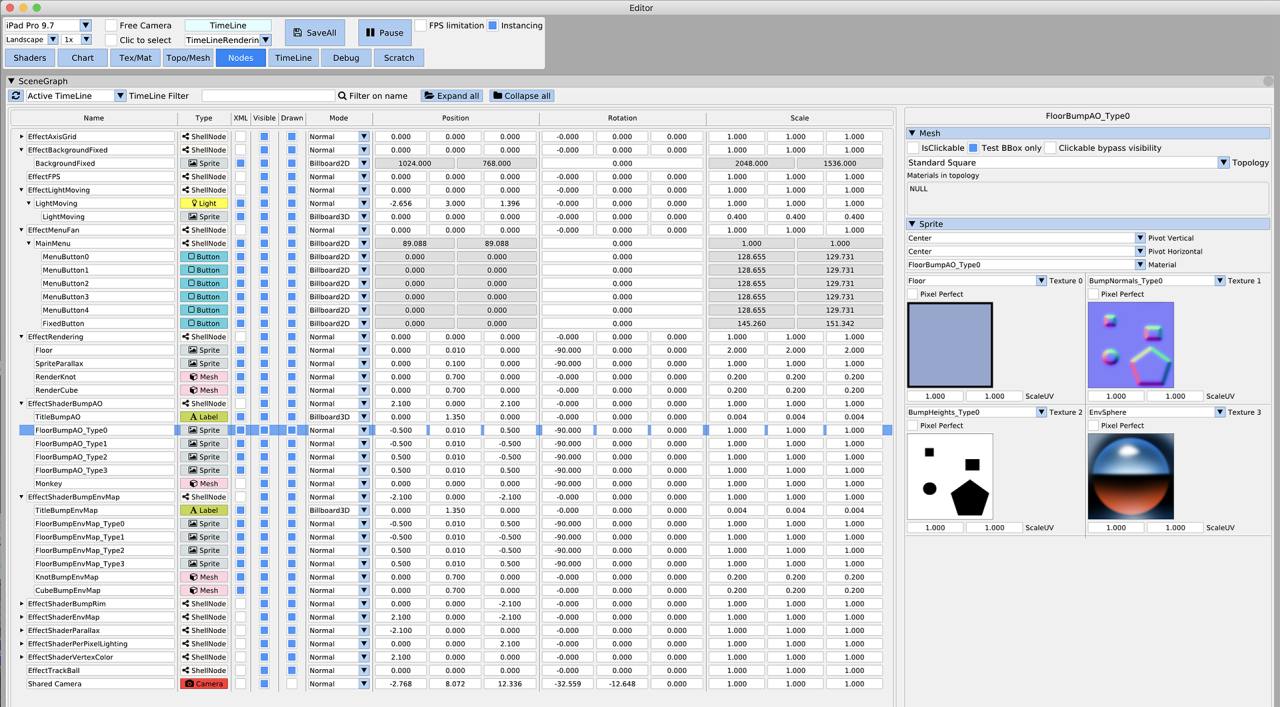](https://raw.githubusercontent.com/wiki/ocornut/imgui/web/v160/editor_white.png) | Tracy Profiler ([github](https://github.com/wolfpld/tracy))<BR>[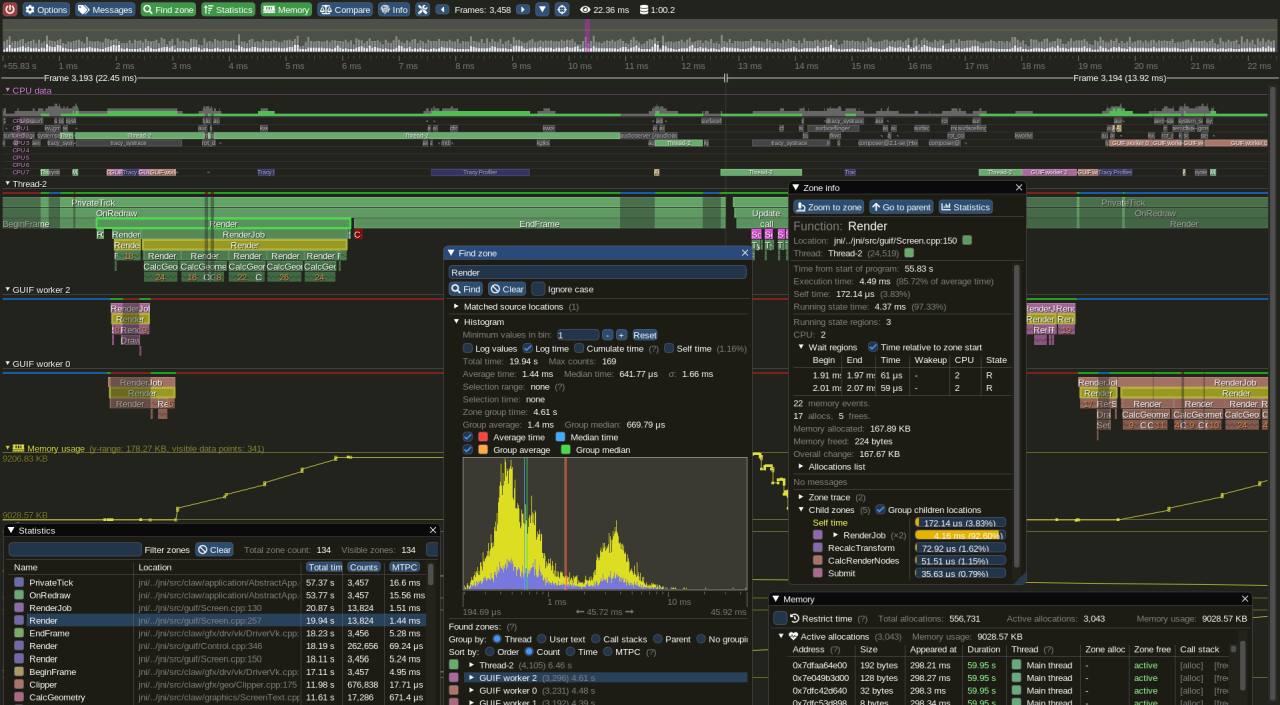](https://raw.githubusercontent.com/wiki/ocornut/imgui/web/v176/tracy_profiler.png) |
|
||||
|
||||
### Support, Frequently Asked Questions (FAQ)
|
||||
|
||||
@ -174,37 +154,35 @@ See: [Wiki](https://github.com/ocornut/imgui/wiki) for many links, references, a
|
||||
|
||||
See: [Articles about the IMGUI paradigm](https://github.com/ocornut/imgui/wiki#about-the-imgui-paradigm) to read/learn about the Immediate Mode GUI paradigm.
|
||||
|
||||
Getting started? For first-time users having issues compiling/linking/running or issues loading fonts, please use [GitHub Discussions](https://github.com/ocornut/imgui/discussions).
|
||||
See: [Upcoming Changes](https://github.com/ocornut/imgui/wiki/Upcoming-Changes).
|
||||
|
||||
For other questions, bug reports, requests, feedback, you may post on [GitHub Issues](https://github.com/ocornut/imgui/issues). Please read and fill the New Issue template carefully.
|
||||
Getting started? For first-time users having issues compiling/linking/running or issues loading fonts, please use [GitHub Discussions](https://github.com/ocornut/imgui/discussions). For other questions, bug reports, requests, feedback, you may post on [GitHub Issues](https://github.com/ocornut/imgui/issues). Please read and fill the New Issue template carefully.
|
||||
|
||||
Private support is available for paying business customers (E-mail: _contact @ dearimgui dot com_).
|
||||
|
||||
**Which version should I get?**
|
||||
|
||||
We occasionally tag [Releases](https://github.com/ocornut/imgui/releases) (with nice releases notes) but it is generally safe and recommended to sync to master/latest. The library is fairly stable and regressions tend to be fixed fast when reported.
|
||||
|
||||
Advanced users may want to use the `docking` branch with [Multi-Viewport](https://github.com/ocornut/imgui/issues/1542) and [Docking](https://github.com/ocornut/imgui/issues/2109) features. This branch is kept in sync with master regularly.
|
||||
We occasionally tag [Releases](https://github.com/ocornut/imgui/releases) (with nice releases notes) but it is generally safe and recommended to sync to master/latest. The library is fairly stable and regressions tend to be fixed fast when reported. Advanced users may want to use the `docking` branch with [Multi-Viewport](https://github.com/ocornut/imgui/issues/1542) and [Docking](https://github.com/ocornut/imgui/issues/2109) features. This branch is kept in sync with master regularly.
|
||||
|
||||
**Who uses Dear ImGui?**
|
||||
|
||||
See the [Quotes](https://github.com/ocornut/imgui/wiki/Quotes), [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors), [Software using dear imgui](https://github.com/ocornut/imgui/wiki/Software-using-dear-imgui) Wiki pages for an idea of who is using Dear ImGui. Please add your game/software if you can! Also see the [Gallery Threads](https://github.com/ocornut/imgui/issues/5243)!
|
||||
See the [Quotes](https://github.com/ocornut/imgui/wiki/Quotes), [Sponsors](https://github.com/ocornut/imgui/wiki/Sponsors), and [Software using Dear ImGui](https://github.com/ocornut/imgui/wiki/Software-using-dear-imgui) Wiki pages for an idea of who is using Dear ImGui. Please add your game/software if you can! Also, see the [Gallery Threads](https://github.com/ocornut/imgui/issues/5243)!
|
||||
|
||||
How to help
|
||||
-----------
|
||||
|
||||
**How can I help?**
|
||||
|
||||
- See [GitHub Forum/issues](https://github.com/ocornut/imgui/issues) and [Github Discussions](https://github.com/ocornut/imgui/discussions).
|
||||
- You may help with development and submit pull requests! Please understand that by submitting a PR you are also submitting a request for the maintainer to review your code and then take over its maintenance forever. PR should be crafted both in the interest in the end-users and also to ease the maintainer into understanding and accepting it.
|
||||
- See [GitHub Forum/Issues](https://github.com/ocornut/imgui/issues) and [GitHub Discussions](https://github.com/ocornut/imgui/discussions).
|
||||
- You may help with development and submit pull requests! Please understand that by submitting a PR you are also submitting a request for the maintainer to review your code and then take over its maintenance forever. PR should be crafted both in the interest of the end-users and also to ease the maintainer into understanding and accepting it.
|
||||
- See [Help wanted](https://github.com/ocornut/imgui/wiki/Help-Wanted) on the [Wiki](https://github.com/ocornut/imgui/wiki/) for some more ideas.
|
||||
- Have your company financially support this project (please reach by e-mail to say hi!).
|
||||
- Have your company financially support this project (please reach out by e-mail to say hi!).
|
||||
|
||||
Sponsors
|
||||
--------
|
||||
|
||||
Ongoing Dear ImGui development is and has been financially supported by users and private sponsors.
|
||||
<BR>Please see **[detailed list of current and past Dear ImGui supporters](https://github.com/ocornut/imgui/wiki/Sponsors)** for details.
|
||||
<BR>Please see the **[detailed list of current and past Dear ImGui supporters](https://github.com/ocornut/imgui/wiki/Sponsors)** for details.
|
||||
<BR>From November 2014 to December 2019, ongoing development has also been financially supported by its users on Patreon and through individual donations.
|
||||
|
||||
**THANK YOU to all past and present supporters for helping to keep this project alive and thriving!**
|
||||
@ -226,8 +204,7 @@ Sponsoring, support contracts and other B2B transactions are hosted and handled
|
||||
Omar: "I first discovered the IMGUI paradigm at [Q-Games](https://www.q-games.com) where Atman Binstock had dropped his own simple implementation in the codebase, which I spent quite some time improving and thinking about. It turned out that Atman was exposed to the concept directly by working with Casey. When I moved to Media Molecule I rewrote a new library trying to overcome the flaws and limitations of the first one I've worked with. It became this library and since then I have spent an unreasonable amount of time iterating and improving it."
|
||||
|
||||
Embeds [ProggyClean.ttf](http://upperbounds.net) font by Tristan Grimmer (MIT license).
|
||||
|
||||
Embeds [stb_textedit.h, stb_truetype.h, stb_rect_pack.h](https://github.com/nothings/stb/) by Sean Barrett (public domain).
|
||||
<br>Embeds [stb_textedit.h, stb_truetype.h, stb_rect_pack.h](https://github.com/nothings/stb/) by Sean Barrett (public domain).
|
||||
|
||||
Inspiration, feedback, and testing for early versions: Casey Muratori, Atman Binstock, Mikko Mononen, Emmanuel Briney, Stefan Kamoda, Anton Mikhailov, Matt Willis. Also thank you to everyone posting feedback, questions and patches on GitHub.
|
||||
|
||||
|
@ -95,7 +95,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -152,7 +152,7 @@ void tick()
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -153,7 +153,7 @@
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -95,7 +95,7 @@
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -131,7 +131,7 @@ static void main_loop(void* arg)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -166,7 +166,7 @@ static void main_loop(void* window)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -125,7 +125,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -106,7 +106,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -58,7 +58,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -127,7 +127,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -507,7 +507,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -38,7 +38,7 @@ void my_display_code()
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -54,7 +54,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -28,7 +28,7 @@ int main(int, char**)
|
||||
{
|
||||
// Setup SDL
|
||||
// (Some versions of SDL before <2.0.10 appears to have performance/stalling issues on a minority of Windows systems,
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to latest version of SDL is recommended!)
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to the latest version of SDL is recommended!)
|
||||
if (SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER | SDL_INIT_GAMECONTROLLER) != 0)
|
||||
{
|
||||
printf("Error: %s\n", SDL_GetError());
|
||||
@ -132,7 +132,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -133,7 +133,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -19,7 +19,7 @@ int main(int, char**)
|
||||
{
|
||||
// Setup SDL
|
||||
// (Some versions of SDL before <2.0.10 appears to have performance/stalling issues on a minority of Windows systems,
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to latest version of SDL is recommended!)
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to the latest version of SDL is recommended!)
|
||||
if (SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER | SDL_INIT_GAMECONTROLLER) != 0)
|
||||
{
|
||||
printf("Error: %s\n", SDL_GetError());
|
||||
@ -113,7 +113,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -58,7 +58,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -19,7 +19,7 @@ int main(int, char**)
|
||||
{
|
||||
// Setup SDL
|
||||
// (Some versions of SDL before <2.0.10 appears to have performance/stalling issues on a minority of Windows systems,
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to latest version of SDL is recommended!)
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to the latest version of SDL is recommended!)
|
||||
if (SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER | SDL_INIT_GAMECONTROLLER) != 0)
|
||||
{
|
||||
printf("Error: %s\n", SDL_GetError());
|
||||
@ -135,7 +135,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -19,7 +19,7 @@
|
||||
</ProjectConfiguration>
|
||||
</ItemGroup>
|
||||
<PropertyGroup Label="Globals">
|
||||
<ProjectGuid>{2AE17FDE-F7F3-4CAC-ADAB-0710EDA4F741}</ProjectGuid>
|
||||
<ProjectGuid>{0C0B2BEA-311F-473C-9652-87923EF639E3}</ProjectGuid>
|
||||
<RootNamespace>example_sdl_sdlrenderer</RootNamespace>
|
||||
<WindowsTargetPlatformVersion>8.1</WindowsTargetPlatformVersion>
|
||||
</PropertyGroup>
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -4,7 +4,7 @@
|
||||
// Read online: https://github.com/ocornut/imgui/tree/master/docs
|
||||
|
||||
// Important to understand: SDL_Renderer is an _optional_ component of SDL. We do not recommend you use SDL_Renderer
|
||||
// because it provide a rather limited API to the end-user. We provide this backend for the sake of completeness.
|
||||
// because it provides a rather limited API to the end-user. We provide this backend for the sake of completeness.
|
||||
// For a multi-platform app consider using e.g. SDL+DirectX on Windows and SDL+OpenGL on Linux/OSX.
|
||||
|
||||
#include "imgui.h"
|
||||
@ -22,7 +22,7 @@ int main(int, char**)
|
||||
{
|
||||
// Setup SDL
|
||||
// (Some versions of SDL before <2.0.10 appears to have performance/stalling issues on a minority of Windows systems,
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to latest version of SDL is recommended!)
|
||||
// depending on whether SDL_INIT_GAMECONTROLLER is enabled or disabled.. updating to the latest version of SDL is recommended!)
|
||||
if (SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER | SDL_INIT_GAMECONTROLLER) != 0)
|
||||
{
|
||||
printf("Error: %s\n", SDL_GetError());
|
||||
@ -107,7 +107,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
@ -508,7 +508,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -54,7 +54,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -115,7 +115,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -54,7 +54,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -120,7 +120,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -166,6 +166,9 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
</ItemGroup>
|
||||
<ItemGroup>
|
||||
<Natvis Include="..\..\misc\debuggers\imgui.natvis" />
|
||||
</ItemGroup>
|
||||
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.targets" />
|
||||
<ImportGroup Label="ExtensionTargets">
|
||||
</ImportGroup>
|
||||
|
@ -54,4 +54,9 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
<ItemGroup>
|
||||
<Natvis Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>imgui</Filter>
|
||||
</Natvis>
|
||||
</ItemGroup>
|
||||
</Project>
|
@ -153,7 +153,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -55,7 +55,7 @@
|
||||
<ItemGroup>
|
||||
<None Include="..\README.txt" />
|
||||
<None Include="..\..\misc\debuggers\imgui.natvis">
|
||||
<Filter>sources</Filter>
|
||||
<Filter>imgui</Filter>
|
||||
</None>
|
||||
</ItemGroup>
|
||||
</Project>
|
||||
</Project>
|
@ -113,7 +113,7 @@ int main(int, char**)
|
||||
if (show_demo_window)
|
||||
ImGui::ShowDemoWindow(&show_demo_window);
|
||||
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to created a named window.
|
||||
// 2. Show a simple window that we create ourselves. We use a Begin/End pair to create a named window.
|
||||
{
|
||||
static float f = 0.0f;
|
||||
static int counter = 0;
|
||||
|
@ -1,7 +1,7 @@
|
||||
|
||||
Microsoft Visual Studio Solution File, Format Version 12.00
|
||||
# Visual Studio 14
|
||||
VisualStudioVersion = 14.0.25420.1
|
||||
# Visual Studio Version 17
|
||||
VisualStudioVersion = 17.2.32616.157
|
||||
MinimumVisualStudioVersion = 10.0.40219.1
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_win32_directx9", "example_win32_directx9\example_win32_directx9.vcxproj", "{4165A294-21F2-44CA-9B38-E3F935ABADF5}"
|
||||
EndProject
|
||||
@ -17,13 +17,15 @@ Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_glfw_opengl3", "exa
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_glfw_vulkan", "example_glfw_vulkan\example_glfw_vulkan.vcxproj", "{57E2DF5A-6FC8-45BB-99DD-91A18C646E80}"
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_directx11", "example_sdl_directx11\example_sdl_directx11.vcxproj", "{9E1987E3-1F19-45CA-B9C9-D31E791836D8}"
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_opengl2", "example_sdl_opengl2\example_sdl_opengl2.vcxproj", "{2AE17FDE-F7F3-4CAC-ADAB-0710EDA4F741}"
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_opengl3", "example_sdl_opengl3\example_sdl_opengl3.vcxproj", "{BBAEB705-1669-40F3-8567-04CF6A991F4C}"
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_vulkan", "example_sdl_vulkan\example_sdl_vulkan.vcxproj", "{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}"
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_sdlrenderer", "example_sdl_sdlrenderer\example_sdl_sdlrenderer.vcxproj", "{0C0B2BEA-311F-473C-9652-87923EF639E3}"
|
||||
EndProject
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_directx11", "example_sdl_directx11\example_sdl_directx11.vcxproj", "{9E1987E3-1F19-45CA-B9C9-D31E791836D8}"
|
||||
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "example_sdl_vulkan", "example_sdl_vulkan\example_sdl_vulkan.vcxproj", "{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}"
|
||||
EndProject
|
||||
Global
|
||||
GlobalSection(SolutionConfigurationPlatforms) = preSolution
|
||||
@ -89,6 +91,14 @@ Global
|
||||
{57E2DF5A-6FC8-45BB-99DD-91A18C646E80}.Release|Win32.Build.0 = Release|Win32
|
||||
{57E2DF5A-6FC8-45BB-99DD-91A18C646E80}.Release|x64.ActiveCfg = Release|x64
|
||||
{57E2DF5A-6FC8-45BB-99DD-91A18C646E80}.Release|x64.Build.0 = Release|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|Win32.ActiveCfg = Debug|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|Win32.Build.0 = Debug|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|x64.ActiveCfg = Debug|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|x64.Build.0 = Debug|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|Win32.ActiveCfg = Release|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|Win32.Build.0 = Release|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|x64.ActiveCfg = Release|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|x64.Build.0 = Release|x64
|
||||
{2AE17FDE-F7F3-4CAC-ADAB-0710EDA4F741}.Debug|Win32.ActiveCfg = Debug|Win32
|
||||
{2AE17FDE-F7F3-4CAC-ADAB-0710EDA4F741}.Debug|Win32.Build.0 = Debug|Win32
|
||||
{2AE17FDE-F7F3-4CAC-ADAB-0710EDA4F741}.Debug|x64.ActiveCfg = Debug|x64
|
||||
@ -105,6 +115,14 @@ Global
|
||||
{BBAEB705-1669-40F3-8567-04CF6A991F4C}.Release|Win32.Build.0 = Release|Win32
|
||||
{BBAEB705-1669-40F3-8567-04CF6A991F4C}.Release|x64.ActiveCfg = Release|x64
|
||||
{BBAEB705-1669-40F3-8567-04CF6A991F4C}.Release|x64.Build.0 = Release|x64
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Debug|Win32.ActiveCfg = Debug|Win32
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Debug|Win32.Build.0 = Debug|Win32
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Debug|x64.ActiveCfg = Debug|x64
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Debug|x64.Build.0 = Debug|x64
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Release|Win32.ActiveCfg = Release|Win32
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Release|Win32.Build.0 = Release|Win32
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Release|x64.ActiveCfg = Release|x64
|
||||
{0C0B2BEA-311F-473C-9652-87923EF639E3}.Release|x64.Build.0 = Release|x64
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Debug|Win32.ActiveCfg = Debug|Win32
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Debug|Win32.Build.0 = Debug|Win32
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Debug|x64.ActiveCfg = Debug|x64
|
||||
@ -113,16 +131,11 @@ Global
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Release|Win32.Build.0 = Release|Win32
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Release|x64.ActiveCfg = Release|x64
|
||||
{BAE3D0B5-9695-4EB1-AD0F-75890EB4A3B3}.Release|x64.Build.0 = Release|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|Win32.ActiveCfg = Debug|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|Win32.Build.0 = Debug|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|x64.ActiveCfg = Debug|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Debug|x64.Build.0 = Debug|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|Win32.ActiveCfg = Release|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|Win32.Build.0 = Release|Win32
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|x64.ActiveCfg = Release|x64
|
||||
{9E1987E3-1F19-45CA-B9C9-D31E791836D8}.Release|x64.Build.0 = Release|x64
|
||||
EndGlobalSection
|
||||
GlobalSection(SolutionProperties) = preSolution
|
||||
HideSolutionNode = FALSE
|
||||
EndGlobalSection
|
||||
GlobalSection(ExtensibilityGlobals) = postSolution
|
||||
SolutionGuid = {B1ACFD20-A0A9-4A4C-ADBA-E7608F0E2BEE}
|
||||
EndGlobalSection
|
||||
EndGlobal
|
||||
|
78
imgui.cpp
78
imgui.cpp
@ -127,7 +127,7 @@ CODE
|
||||
- Hold SHIFT or use mouse to select text.
|
||||
- CTRL+Left/Right to word jump.
|
||||
- CTRL+Shift+Left/Right to select words.
|
||||
- CTRL+A our Double-Click to select all.
|
||||
- CTRL+A or Double-Click to select all.
|
||||
- CTRL+X,CTRL+C,CTRL+V to use OS clipboard/
|
||||
- CTRL+Z,CTRL+Y to undo/redo.
|
||||
- ESCAPE to revert text to its original value.
|
||||
@ -291,7 +291,7 @@ CODE
|
||||
---------------------------------------------
|
||||
The backends in impl_impl_XXX.cpp files contain many working implementations of a rendering function.
|
||||
|
||||
void void MyImGuiRenderFunction(ImDrawData* draw_data)
|
||||
void MyImGuiRenderFunction(ImDrawData* draw_data)
|
||||
{
|
||||
// TODO: Setup render state: alpha-blending enabled, no face culling, no depth testing, scissor enabled
|
||||
// TODO: Setup texture sampling state: sample with bilinear filtering (NOT point/nearest filtering). Use 'io.Fonts->Flags |= ImFontAtlasFlags_NoBakedLines;' to allow point/nearest filtering.
|
||||
@ -392,6 +392,7 @@ CODE
|
||||
- likewise io.MousePos and GetMousePos() will use OS coordinates.
|
||||
If you query mouse positions to interact with non-imgui coordinates you will need to offset them, e.g. subtract GetWindowViewport()->Pos.
|
||||
|
||||
- 2022/09/12 (1.89) - removed the bizarre legacy default argument for 'TreePush(const void* ptr = NULL)', always pass a pointer value explicitly, NULL is ok.
|
||||
- 2022/09/05 (1.89) - commented out redirecting functions/enums names that were marked obsolete in 1.77 and 1.78 (June 2020):
|
||||
- DragScalar(), DragScalarN(), DragFloat(), DragFloat2(), DragFloat3(), DragFloat4(): For old signatures ending with (..., const char* format, float power = 1.0f) -> use (..., format ImGuiSliderFlags_Logarithmic) if power != 1.0f.
|
||||
- SliderScalar(), SliderScalarN(), SliderFloat(), SliderFloat2(), SliderFloat3(), SliderFloat4(): For old signatures ending with (..., const char* format, float power = 1.0f) -> use (..., format ImGuiSliderFlags_Logarithmic) if power != 1.0f.
|
||||
@ -799,7 +800,7 @@ CODE
|
||||
- How can I have widgets with an empty label?
|
||||
- How can I have multiple widgets with the same label?
|
||||
- How can I have multiple windows with the same label?
|
||||
Q: How can I display an image? What is ImTextureID, how does it works?
|
||||
Q: How can I display an image? What is ImTextureID, how does it work?
|
||||
Q: How can I use my own math types instead of ImVec2/ImVec4?
|
||||
Q: How can I interact with standard C++ types (such as std::string and std::vector)?
|
||||
Q: How can I display custom shapes? (using low-level ImDrawList API)
|
||||
@ -905,7 +906,7 @@ CODE
|
||||
#if defined(_MSC_VER) && _MSC_VER >= 1922 // MSVC 2019 16.2 or later
|
||||
#pragma warning (disable: 5054) // operator '|': deprecated between enumerations of different types
|
||||
#endif
|
||||
#pragma warning (disable: 26451) // [Static Analyzer] Arithmetic overflow : Using operator 'xxx' on a 4 byte value and then casting the result to a 8 byte value. Cast the value to the wider type before calling operator 'xxx' to avoid overflow(io.2).
|
||||
#pragma warning (disable: 26451) // [Static Analyzer] Arithmetic overflow : Using operator 'xxx' on a 4 byte value and then casting the result to an 8 byte value. Cast the value to the wider type before calling operator 'xxx' to avoid overflow(io.2).
|
||||
#pragma warning (disable: 26495) // [Static Analyzer] Variable 'XXX' is uninitialized. Always initialize a member variable (type.6).
|
||||
#pragma warning (disable: 26812) // [Static Analyzer] The enum type 'xxx' is unscoped. Prefer 'enum class' over 'enum' (Enum.3).
|
||||
#endif
|
||||
@ -928,7 +929,7 @@ CODE
|
||||
#pragma clang diagnostic ignored "-Wdouble-promotion" // warning: implicit conversion from 'float' to 'double' when passing argument to function // using printf() is a misery with this as C++ va_arg ellipsis changes float to double.
|
||||
#pragma clang diagnostic ignored "-Wimplicit-int-float-conversion" // warning: implicit conversion from 'xxx' to 'float' may lose precision
|
||||
#elif defined(__GNUC__)
|
||||
// We disable -Wpragmas because GCC doesn't provide an has_warning equivalent and some forks/patches may not following the warning/version association.
|
||||
// We disable -Wpragmas because GCC doesn't provide a has_warning equivalent and some forks/patches may not follow the warning/version association.
|
||||
#pragma GCC diagnostic ignored "-Wpragmas" // warning: unknown option after '#pragma GCC diagnostic' kind
|
||||
#pragma GCC diagnostic ignored "-Wunused-function" // warning: 'xxxx' defined but not used
|
||||
#pragma GCC diagnostic ignored "-Wint-to-pointer-cast" // warning: cast to pointer from integer of different size
|
||||
@ -1118,7 +1119,7 @@ ImGuiStyle::ImGuiStyle()
|
||||
LogSliderDeadzone = 4.0f; // The size in pixels of the dead-zone around zero on logarithmic sliders that cross zero.
|
||||
TabRounding = 4.0f; // Radius of upper corners of a tab. Set to 0.0f to have rectangular tabs.
|
||||
TabBorderSize = 0.0f; // Thickness of border around tabs.
|
||||
TabMinWidthForCloseButton = 0.0f; // Minimum width for close button to appears on an unselected tab when hovered. Set to 0.0f to always show when hovering, set to FLT_MAX to never show close button unless selected.
|
||||
TabMinWidthForCloseButton = 0.0f; // Minimum width for close button to appear on an unselected tab when hovered. Set to 0.0f to always show when hovering, set to FLT_MAX to never show close button unless selected.
|
||||
ColorButtonPosition = ImGuiDir_Right; // Side of the color button in the ColorEdit4 widget (left/right). Defaults to ImGuiDir_Right.
|
||||
ButtonTextAlign = ImVec2(0.5f,0.5f);// Alignment of button text when button is larger than text.
|
||||
SelectableTextAlign = ImVec2(0.0f,0.0f);// Alignment of selectable text. Defaults to (0.0f, 0.0f) (top-left aligned). It's generally important to keep this left-aligned if you want to lay multiple items on a same line.
|
||||
@ -2543,7 +2544,7 @@ static bool GetSkipItemForListClipping()
|
||||
|
||||
#ifndef IMGUI_DISABLE_OBSOLETE_FUNCTIONS
|
||||
// Legacy helper to calculate coarse clipping of large list of evenly sized items.
|
||||
// This legacy API is not ideal because it assume we will return a single contiguous rectangle.
|
||||
// This legacy API is not ideal because it assumes we will return a single contiguous rectangle.
|
||||
// Prefer using ImGuiListClipper which can returns non-contiguous ranges.
|
||||
void ImGui::CalcListClipping(int items_count, float items_height, int* out_items_display_start, int* out_items_display_end)
|
||||
{
|
||||
@ -3582,7 +3583,7 @@ void ImGui::KeepAliveID(ImGuiID id)
|
||||
void ImGui::MarkItemEdited(ImGuiID id)
|
||||
{
|
||||
// This marking is solely to be able to provide info for IsItemDeactivatedAfterEdit().
|
||||
// ActiveId might have been released by the time we call this (as in the typical press/release button behavior) but still need need to fill the data.
|
||||
// ActiveId might have been released by the time we call this (as in the typical press/release button behavior) but still need to fill the data.
|
||||
ImGuiContext& g = *GImGui;
|
||||
IM_ASSERT(g.ActiveId == id || g.ActiveId == 0 || g.DragDropActive);
|
||||
IM_UNUSED(id); // Avoid unused variable warnings when asserts are compiled out.
|
||||
@ -3602,11 +3603,17 @@ static inline bool IsWindowContentHoverable(ImGuiWindow* window, ImGuiHoveredFla
|
||||
if (focused_root_window->WasActive && focused_root_window != window->RootWindowDockTree)
|
||||
{
|
||||
// For the purpose of those flags we differentiate "standard popup" from "modal popup"
|
||||
// NB: The order of those two tests is important because Modal windows are also Popups.
|
||||
// NB: The 'else' is important because Modal windows are also Popups.
|
||||
bool want_inhibit = false;
|
||||
if (focused_root_window->Flags & ImGuiWindowFlags_Modal)
|
||||
return false;
|
||||
if ((focused_root_window->Flags & ImGuiWindowFlags_Popup) && !(flags & ImGuiHoveredFlags_AllowWhenBlockedByPopup))
|
||||
return false;
|
||||
want_inhibit = true;
|
||||
else if ((focused_root_window->Flags & ImGuiWindowFlags_Popup) && !(flags & ImGuiHoveredFlags_AllowWhenBlockedByPopup))
|
||||
want_inhibit = true;
|
||||
|
||||
// Inhibit hover unless the window is within the stack of our modal/popup
|
||||
if (want_inhibit)
|
||||
if (!ImGui::IsWindowWithinBeginStackOf(window->RootWindow, focused_root_window))
|
||||
return false;
|
||||
}
|
||||
|
||||
// Filter by viewport
|
||||
@ -4798,7 +4805,7 @@ void ImGui::NewFrame()
|
||||
|
||||
// Create implicit/fallback window - which we will only render it if the user has added something to it.
|
||||
// We don't use "Debug" to avoid colliding with user trying to create a "Debug" window with custom flags.
|
||||
// This fallback is particularly important as it avoid ImGui:: calls from crashing.
|
||||
// This fallback is particularly important as it prevents ImGui:: calls from crashing.
|
||||
g.WithinFrameScopeWithImplicitWindow = true;
|
||||
SetNextWindowSize(ImVec2(400, 400), ImGuiCond_FirstUseEver);
|
||||
Begin("Debug##Default");
|
||||
@ -5621,7 +5628,7 @@ bool ImGui::BeginChildEx(const char* name, ImGuiID id, const ImVec2& size_arg, b
|
||||
ImVec2 size = ImFloor(size_arg);
|
||||
const int auto_fit_axises = ((size.x == 0.0f) ? (1 << ImGuiAxis_X) : 0x00) | ((size.y == 0.0f) ? (1 << ImGuiAxis_Y) : 0x00);
|
||||
if (size.x <= 0.0f)
|
||||
size.x = ImMax(content_avail.x + size.x, 4.0f); // Arbitrary minimum child size (0.0f causing too much issues)
|
||||
size.x = ImMax(content_avail.x + size.x, 4.0f); // Arbitrary minimum child size (0.0f causing too many issues)
|
||||
if (size.y <= 0.0f)
|
||||
size.y = ImMax(content_avail.y + size.y, 4.0f);
|
||||
SetNextWindowSize(size);
|
||||
@ -5777,7 +5784,8 @@ static void ApplyWindowSettings(ImGuiWindow* window, ImGuiWindowSettings* settin
|
||||
static void UpdateWindowInFocusOrderList(ImGuiWindow* window, bool just_created, ImGuiWindowFlags new_flags)
|
||||
{
|
||||
ImGuiContext& g = *GImGui;
|
||||
const bool new_is_explicit_child = (new_flags & ImGuiWindowFlags_ChildWindow) != 0;
|
||||
|
||||
const bool new_is_explicit_child = (new_flags & ImGuiWindowFlags_ChildWindow) != 0 && ((new_flags & ImGuiWindowFlags_Popup) == 0 || (new_flags & ImGuiWindowFlags_ChildMenu) != 0);
|
||||
const bool child_flag_changed = new_is_explicit_child != window->IsExplicitChild;
|
||||
if ((just_created || child_flag_changed) && !new_is_explicit_child)
|
||||
{
|
||||
@ -6050,7 +6058,7 @@ ImGuiID ImGui::GetWindowResizeBorderID(ImGuiWindow* window, ImGuiDir dir)
|
||||
}
|
||||
|
||||
// Handle resize for: Resize Grips, Borders, Gamepad
|
||||
// Return true when using auto-fit (double click on resize grip)
|
||||
// Return true when using auto-fit (double-click on resize grip)
|
||||
static bool ImGui::UpdateWindowManualResize(ImGuiWindow* window, const ImVec2& size_auto_fit, int* border_held, int resize_grip_count, ImU32 resize_grip_col[4], const ImRect& visibility_rect)
|
||||
{
|
||||
ImGuiContext& g = *GImGui;
|
||||
@ -6058,7 +6066,7 @@ static bool ImGui::UpdateWindowManualResize(ImGuiWindow* window, const ImVec2& s
|
||||
|
||||
if ((flags & ImGuiWindowFlags_NoResize) || (flags & ImGuiWindowFlags_AlwaysAutoResize) || window->AutoFitFramesX > 0 || window->AutoFitFramesY > 0)
|
||||
return false;
|
||||
if (window->WasActive == false) // Early out to avoid running this code for e.g. an hidden implicit/fallback Debug window.
|
||||
if (window->WasActive == false) // Early out to avoid running this code for e.g. a hidden implicit/fallback Debug window.
|
||||
return false;
|
||||
|
||||
bool ret_auto_fit = false;
|
||||
@ -6247,7 +6255,7 @@ void ImGui::RenderWindowDecorations(ImGuiWindow* window, const ImRect& title_bar
|
||||
window->SkipItems = false;
|
||||
|
||||
// Draw window + handle manual resize
|
||||
// As we highlight the title bar when want_focus is set, multiple reappearing windows will have have their title bar highlighted on their reappearing frame.
|
||||
// As we highlight the title bar when want_focus is set, multiple reappearing windows will have their title bar highlighted on their reappearing frame.
|
||||
const float window_rounding = window->WindowRounding;
|
||||
const float window_border_size = window->WindowBorderSize;
|
||||
if (window->Collapsed)
|
||||
@ -7034,7 +7042,7 @@ bool ImGui::Begin(const char* name, bool* p_open, ImGuiWindowFlags flags)
|
||||
}
|
||||
|
||||
// UPDATE RECTANGLES (1- THOSE NOT AFFECTED BY SCROLLING)
|
||||
// Update various regions. Variables they depends on should be set above in this function.
|
||||
// Update various regions. Variables they depend on should be set above in this function.
|
||||
// We set this up after processing the resize grip so that our rectangles doesn't lag by a frame.
|
||||
|
||||
// Outer rectangle
|
||||
@ -8188,7 +8196,7 @@ void ImGui::SetItemDefaultFocus()
|
||||
g.NavInitResultRectRel = WindowRectAbsToRel(window, g.LastItemData.Rect);
|
||||
NavUpdateAnyRequestFlag();
|
||||
|
||||
// Scroll could be done in NavInitRequestApplyResult() via a opt-in flag (we however don't want regular init requests to scroll)
|
||||
// Scroll could be done in NavInitRequestApplyResult() via an opt-in flag (we however don't want regular init requests to scroll)
|
||||
if (!IsItemVisible())
|
||||
ScrollToRectEx(window, g.LastItemData.Rect, ImGuiScrollFlags_None);
|
||||
}
|
||||
@ -8443,7 +8451,7 @@ int ImGui::GetKeyPressedAmount(ImGuiKey key, float repeat_delay, float repeat_ra
|
||||
{
|
||||
ImGuiContext& g = *GImGui;
|
||||
const ImGuiKeyData* key_data = GetKeyData(key);
|
||||
if (!key_data->Down) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitate eating mechanism (until we finish work on input ownership)
|
||||
if (!key_data->Down) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitates eating mechanism (until we finish work on input ownership)
|
||||
return 0;
|
||||
const float t = key_data->DownDuration;
|
||||
return CalcTypematicRepeatAmount(t - g.IO.DeltaTime, t, repeat_delay, repeat_rate);
|
||||
@ -8477,7 +8485,7 @@ bool ImGui::IsKeyPressed(ImGuiKey key, bool repeat)
|
||||
bool ImGui::IsKeyPressedEx(ImGuiKey key, ImGuiInputFlags flags)
|
||||
{
|
||||
const ImGuiKeyData* key_data = GetKeyData(key);
|
||||
if (!key_data->Down) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitate eating mechanism (until we finish work on input ownership)
|
||||
if (!key_data->Down) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitates eating mechanism (until we finish work on input ownership)
|
||||
return false;
|
||||
const float t = key_data->DownDuration;
|
||||
if (t < 0.0f)
|
||||
@ -8515,7 +8523,7 @@ bool ImGui::IsMouseClicked(ImGuiMouseButton button, bool repeat)
|
||||
{
|
||||
ImGuiContext& g = *GImGui;
|
||||
IM_ASSERT(button >= 0 && button < IM_ARRAYSIZE(g.IO.MouseDown));
|
||||
if (!g.IO.MouseDown[button]) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitate eating mechanism (until we finish work on input ownership)
|
||||
if (!g.IO.MouseDown[button]) // In theory this should already be encoded as (DownDuration < 0.0f), but testing this facilitates eating mechanism (until we finish work on input ownership)
|
||||
return false;
|
||||
const float t = g.IO.MouseDownDuration[button];
|
||||
if (t == 0.0f)
|
||||
@ -9596,7 +9604,7 @@ void ImGui::EndGroup()
|
||||
ItemAdd(group_bb, 0, NULL, ImGuiItemFlags_NoTabStop);
|
||||
|
||||
// If the current ActiveId was declared within the boundary of our group, we copy it to LastItemId so IsItemActive(), IsItemDeactivated() etc. will be functional on the entire group.
|
||||
// It would be be neater if we replaced window.DC.LastItemId by e.g. 'bool LastItemIsActive', but would put a little more burden on individual widgets.
|
||||
// It would be neater if we replaced window.DC.LastItemId by e.g. 'bool LastItemIsActive', but would put a little more burden on individual widgets.
|
||||
// Also if you grep for LastItemId you'll notice it is only used in that context.
|
||||
// (The two tests not the same because ActiveIdIsAlive is an ID itself, in order to be able to handle ActiveId being overwritten during the frame.)
|
||||
const bool group_contains_curr_active_id = (group_data.BackupActiveIdIsAlive != g.ActiveId) && (g.ActiveIdIsAlive == g.ActiveId) && g.ActiveId;
|
||||
@ -9815,7 +9823,7 @@ void ImGui::SetScrollY(float scroll_y)
|
||||
// - local_pos = (absolution_pos - window->Pos)
|
||||
// - So local_x/local_y are 0.0f for a position at the upper-left corner of a window,
|
||||
// and generally local_x/local_y are >(padding+decoration) && <(size-padding-decoration) when in the visible area.
|
||||
// - They mostly exists because of legacy API.
|
||||
// - They mostly exist because of legacy API.
|
||||
// Following the rules above, when trying to work with scrolling code, consider that:
|
||||
// - SetScrollFromPosY(0.0f) == SetScrollY(0.0f + scroll.y) == has no effect!
|
||||
// - SetScrollFromPosY(-scroll.y) == SetScrollY(-scroll.y + scroll.y) == SetScrollY(0.0f) == reset scroll. Of course writing SetScrollY(0.0f) directly then makes more sense
|
||||
@ -10397,7 +10405,7 @@ ImVec2 ImGui::FindBestWindowPosForPopupEx(const ImVec2& ref_pos, const ImVec2& s
|
||||
const float avail_w = (dir == ImGuiDir_Left ? r_avoid.Min.x : r_outer.Max.x) - (dir == ImGuiDir_Right ? r_avoid.Max.x : r_outer.Min.x);
|
||||
const float avail_h = (dir == ImGuiDir_Up ? r_avoid.Min.y : r_outer.Max.y) - (dir == ImGuiDir_Down ? r_avoid.Max.y : r_outer.Min.y);
|
||||
|
||||
// If there not enough room on one axis, there's no point in positioning on a side on this axis (e.g. when not enough width, use a top/bottom position to maximize available width)
|
||||
// If there's not enough room on one axis, there's no point in positioning on a side on this axis (e.g. when not enough width, use a top/bottom position to maximize available width)
|
||||
if (avail_w < size.x && (dir == ImGuiDir_Left || dir == ImGuiDir_Right))
|
||||
continue;
|
||||
if (avail_h < size.y && (dir == ImGuiDir_Up || dir == ImGuiDir_Down))
|
||||
@ -10599,7 +10607,7 @@ static bool ImGui::NavScoreItem(ImGuiNavItemData* result)
|
||||
}
|
||||
|
||||
// We perform scoring on items bounding box clipped by the current clipping rectangle on the other axis (clipping on our movement axis would give us equal scores for all clipped items)
|
||||
// For example, this ensure that items in one column are not reached when moving vertically from items in another column.
|
||||
// For example, this ensures that items in one column are not reached when moving vertically from items in another column.
|
||||
NavClampRectToVisibleAreaForMoveDir(g.NavMoveClipDir, cand, window->ClipRect);
|
||||
|
||||
// Compute distance between boxes
|
||||
@ -10663,7 +10671,7 @@ static bool ImGui::NavScoreItem(ImGuiNavItemData* result)
|
||||
}
|
||||
#endif
|
||||
|
||||
// Is it in the quadrant we're interesting in moving to?
|
||||
// Is it in the quadrant we're interested in moving to?
|
||||
bool new_best = false;
|
||||
const ImGuiDir move_dir = g.NavMoveDir;
|
||||
if (quadrant == move_dir)
|
||||
@ -11292,7 +11300,7 @@ void ImGui::NavUpdateCreateMoveRequest()
|
||||
if (g.NavMoveDir != ImGuiDir_None)
|
||||
NavMoveRequestSubmit(g.NavMoveDir, g.NavMoveClipDir, g.NavMoveFlags, g.NavMoveScrollFlags);
|
||||
|
||||
// Moving with no reference triggers a init request (will be used as a fallback if the direction fails to find a match)
|
||||
// Moving with no reference triggers an init request (will be used as a fallback if the direction fails to find a match)
|
||||
if (g.NavMoveSubmitted && g.NavId == 0)
|
||||
{
|
||||
IMGUI_DEBUG_LOG_NAV("[nav] NavInitRequest: from move, window \"%s\", layer=%d\n", window ? window->Name : "<NULL>", g.NavLayer);
|
||||
@ -11302,7 +11310,7 @@ void ImGui::NavUpdateCreateMoveRequest()
|
||||
}
|
||||
|
||||
// When using gamepad, we project the reference nav bounding box into window visible area.
|
||||
// This is to allow resuming navigation inside the visible area after doing a large amount of scrolling, since with gamepad every movements are relative
|
||||
// This is to allow resuming navigation inside the visible area after doing a large amount of scrolling, since with gamepad all movements are relative
|
||||
// (can't focus a visible object like we can with the mouse).
|
||||
if (g.NavMoveSubmitted && g.NavInputSource == ImGuiInputSource_Gamepad && g.NavLayer == ImGuiNavLayer_Main && window != NULL)// && (g.NavMoveFlags & ImGuiNavMoveFlags_Forwarded))
|
||||
{
|
||||
@ -11332,7 +11340,7 @@ void ImGui::NavUpdateCreateMoveRequest()
|
||||
scoring_rect.TranslateY(scoring_rect_offset_y);
|
||||
scoring_rect.Min.x = ImMin(scoring_rect.Min.x + 1.0f, scoring_rect.Max.x);
|
||||
scoring_rect.Max.x = scoring_rect.Min.x;
|
||||
IM_ASSERT(!scoring_rect.IsInverted()); // Ensure if we have a finite, non-inverted bounding box here will allows us to remove extraneous ImFabs() calls in NavScoreItem().
|
||||
IM_ASSERT(!scoring_rect.IsInverted()); // Ensure if we have a finite, non-inverted bounding box here will allow us to remove extraneous ImFabs() calls in NavScoreItem().
|
||||
//GetForegroundDrawList()->AddRect(scoring_rect.Min, scoring_rect.Max, IM_COL32(255,200,0,255)); // [DEBUG]
|
||||
//if (!g.NavScoringNoClipRect.IsInverted()) { GetForegroundDrawList()->AddRect(g.NavScoringNoClipRect.Min, g.NavScoringNoClipRect.Max, IM_COL32(255, 200, 0, 255)); } // [DEBUG]
|
||||
}
|
||||
@ -11381,7 +11389,7 @@ void ImGui::NavMoveRequestApplyResult()
|
||||
if ((g.NavTabbingCounter == 1 || g.NavTabbingDir == 0) && g.NavTabbingResultFirst.ID)
|
||||
result = &g.NavTabbingResultFirst;
|
||||
|
||||
// In a situation when there is no results but NavId != 0, re-enable the Navigation highlight (because g.NavId is not considered as a possible result)
|
||||
// In a situation when there are no results but NavId != 0, re-enable the Navigation highlight (because g.NavId is not considered as a possible result)
|
||||
if (result == NULL)
|
||||
{
|
||||
if (g.NavMoveFlags & ImGuiNavMoveFlags_Tabbing)
|
||||
@ -12152,7 +12160,7 @@ bool ImGui::BeginDragDropTargetCustom(const ImRect& bb, ImGuiID id)
|
||||
}
|
||||
|
||||
// We don't use BeginDragDropTargetCustom() and duplicate its code because:
|
||||
// 1) we use LastItemRectHoveredRect which handles items that pushes a temporarily clip rectangle in their code. Calling BeginDragDropTargetCustom(LastItemRect) would not handle them.
|
||||
// 1) we use LastItemRectHoveredRect which handles items that push a temporarily clip rectangle in their code. Calling BeginDragDropTargetCustom(LastItemRect) would not handle them.
|
||||
// 2) and it's faster. as this code may be very frequently called, we want to early out as fast as we can.
|
||||
// Also note how the HoveredWindow test is positioned differently in both functions (in both functions we optimize for the cheapest early out case)
|
||||
bool ImGui::BeginDragDropTarget()
|
||||
@ -12216,7 +12224,7 @@ const ImGuiPayload* ImGui::AcceptDragDropPayload(const char* type, ImGuiDragDrop
|
||||
// Render default drop visuals
|
||||
// FIXME-DRAGDROP: Settle on a proper default visuals for drop target.
|
||||
payload.Preview = was_accepted_previously;
|
||||
flags |= (g.DragDropSourceFlags & ImGuiDragDropFlags_AcceptNoDrawDefaultRect); // Source can also inhibit the preview (useful for external sources that lives for 1 frame)
|
||||
flags |= (g.DragDropSourceFlags & ImGuiDragDropFlags_AcceptNoDrawDefaultRect); // Source can also inhibit the preview (useful for external sources that live for 1 frame)
|
||||
if (!(flags & ImGuiDragDropFlags_AcceptNoDrawDefaultRect) && payload.Preview)
|
||||
window->DrawList->AddRect(r.Min - ImVec2(3.5f,3.5f), r.Max + ImVec2(3.5f, 3.5f), GetColorU32(ImGuiCol_DragDropTarget), 0.0f, 0, 2.0f);
|
||||
|
||||
@ -19157,7 +19165,7 @@ void ImGui::DebugHookIdInfo(ImGuiID id, ImGuiDataType data_type, const void* dat
|
||||
ImGuiStackTool* tool = &g.DebugStackTool;
|
||||
|
||||
// Step 0: stack query
|
||||
// This assume that the ID was computed with the current ID stack, which tends to be the case for our widget.
|
||||
// This assumes that the ID was computed with the current ID stack, which tends to be the case for our widget.
|
||||
if (tool->StackLevel == -1)
|
||||
{
|
||||
tool->StackLevel++;
|
||||
|
94
imgui.h
94
imgui.h
@ -20,6 +20,14 @@
|
||||
// - For first-time users having issues compiling/linking/running or issues loading fonts:
|
||||
// please post in https://github.com/ocornut/imgui/discussions if you cannot find a solution in resources above.
|
||||
|
||||
// Library Version
|
||||
// (Integer encoded as XYYZZ for use in #if preprocessor conditionals, e.g. '#if IMGUI_VERSION_NUM > 12345')
|
||||
#define IMGUI_VERSION "1.89 WIP"
|
||||
#define IMGUI_VERSION_NUM 18821
|
||||
#define IMGUI_HAS_TABLE
|
||||
#define IMGUI_HAS_VIEWPORT // Viewport WIP branch
|
||||
#define IMGUI_HAS_DOCK // Docking WIP branch
|
||||
|
||||
/*
|
||||
|
||||
Index of this file:
|
||||
@ -62,15 +70,6 @@ Index of this file:
|
||||
#include <stddef.h> // ptrdiff_t, NULL
|
||||
#include <string.h> // memset, memmove, memcpy, strlen, strchr, strcpy, strcmp
|
||||
|
||||
// Version
|
||||
// (Integer encoded as XYYZZ for use in #if preprocessor conditionals. Work in progress versions typically starts at XYY99 then bounce up to XYY00, XYY01 etc. when release tagging happens)
|
||||
#define IMGUI_VERSION "1.89 WIP"
|
||||
#define IMGUI_VERSION_NUM 18818
|
||||
#define IMGUI_CHECKVERSION() ImGui::DebugCheckVersionAndDataLayout(IMGUI_VERSION, sizeof(ImGuiIO), sizeof(ImGuiStyle), sizeof(ImVec2), sizeof(ImVec4), sizeof(ImDrawVert), sizeof(ImDrawIdx))
|
||||
#define IMGUI_HAS_TABLE
|
||||
#define IMGUI_HAS_VIEWPORT // Viewport WIP branch
|
||||
#define IMGUI_HAS_DOCK // Docking WIP branch
|
||||
|
||||
// Define attributes of all API symbols declarations (e.g. for DLL under Windows)
|
||||
// IMGUI_API is used for core imgui functions, IMGUI_IMPL_API is used for the default backends files (imgui_impl_xxx.h)
|
||||
// Using dear imgui via a shared library is not recommended, because we don't guarantee backward nor forward ABI compatibility (also function call overhead, as dear imgui is a call-heavy API)
|
||||
@ -89,6 +88,7 @@ Index of this file:
|
||||
#define IM_ARRAYSIZE(_ARR) ((int)(sizeof(_ARR) / sizeof(*(_ARR)))) // Size of a static C-style array. Don't use on pointers!
|
||||
#define IM_UNUSED(_VAR) ((void)(_VAR)) // Used to silence "unused variable warnings". Often useful as asserts may be stripped out from final builds.
|
||||
#define IM_OFFSETOF(_TYPE,_MEMBER) offsetof(_TYPE, _MEMBER) // Offset of _MEMBER within _TYPE. Standardized as offsetof() in C++11
|
||||
#define IMGUI_CHECKVERSION() ImGui::DebugCheckVersionAndDataLayout(IMGUI_VERSION, sizeof(ImGuiIO), sizeof(ImGuiStyle), sizeof(ImVec2), sizeof(ImVec4), sizeof(ImDrawVert), sizeof(ImDrawIdx))
|
||||
|
||||
// Helper Macros - IM_FMTARGS, IM_FMTLIST: Apply printf-style warnings to our formatting functions.
|
||||
#if !defined(IMGUI_USE_STB_SPRINTF) && defined(__MINGW32__) && !defined(__clang__)
|
||||
@ -308,7 +308,7 @@ namespace ImGui
|
||||
IMGUI_API void ShowStyleEditor(ImGuiStyle* ref = NULL); // add style editor block (not a window). you can pass in a reference ImGuiStyle structure to compare to, revert to and save to (else it uses the default style)
|
||||
IMGUI_API bool ShowStyleSelector(const char* label); // add style selector block (not a window), essentially a combo listing the default styles.
|
||||
IMGUI_API void ShowFontSelector(const char* label); // add font selector block (not a window), essentially a combo listing the loaded fonts.
|
||||
IMGUI_API void ShowUserGuide(); // add basic help/info block (not a window): how to manipulate ImGui as a end-user (mouse/keyboard controls).
|
||||
IMGUI_API void ShowUserGuide(); // add basic help/info block (not a window): how to manipulate ImGui as an end-user (mouse/keyboard controls).
|
||||
IMGUI_API const char* GetVersion(); // get the compiled version string e.g. "1.80 WIP" (essentially the value for IMGUI_VERSION from the compiled version of imgui.cpp)
|
||||
|
||||
// Styles
|
||||
@ -383,7 +383,7 @@ namespace ImGui
|
||||
IMGUI_API ImVec2 GetContentRegionAvail(); // == GetContentRegionMax() - GetCursorPos()
|
||||
IMGUI_API ImVec2 GetContentRegionMax(); // current content boundaries (typically window boundaries including scrolling, or current column boundaries), in windows coordinates
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMin(); // content boundaries min for the full window (roughly (0,0)-Scroll), in window coordinates
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMax(); // content boundaries max for the full window (roughly (0,0)+Size-Scroll) where Size can be override with SetNextWindowContentSize(), in window coordinates
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMax(); // content boundaries max for the full window (roughly (0,0)+Size-Scroll) where Size can be overridden with SetNextWindowContentSize(), in window coordinates
|
||||
|
||||
// Windows Scrolling
|
||||
IMGUI_API float GetScrollX(); // get scrolling amount [0 .. GetScrollMaxX()]
|
||||
@ -438,7 +438,7 @@ namespace ImGui
|
||||
// Absolute coordinate: GetCursorScreenPos(), SetCursorScreenPos(), all ImDrawList:: functions.
|
||||
IMGUI_API void Separator(); // separator, generally horizontal. inside a menu bar or in horizontal layout mode, this becomes a vertical separator.
|
||||
IMGUI_API void SameLine(float offset_from_start_x=0.0f, float spacing=-1.0f); // call between widgets or groups to layout them horizontally. X position given in window coordinates.
|
||||
IMGUI_API void NewLine(); // undo a SameLine() or force a new line when in an horizontal-layout context.
|
||||
IMGUI_API void NewLine(); // undo a SameLine() or force a new line when in a horizontal-layout context.
|
||||
IMGUI_API void Spacing(); // add vertical spacing.
|
||||
IMGUI_API void Dummy(const ImVec2& size); // add a dummy item of given size. unlike InvisibleButton(), Dummy() won't take the mouse click or be navigable into.
|
||||
IMGUI_API void Indent(float indent_w = 0.0f); // move content position toward the right, by indent_w, or style.IndentSpacing if indent_w <= 0
|
||||
@ -526,7 +526,7 @@ namespace ImGui
|
||||
|
||||
// Widgets: Drag Sliders
|
||||
// - CTRL+Click on any drag box to turn them into an input box. Manually input values aren't clamped by default and can go off-bounds. Use ImGuiSliderFlags_AlwaysClamp to always clamp.
|
||||
// - For all the Float2/Float3/Float4/Int2/Int3/Int4 versions of every functions, note that a 'float v[X]' function argument is the same as 'float* v',
|
||||
// - For all the Float2/Float3/Float4/Int2/Int3/Int4 versions of every function, note that a 'float v[X]' function argument is the same as 'float* v',
|
||||
// the array syntax is just a way to document the number of elements that are expected to be accessible. You can pass address of your first element out of a contiguous set, e.g. &myvector.x
|
||||
// - Adjust format string to decorate the value with a prefix, a suffix, or adapt the editing and display precision e.g. "%.3f" -> 1.234; "%5.2f secs" -> 01.23 secs; "Biscuit: %.0f" -> Biscuit: 1; etc.
|
||||
// - Format string may also be set to NULL or use the default format ("%f" or "%d").
|
||||
@ -534,7 +534,7 @@ namespace ImGui
|
||||
// - Use v_min < v_max to clamp edits to given limits. Note that CTRL+Click manual input can override those limits if ImGuiSliderFlags_AlwaysClamp is not used.
|
||||
// - Use v_max = FLT_MAX / INT_MAX etc to avoid clamping to a maximum, same with v_min = -FLT_MAX / INT_MIN to avoid clamping to a minimum.
|
||||
// - We use the same sets of flags for DragXXX() and SliderXXX() functions as the features are the same and it makes it easier to swap them.
|
||||
// - Legacy: Pre-1.78 there are DragXXX() function signatures that takes a final `float power=1.0f' argument instead of the `ImGuiSliderFlags flags=0' argument.
|
||||
// - Legacy: Pre-1.78 there are DragXXX() function signatures that take a final `float power=1.0f' argument instead of the `ImGuiSliderFlags flags=0' argument.
|
||||
// If you get a warning converting a float to ImGuiSliderFlags, read https://github.com/ocornut/imgui/issues/3361
|
||||
IMGUI_API bool DragFloat(const char* label, float* v, float v_speed = 1.0f, float v_min = 0.0f, float v_max = 0.0f, const char* format = "%.3f", ImGuiSliderFlags flags = 0); // If v_min >= v_max we have no bound
|
||||
IMGUI_API bool DragFloat2(const char* label, float v[2], float v_speed = 1.0f, float v_min = 0.0f, float v_max = 0.0f, const char* format = "%.3f", ImGuiSliderFlags flags = 0);
|
||||
@ -553,7 +553,7 @@ namespace ImGui
|
||||
// - CTRL+Click on any slider to turn them into an input box. Manually input values aren't clamped by default and can go off-bounds. Use ImGuiSliderFlags_AlwaysClamp to always clamp.
|
||||
// - Adjust format string to decorate the value with a prefix, a suffix, or adapt the editing and display precision e.g. "%.3f" -> 1.234; "%5.2f secs" -> 01.23 secs; "Biscuit: %.0f" -> Biscuit: 1; etc.
|
||||
// - Format string may also be set to NULL or use the default format ("%f" or "%d").
|
||||
// - Legacy: Pre-1.78 there are SliderXXX() function signatures that takes a final `float power=1.0f' argument instead of the `ImGuiSliderFlags flags=0' argument.
|
||||
// - Legacy: Pre-1.78 there are SliderXXX() function signatures that take a final `float power=1.0f' argument instead of the `ImGuiSliderFlags flags=0' argument.
|
||||
// If you get a warning converting a float to ImGuiSliderFlags, read https://github.com/ocornut/imgui/issues/3361
|
||||
IMGUI_API bool SliderFloat(const char* label, float* v, float v_min, float v_max, const char* format = "%.3f", ImGuiSliderFlags flags = 0); // adjust format to decorate the value with a prefix or a suffix for in-slider labels or unit display.
|
||||
IMGUI_API bool SliderFloat2(const char* label, float v[2], float v_min, float v_max, const char* format = "%.3f", ImGuiSliderFlags flags = 0);
|
||||
@ -611,7 +611,7 @@ namespace ImGui
|
||||
IMGUI_API bool TreeNodeExV(const char* str_id, ImGuiTreeNodeFlags flags, const char* fmt, va_list args) IM_FMTLIST(3);
|
||||
IMGUI_API bool TreeNodeExV(const void* ptr_id, ImGuiTreeNodeFlags flags, const char* fmt, va_list args) IM_FMTLIST(3);
|
||||
IMGUI_API void TreePush(const char* str_id); // ~ Indent()+PushId(). Already called by TreeNode() when returning true, but you can call TreePush/TreePop yourself if desired.
|
||||
IMGUI_API void TreePush(const void* ptr_id = NULL); // "
|
||||
IMGUI_API void TreePush(const void* ptr_id); // "
|
||||
IMGUI_API void TreePop(); // ~ Unindent()+PopId()
|
||||
IMGUI_API float GetTreeNodeToLabelSpacing(); // horizontal distance preceding label when using TreeNode*() or Bullet() == (g.FontSize + style.FramePadding.x*2) for a regular unframed TreeNode
|
||||
IMGUI_API bool CollapsingHeader(const char* label, ImGuiTreeNodeFlags flags = 0); // if returning 'true' the header is open. doesn't indent nor push on ID stack. user doesn't have to call TreePop().
|
||||
@ -681,7 +681,7 @@ namespace ImGui
|
||||
|
||||
// Popups: begin/end functions
|
||||
// - BeginPopup(): query popup state, if open start appending into the window. Call EndPopup() afterwards. ImGuiWindowFlags are forwarded to the window.
|
||||
// - BeginPopupModal(): block every interactions behind the window, cannot be closed by user, add a dimming background, has a title bar.
|
||||
// - BeginPopupModal(): block every interaction behind the window, cannot be closed by user, add a dimming background, has a title bar.
|
||||
IMGUI_API bool BeginPopup(const char* str_id, ImGuiWindowFlags flags = 0); // return true if the popup is open, and you can start outputting to it.
|
||||
IMGUI_API bool BeginPopupModal(const char* name, bool* p_open = NULL, ImGuiWindowFlags flags = 0); // return true if the modal is open, and you can start outputting to it.
|
||||
IMGUI_API void EndPopup(); // only call EndPopup() if BeginPopupXXX() returns true!
|
||||
@ -725,7 +725,7 @@ namespace ImGui
|
||||
// - 4. Optionally call TableHeadersRow() to submit a header row. Names are pulled from TableSetupColumn() data.
|
||||
// - 5. Populate contents:
|
||||
// - In most situations you can use TableNextRow() + TableSetColumnIndex(N) to start appending into a column.
|
||||
// - If you are using tables as a sort of grid, where every columns is holding the same type of contents,
|
||||
// - If you are using tables as a sort of grid, where every column is holding the same type of contents,
|
||||
// you may prefer using TableNextColumn() instead of TableNextRow() + TableSetColumnIndex().
|
||||
// TableNextColumn() will automatically wrap-around into the next row if needed.
|
||||
// - IMPORTANT: Comparatively to the old Columns() API, we need to call TableNextColumn() for the first column!
|
||||
@ -857,12 +857,12 @@ namespace ImGui
|
||||
IMGUI_API bool IsItemHovered(ImGuiHoveredFlags flags = 0); // is the last item hovered? (and usable, aka not blocked by a popup, etc.). See ImGuiHoveredFlags for more options.
|
||||
IMGUI_API bool IsItemActive(); // is the last item active? (e.g. button being held, text field being edited. This will continuously return true while holding mouse button on an item. Items that don't interact will always return false)
|
||||
IMGUI_API bool IsItemFocused(); // is the last item focused for keyboard/gamepad navigation?
|
||||
IMGUI_API bool IsItemClicked(ImGuiMouseButton mouse_button = 0); // is the last item hovered and mouse clicked on? (**) == IsMouseClicked(mouse_button) && IsItemHovered()Important. (**) this it NOT equivalent to the behavior of e.g. Button(). Read comments in function definition.
|
||||
IMGUI_API bool IsItemClicked(ImGuiMouseButton mouse_button = 0); // is the last item hovered and mouse clicked on? (**) == IsMouseClicked(mouse_button) && IsItemHovered()Important. (**) this is NOT equivalent to the behavior of e.g. Button(). Read comments in function definition.
|
||||
IMGUI_API bool IsItemVisible(); // is the last item visible? (items may be out of sight because of clipping/scrolling)
|
||||
IMGUI_API bool IsItemEdited(); // did the last item modify its underlying value this frame? or was pressed? This is generally the same as the "bool" return value of many widgets.
|
||||
IMGUI_API bool IsItemActivated(); // was the last item just made active (item was previously inactive).
|
||||
IMGUI_API bool IsItemDeactivated(); // was the last item just made inactive (item was previously active). Useful for Undo/Redo patterns with widgets that requires continuous editing.
|
||||
IMGUI_API bool IsItemDeactivatedAfterEdit(); // was the last item just made inactive and made a value change when it was active? (e.g. Slider/Drag moved). Useful for Undo/Redo patterns with widgets that requires continuous editing. Note that you may get false positives (some widgets such as Combo()/ListBox()/Selectable() will return true even when clicking an already selected item).
|
||||
IMGUI_API bool IsItemDeactivated(); // was the last item just made inactive (item was previously active). Useful for Undo/Redo patterns with widgets that require continuous editing.
|
||||
IMGUI_API bool IsItemDeactivatedAfterEdit(); // was the last item just made inactive and made a value change when it was active? (e.g. Slider/Drag moved). Useful for Undo/Redo patterns with widgets that require continuous editing. Note that you may get false positives (some widgets such as Combo()/ListBox()/Selectable() will return true even when clicking an already selected item).
|
||||
IMGUI_API bool IsItemToggledOpen(); // was the last item open state toggled? set by TreeNode().
|
||||
IMGUI_API bool IsAnyItemHovered(); // is any item hovered?
|
||||
IMGUI_API bool IsAnyItemActive(); // is any item active?
|
||||
@ -1081,7 +1081,7 @@ enum ImGuiTreeNodeFlags_
|
||||
// It is therefore guaranteed to be legal to pass a mouse button index in ImGuiPopupFlags.
|
||||
// - For the same reason, we exceptionally default the ImGuiPopupFlags argument of BeginPopupContextXXX functions to 1 instead of 0.
|
||||
// IMPORTANT: because the default parameter is 1 (==ImGuiPopupFlags_MouseButtonRight), if you rely on the default parameter
|
||||
// and want to another another flag, you need to pass in the ImGuiPopupFlags_MouseButtonRight flag.
|
||||
// and want to use another flag, you need to pass in the ImGuiPopupFlags_MouseButtonRight flag explicitly.
|
||||
// - Multiple buttons currently cannot be combined/or-ed in those functions (we could allow it later).
|
||||
enum ImGuiPopupFlags_
|
||||
{
|
||||
@ -1102,7 +1102,7 @@ enum ImGuiPopupFlags_
|
||||
enum ImGuiSelectableFlags_
|
||||
{
|
||||
ImGuiSelectableFlags_None = 0,
|
||||
ImGuiSelectableFlags_DontClosePopups = 1 << 0, // Clicking this don't close parent popup window
|
||||
ImGuiSelectableFlags_DontClosePopups = 1 << 0, // Clicking this doesn't close parent popup window
|
||||
ImGuiSelectableFlags_SpanAllColumns = 1 << 1, // Selectable frame can span all columns (text will still fit in current column)
|
||||
ImGuiSelectableFlags_AllowDoubleClick = 1 << 2, // Generate press events on double clicks too
|
||||
ImGuiSelectableFlags_Disabled = 1 << 3, // Cannot be selected, display grayed out text
|
||||
@ -1170,7 +1170,7 @@ enum ImGuiTabItemFlags_
|
||||
// - When ScrollX is on:
|
||||
// - Table defaults to ImGuiTableFlags_SizingFixedFit -> all Columns defaults to ImGuiTableColumnFlags_WidthFixed
|
||||
// - Columns sizing policy allowed: Fixed/Auto mostly.
|
||||
// - Fixed Columns can be enlarged as needed. Table will show an horizontal scrollbar if needed.
|
||||
// - Fixed Columns can be enlarged as needed. Table will show a horizontal scrollbar if needed.
|
||||
// - When using auto-resizing (non-resizable) fixed columns, querying the content width to use item right-alignment e.g. SetNextItemWidth(-FLT_MIN) doesn't make sense, would create a feedback loop.
|
||||
// - Using Stretch columns OFTEN DOES NOT MAKE SENSE if ScrollX is on, UNLESS you have specified a value for 'inner_width' in BeginTable().
|
||||
// If you specify a value for 'inner_width' then effectively the scrolling space is known and Stretch or mixed Fixed/Stretch columns become meaningful again.
|
||||
@ -1196,8 +1196,8 @@ enum ImGuiTableFlags_
|
||||
ImGuiTableFlags_BordersInner = ImGuiTableFlags_BordersInnerV | ImGuiTableFlags_BordersInnerH, // Draw inner borders.
|
||||
ImGuiTableFlags_BordersOuter = ImGuiTableFlags_BordersOuterV | ImGuiTableFlags_BordersOuterH, // Draw outer borders.
|
||||
ImGuiTableFlags_Borders = ImGuiTableFlags_BordersInner | ImGuiTableFlags_BordersOuter, // Draw all borders.
|
||||
ImGuiTableFlags_NoBordersInBody = 1 << 11, // [ALPHA] Disable vertical borders in columns Body (borders will always appears in Headers). -> May move to style
|
||||
ImGuiTableFlags_NoBordersInBodyUntilResize = 1 << 12, // [ALPHA] Disable vertical borders in columns Body until hovered for resize (borders will always appears in Headers). -> May move to style
|
||||
ImGuiTableFlags_NoBordersInBody = 1 << 11, // [ALPHA] Disable vertical borders in columns Body (borders will always appear in Headers). -> May move to style
|
||||
ImGuiTableFlags_NoBordersInBodyUntilResize = 1 << 12, // [ALPHA] Disable vertical borders in columns Body until hovered for resize (borders will always appear in Headers). -> May move to style
|
||||
// Sizing Policy (read above for defaults)
|
||||
ImGuiTableFlags_SizingFixedFit = 1 << 13, // Columns default to _WidthFixed or _WidthAuto (if resizable or not resizable), matching contents width.
|
||||
ImGuiTableFlags_SizingFixedSame = 2 << 13, // Columns default to _WidthFixed or _WidthAuto (if resizable or not resizable), matching the maximum contents width of all columns. Implicitly enable ImGuiTableFlags_NoKeepColumnsVisible.
|
||||
@ -1211,11 +1211,11 @@ enum ImGuiTableFlags_
|
||||
// Clipping
|
||||
ImGuiTableFlags_NoClip = 1 << 20, // Disable clipping rectangle for every individual columns (reduce draw command count, items will be able to overflow into other columns). Generally incompatible with TableSetupScrollFreeze().
|
||||
// Padding
|
||||
ImGuiTableFlags_PadOuterX = 1 << 21, // Default if BordersOuterV is on. Enable outer-most padding. Generally desirable if you have headers.
|
||||
ImGuiTableFlags_NoPadOuterX = 1 << 22, // Default if BordersOuterV is off. Disable outer-most padding.
|
||||
ImGuiTableFlags_PadOuterX = 1 << 21, // Default if BordersOuterV is on. Enable outermost padding. Generally desirable if you have headers.
|
||||
ImGuiTableFlags_NoPadOuterX = 1 << 22, // Default if BordersOuterV is off. Disable outermost padding.
|
||||
ImGuiTableFlags_NoPadInnerX = 1 << 23, // Disable inner padding between columns (double inner padding if BordersOuterV is on, single inner padding if BordersOuterV is off).
|
||||
// Scrolling
|
||||
ImGuiTableFlags_ScrollX = 1 << 24, // Enable horizontal scrolling. Require 'outer_size' parameter of BeginTable() to specify the container size. Changes default sizing policy. Because this create a child window, ScrollY is currently generally recommended when using ScrollX.
|
||||
ImGuiTableFlags_ScrollX = 1 << 24, // Enable horizontal scrolling. Require 'outer_size' parameter of BeginTable() to specify the container size. Changes default sizing policy. Because this creates a child window, ScrollY is currently generally recommended when using ScrollX.
|
||||
ImGuiTableFlags_ScrollY = 1 << 25, // Enable vertical scrolling. Require 'outer_size' parameter of BeginTable() to specify the container size.
|
||||
// Sorting
|
||||
ImGuiTableFlags_SortMulti = 1 << 26, // Hold shift when clicking headers to sort on multiple column. TableGetSortSpecs() may return specs where (SpecsCount > 1).
|
||||
@ -1285,7 +1285,7 @@ enum ImGuiTableRowFlags_
|
||||
// - Layer 0: draw with RowBg0 color if set, otherwise draw with ColumnBg0 if set.
|
||||
// - Layer 1: draw with RowBg1 color if set, otherwise draw with ColumnBg1 if set.
|
||||
// - Layer 2: draw with CellBg color if set.
|
||||
// The purpose of the two row/columns layers is to let you decide if a background color changes should override or blend with the existing color.
|
||||
// The purpose of the two row/columns layers is to let you decide if a background color change should override or blend with the existing color.
|
||||
// When using ImGuiTableFlags_RowBg on the table, each row has the RowBg0 color automatically set for odd/even rows.
|
||||
// If you set the color of RowBg0 target, your color will override the existing RowBg0 color.
|
||||
// If you set the color of RowBg1 or ColumnBg1 target, your color will blend over the RowBg0 color.
|
||||
@ -1355,8 +1355,8 @@ enum ImGuiDragDropFlags_
|
||||
{
|
||||
ImGuiDragDropFlags_None = 0,
|
||||
// BeginDragDropSource() flags
|
||||
ImGuiDragDropFlags_SourceNoPreviewTooltip = 1 << 0, // By default, a successful call to BeginDragDropSource opens a tooltip so you can display a preview or description of the source contents. This flag disable this behavior.
|
||||
ImGuiDragDropFlags_SourceNoDisableHover = 1 << 1, // By default, when dragging we clear data so that IsItemHovered() will return false, to avoid subsequent user code submitting tooltips. This flag disable this behavior so you can still call IsItemHovered() on the source item.
|
||||
ImGuiDragDropFlags_SourceNoPreviewTooltip = 1 << 0, // By default, a successful call to BeginDragDropSource opens a tooltip so you can display a preview or description of the source contents. This flag disables this behavior.
|
||||
ImGuiDragDropFlags_SourceNoDisableHover = 1 << 1, // By default, when dragging we clear data so that IsItemHovered() will return false, to avoid subsequent user code submitting tooltips. This flag disables this behavior so you can still call IsItemHovered() on the source item.
|
||||
ImGuiDragDropFlags_SourceNoHoldToOpenOthers = 1 << 2, // Disable the behavior that allows to open tree nodes and collapsing header by holding over them while dragging a source item.
|
||||
ImGuiDragDropFlags_SourceAllowNullID = 1 << 3, // Allow items such as Text(), Image() that have no unique identifier to be used as drag source, by manufacturing a temporary identifier based on their window-relative position. This is extremely unusual within the dear imgui ecosystem and so we made it explicit.
|
||||
ImGuiDragDropFlags_SourceExtern = 1 << 4, // External source (from outside of dear imgui), won't attempt to read current item/window info. Will always return true. Only one Extern source can be active simultaneously.
|
||||
@ -1493,7 +1493,7 @@ enum ImGuiKey_
|
||||
// Keyboard Modifiers (explicitly submitted by backend via AddKeyEvent() calls)
|
||||
// - This is mirroring the data also written to io.KeyCtrl, io.KeyShift, io.KeyAlt, io.KeySuper, in a format allowing
|
||||
// them to be accessed via standard key API, allowing calls such as IsKeyPressed(), IsKeyReleased(), querying duration etc.
|
||||
// - Code polling every keys (e.g. an interface to detect a key press for input mapping) might want to ignore those
|
||||
// - Code polling every key (e.g. an interface to detect a key press for input mapping) might want to ignore those
|
||||
// and prefer using the real keys (e.g. ImGuiKey_LeftCtrl, ImGuiKey_RightCtrl instead of ImGuiKey_ModCtrl).
|
||||
// - In theory the value of keyboard modifiers should be roughly equivalent to a logical or of the equivalent left/right keys.
|
||||
// In practice: it's complicated; mods are often provided from different sources. Keyboard layout, IME, sticky keys and
|
||||
@ -1507,7 +1507,7 @@ enum ImGuiKey_
|
||||
// End of list
|
||||
ImGuiKey_COUNT, // No valid ImGuiKey is ever greater than this value
|
||||
|
||||
// [Internal] Prior to 1.87 we required user to fill io.KeysDown[512] using their own native index + a io.KeyMap[] array.
|
||||
// [Internal] Prior to 1.87 we required user to fill io.KeysDown[512] using their own native index + the io.KeyMap[] array.
|
||||
// We are ditching this method but keeping a legacy path for user code doing e.g. IsKeyPressed(MY_NATIVE_KEY_CODE)
|
||||
ImGuiKey_NamedKey_BEGIN = 512,
|
||||
ImGuiKey_NamedKey_END = ImGuiKey_COUNT,
|
||||
@ -1779,7 +1779,7 @@ enum ImGuiMouseCursor_
|
||||
ImGuiMouseCursor_Arrow = 0,
|
||||
ImGuiMouseCursor_TextInput, // When hovering over InputText, etc.
|
||||
ImGuiMouseCursor_ResizeAll, // (Unused by Dear ImGui functions)
|
||||
ImGuiMouseCursor_ResizeNS, // When hovering over an horizontal border
|
||||
ImGuiMouseCursor_ResizeNS, // When hovering over a horizontal border
|
||||
ImGuiMouseCursor_ResizeEW, // When hovering over a vertical border or a column
|
||||
ImGuiMouseCursor_ResizeNESW, // When hovering over the bottom-left corner of a window
|
||||
ImGuiMouseCursor_ResizeNWSE, // When hovering over the bottom-right corner of a window
|
||||
@ -1910,7 +1910,7 @@ struct ImGuiStyle
|
||||
ImVec2 WindowPadding; // Padding within a window.
|
||||
float WindowRounding; // Radius of window corners rounding. Set to 0.0f to have rectangular windows. Large values tend to lead to variety of artifacts and are not recommended.
|
||||
float WindowBorderSize; // Thickness of border around windows. Generally set to 0.0f or 1.0f. (Other values are not well tested and more CPU/GPU costly).
|
||||
ImVec2 WindowMinSize; // Minimum window size. This is a global setting. If you want to constraint individual windows, use SetNextWindowSizeConstraints().
|
||||
ImVec2 WindowMinSize; // Minimum window size. This is a global setting. If you want to constrain individual windows, use SetNextWindowSizeConstraints().
|
||||
ImVec2 WindowTitleAlign; // Alignment for title bar text. Defaults to (0.0f,0.5f) for left-aligned,vertically centered.
|
||||
ImGuiDir WindowMenuButtonPosition; // Side of the collapsing/docking button in the title bar (None/Left/Right). Defaults to ImGuiDir_Left.
|
||||
float ChildRounding; // Radius of child window corners rounding. Set to 0.0f to have rectangular windows.
|
||||
@ -1933,7 +1933,7 @@ struct ImGuiStyle
|
||||
float LogSliderDeadzone; // The size in pixels of the dead-zone around zero on logarithmic sliders that cross zero.
|
||||
float TabRounding; // Radius of upper corners of a tab. Set to 0.0f to have rectangular tabs.
|
||||
float TabBorderSize; // Thickness of border around tabs.
|
||||
float TabMinWidthForCloseButton; // Minimum width for close button to appears on an unselected tab when hovered. Set to 0.0f to always show when hovering, set to FLT_MAX to never show close button unless selected.
|
||||
float TabMinWidthForCloseButton; // Minimum width for close button to appear on an unselected tab when hovered. Set to 0.0f to always show when hovering, set to FLT_MAX to never show close button unless selected.
|
||||
ImGuiDir ColorButtonPosition; // Side of the color button in the ColorEdit4 widget (left/right). Defaults to ImGuiDir_Right.
|
||||
ImVec2 ButtonTextAlign; // Alignment of button text when button is larger than text. Defaults to (0.5f, 0.5f) (centered).
|
||||
ImVec2 SelectableTextAlign; // Alignment of selectable text. Defaults to (0.0f, 0.0f) (top-left aligned). It's generally important to keep this left-aligned if you want to lay multiple items on a same line.
|
||||
@ -2059,8 +2059,8 @@ struct ImGuiIO
|
||||
IMGUI_API void AddMouseViewportEvent(ImGuiID id); // Queue a mouse hovered viewport. Requires backend to set ImGuiBackendFlags_HasMouseHoveredViewport to call this (for multi-viewport support).
|
||||
IMGUI_API void AddFocusEvent(bool focused); // Queue a gain/loss of focus for the application (generally based on OS/platform focus of your window)
|
||||
IMGUI_API void AddInputCharacter(unsigned int c); // Queue a new character input
|
||||
IMGUI_API void AddInputCharacterUTF16(ImWchar16 c); // Queue a new character input from an UTF-16 character, it can be a surrogate
|
||||
IMGUI_API void AddInputCharactersUTF8(const char* str); // Queue a new characters input from an UTF-8 string
|
||||
IMGUI_API void AddInputCharacterUTF16(ImWchar16 c); // Queue a new character input from a UTF-16 character, it can be a surrogate
|
||||
IMGUI_API void AddInputCharactersUTF8(const char* str); // Queue a new characters input from a UTF-8 string
|
||||
|
||||
IMGUI_API void SetKeyEventNativeData(ImGuiKey key, int native_keycode, int native_scancode, int native_legacy_index = -1); // [Optional] Specify index for legacy <1.87 IsKeyXXX() functions with native indices + specify native keycode, scancode.
|
||||
IMGUI_API void SetAppAcceptingEvents(bool accepting_events); // Set master flag for accepting key/mouse/text events (default to true). Useful if you have native dialog boxes that are interrupting your application loop/refresh, and you want to disable events being queued while your app is frozen.
|
||||
@ -2104,9 +2104,9 @@ struct ImGuiIO
|
||||
// (this block used to be written by backend, since 1.87 it is best to NOT write to those directly, call the AddXXX functions above instead)
|
||||
// (reading from those variables is fair game, as they are extremely unlikely to be moving anywhere)
|
||||
ImVec2 MousePos; // Mouse position, in pixels. Set to ImVec2(-FLT_MAX, -FLT_MAX) if mouse is unavailable (on another screen, etc.)
|
||||
bool MouseDown[5]; // Mouse buttons: 0=left, 1=right, 2=middle + extras (ImGuiMouseButton_COUNT == 5). Dear ImGui mostly uses left and right buttons. Others buttons allows us to track if the mouse is being used by your application + available to user as a convenience via IsMouse** API.
|
||||
bool MouseDown[5]; // Mouse buttons: 0=left, 1=right, 2=middle + extras (ImGuiMouseButton_COUNT == 5). Dear ImGui mostly uses left and right buttons. Other buttons allow us to track if the mouse is being used by your application + available to user as a convenience via IsMouse** API.
|
||||
float MouseWheel; // Mouse wheel Vertical: 1 unit scrolls about 5 lines text.
|
||||
float MouseWheelH; // Mouse wheel Horizontal. Most users don't have a mouse with an horizontal wheel, may not be filled by all backends.
|
||||
float MouseWheelH; // Mouse wheel Horizontal. Most users don't have a mouse with a horizontal wheel, may not be filled by all backends.
|
||||
ImGuiID MouseHoveredViewport; // (Optional) Modify using io.AddMouseViewportEvent(). With multi-viewports: viewport the OS mouse is hovering. If possible _IGNORING_ viewports with the ImGuiViewportFlags_NoInputs flag is much better (few backends can handle that). Set io.BackendFlags |= ImGuiBackendFlags_HasMouseHoveredViewport if you can provide this info. If you don't imgui will infer the value using the rectangles and last focused time of the viewports it knows about (ignoring other OS windows).
|
||||
bool KeyCtrl; // Keyboard modifier down: Control
|
||||
bool KeyShift; // Keyboard modifier down: Shift
|
||||
@ -2273,7 +2273,7 @@ struct ImGuiTableSortSpecs
|
||||
#define IM_UNICODE_CODEPOINT_MAX 0xFFFF // Maximum Unicode code point supported by this build.
|
||||
#endif
|
||||
|
||||
// Helper: Execute a block of code at maximum once a frame. Convenient if you want to quickly create an UI within deep-nested code that runs multiple times every frame.
|
||||
// Helper: Execute a block of code at maximum once a frame. Convenient if you want to quickly create a UI within deep-nested code that runs multiple times every frame.
|
||||
// Usage: static ImGuiOnceUponAFrame oaf; if (oaf) ImGui::Text("This will be called only once per frame");
|
||||
struct ImGuiOnceUponAFrame
|
||||
{
|
||||
@ -2381,7 +2381,7 @@ struct ImGuiStorage
|
||||
};
|
||||
|
||||
// Helper: Manually clip large list of items.
|
||||
// If you have lots evenly spaced items and you have a random access to the list, you can perform coarse
|
||||
// If you have lots evenly spaced items and you have random access to the list, you can perform coarse
|
||||
// clipping based on visibility to only submit items that are in view.
|
||||
// The clipper calculates the range of visible items and advance the cursor to compensate for the non-visible items we have skipped.
|
||||
// (Dear ImGui already clip items based on their bounds but: it needs to first layout the item to do so, and generally
|
||||
@ -2527,7 +2527,7 @@ struct ImDrawVert
|
||||
#else
|
||||
// You can override the vertex format layout by defining IMGUI_OVERRIDE_DRAWVERT_STRUCT_LAYOUT in imconfig.h
|
||||
// The code expect ImVec2 pos (8 bytes), ImVec2 uv (8 bytes), ImU32 col (4 bytes), but you can re-order them or add other fields as needed to simplify integration in your engine.
|
||||
// The type has to be described within the macro (you can either declare the struct or use a typedef). This is because ImVec2/ImU32 are likely not declared a the time you'd want to set your type up.
|
||||
// The type has to be described within the macro (you can either declare the struct or use a typedef). This is because ImVec2/ImU32 are likely not declared at the time you'd want to set your type up.
|
||||
// NOTE: IMGUI DOESN'T CLEAR THE STRUCTURE AND DOESN'T CALL A CONSTRUCTOR SO ANY CUSTOM FIELD WILL BE UNINITIALIZED. IF YOU ADD EXTRA FIELDS (SUCH AS A 'Z' COORDINATES) YOU WILL NEED TO CLEAR THEM DURING RENDER OR TO IGNORE THEM.
|
||||
IMGUI_OVERRIDE_DRAWVERT_STRUCT_LAYOUT;
|
||||
#endif
|
||||
@ -2644,7 +2644,7 @@ struct ImDrawList
|
||||
// - For circle primitives, use "num_segments == 0" to automatically calculate tessellation (preferred).
|
||||
// In older versions (until Dear ImGui 1.77) the AddCircle functions defaulted to num_segments == 12.
|
||||
// In future versions we will use textures to provide cheaper and higher-quality circles.
|
||||
// Use AddNgon() and AddNgonFilled() functions if you need to guaranteed a specific number of sides.
|
||||
// Use AddNgon() and AddNgonFilled() functions if you need to guarantee a specific number of sides.
|
||||
IMGUI_API void AddLine(const ImVec2& p1, const ImVec2& p2, ImU32 col, float thickness = 1.0f);
|
||||
IMGUI_API void AddRect(const ImVec2& p_min, const ImVec2& p_max, ImU32 col, float rounding = 0.0f, ImDrawFlags flags = 0, float thickness = 1.0f); // a: upper-left, b: lower-right (== upper-left + size)
|
||||
IMGUI_API void AddRectFilled(const ImVec2& p_min, const ImVec2& p_max, ImU32 col, float rounding = 0.0f, ImDrawFlags flags = 0); // a: upper-left, b: lower-right (== upper-left + size)
|
||||
@ -2849,7 +2849,7 @@ enum ImFontAtlasFlags_
|
||||
// - Important: By default, AddFontFromMemoryTTF() takes ownership of the data. Even though we are not writing to it, we will free the pointer on destruction.
|
||||
// You can set font_cfg->FontDataOwnedByAtlas=false to keep ownership of your data and it won't be freed,
|
||||
// - Even though many functions are suffixed with "TTF", OTF data is supported just as well.
|
||||
// - This is an old API and it is currently awkward for those and and various other reasons! We will address them in the future!
|
||||
// - This is an old API and it is currently awkward for those and various other reasons! We will address them in the future!
|
||||
struct ImFontAtlas
|
||||
{
|
||||
IMGUI_API ImFontAtlas();
|
||||
@ -2873,7 +2873,7 @@ struct ImFontAtlas
|
||||
IMGUI_API bool Build(); // Build pixels data. This is called automatically for you by the GetTexData*** functions.
|
||||
IMGUI_API void GetTexDataAsAlpha8(unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel = NULL); // 1 byte per-pixel
|
||||
IMGUI_API void GetTexDataAsRGBA32(unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel = NULL); // 4 bytes-per-pixel
|
||||
bool IsBuilt() const { return Fonts.Size > 0 && TexReady; } // Bit ambiguous: used to detect when user didn't built texture but effectively we should check TexID != 0 except that would be backend dependent...
|
||||
bool IsBuilt() const { return Fonts.Size > 0 && TexReady; } // Bit ambiguous: used to detect when user didn't build texture but effectively we should check TexID != 0 except that would be backend dependent...
|
||||
void SetTexID(ImTextureID id) { TexID = id; }
|
||||
|
||||
//-------------------------------------------
|
||||
|
157
imgui_demo.cpp
157
imgui_demo.cpp
@ -52,7 +52,7 @@ Index of this file:
|
||||
// - sub section: ShowDemoWindowLayout()
|
||||
// - sub section: ShowDemoWindowPopups()
|
||||
// - sub section: ShowDemoWindowTables()
|
||||
// - sub section: ShowDemoWindowMisc()
|
||||
// - sub section: ShowDemoWindowInputs()
|
||||
// [SECTION] About Window / ShowAboutWindow()
|
||||
// [SECTION] Style Editor / ShowStyleEditor()
|
||||
// [SECTION] Example App: Main Menu Bar / ShowExampleAppMainMenuBar()
|
||||
@ -95,7 +95,7 @@ Index of this file:
|
||||
#ifdef _MSC_VER
|
||||
#pragma warning (disable: 4127) // condition expression is constant
|
||||
#pragma warning (disable: 4996) // 'This function or variable may be unsafe': strcpy, strdup, sprintf, vsnprintf, sscanf, fopen
|
||||
#pragma warning (disable: 26451) // [Static Analyzer] Arithmetic overflow : Using operator 'xxx' on a 4 byte value and then casting the result to a 8 byte value. Cast the value to the wider type before calling operator 'xxx' to avoid overflow(io.2).
|
||||
#pragma warning (disable: 26451) // [Static Analyzer] Arithmetic overflow : Using operator 'xxx' on a 4 byte value and then casting the result to an 8 byte value. Cast the value to the wider type before calling operator 'xxx' to avoid overflow(io.2).
|
||||
#endif
|
||||
|
||||
// Clang/GCC warnings with -Weverything
|
||||
@ -213,7 +213,7 @@ static void ShowDockingDisabledMessage()
|
||||
io.ConfigFlags |= ImGuiConfigFlags_DockingEnable;
|
||||
}
|
||||
|
||||
// Helper to wire demo markers located in code to a interactive browser
|
||||
// Helper to wire demo markers located in code to an interactive browser
|
||||
typedef void (*ImGuiDemoMarkerCallback)(const char* file, int line, const char* section, void* user_data);
|
||||
extern ImGuiDemoMarkerCallback GImGuiDemoMarkerCallback;
|
||||
extern void* GImGuiDemoMarkerCallbackUserData;
|
||||
@ -260,7 +260,7 @@ void ImGui::ShowUserGuide()
|
||||
// - ShowDemoWindowPopups()
|
||||
// - ShowDemoWindowTables()
|
||||
// - ShowDemoWindowColumns()
|
||||
// - ShowDemoWindowMisc()
|
||||
// - ShowDemoWindowInputs()
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
// We split the contents of the big ShowDemoWindow() function into smaller functions
|
||||
@ -270,7 +270,7 @@ static void ShowDemoWindowLayout();
|
||||
static void ShowDemoWindowPopups();
|
||||
static void ShowDemoWindowTables();
|
||||
static void ShowDemoWindowColumns();
|
||||
static void ShowDemoWindowMisc();
|
||||
static void ShowDemoWindowInputs();
|
||||
|
||||
// Demonstrate most Dear ImGui features (this is big function!)
|
||||
// You may execute this function to experiment with the UI and understand what it does.
|
||||
@ -617,7 +617,7 @@ void ImGui::ShowDemoWindow(bool* p_open)
|
||||
ShowDemoWindowLayout();
|
||||
ShowDemoWindowPopups();
|
||||
ShowDemoWindowTables();
|
||||
ShowDemoWindowMisc();
|
||||
ShowDemoWindowInputs();
|
||||
|
||||
// End of ShowDemoWindow()
|
||||
ImGui::PopItemWidth();
|
||||
@ -715,7 +715,7 @@ static void ShowDemoWindowWidgets()
|
||||
"USER:\n"
|
||||
"Hold SHIFT or use mouse to select text.\n"
|
||||
"CTRL+Left/Right to word jump.\n"
|
||||
"CTRL+A or double-click to select all.\n"
|
||||
"CTRL+A or Double-Click to select all.\n"
|
||||
"CTRL+X,CTRL+C,CTRL+V clipboard.\n"
|
||||
"CTRL+Z,CTRL+Y undo/redo.\n"
|
||||
"ESCAPE to revert.\n\n"
|
||||
@ -1066,7 +1066,7 @@ static void ShowDemoWindowWidgets()
|
||||
// Note that characters values are preserved even by InputText() if the font cannot be displayed,
|
||||
// so you can safely copy & paste garbled characters into another application.
|
||||
ImGui::TextWrapped(
|
||||
"CJK text will only appears if the font was loaded with the appropriate CJK character ranges. "
|
||||
"CJK text will only appear if the font was loaded with the appropriate CJK character ranges. "
|
||||
"Call io.Fonts->AddFontFromFileTTF() manually to load extra character ranges. "
|
||||
"Read docs/FONTS.md for details.");
|
||||
ImGui::Text("Hiragana: \xe3\x81\x8b\xe3\x81\x8d\xe3\x81\x8f\xe3\x81\x91\xe3\x81\x93 (kakikukeko)"); // Normally we would use u8"blah blah" with the proper characters directly in the string.
|
||||
@ -1529,7 +1529,7 @@ static void ShowDemoWindowWidgets()
|
||||
static char buf3[64];
|
||||
static int edit_count = 0;
|
||||
ImGui::InputText("Edit", buf3, 64, ImGuiInputTextFlags_CallbackEdit, Funcs::MyCallback, (void*)&edit_count);
|
||||
ImGui::SameLine(); HelpMarker("Here we toggle the casing of the first character on every edits + count edits.");
|
||||
ImGui::SameLine(); HelpMarker("Here we toggle the casing of the first character on every edit + count edits.");
|
||||
ImGui::SameLine(); ImGui::Text("(%d)", edit_count);
|
||||
|
||||
ImGui::TreePop();
|
||||
@ -2054,7 +2054,7 @@ static void ShowDemoWindowWidgets()
|
||||
// - integer/float/double
|
||||
// To avoid polluting the public API with all possible combinations, we use the ImGuiDataType enum
|
||||
// to pass the type, and passing all arguments by pointer.
|
||||
// This is the reason the test code below creates local variables to hold "zero" "one" etc. for each types.
|
||||
// This is the reason the test code below creates local variables to hold "zero" "one" etc. for each type.
|
||||
// In practice, if you frequently use a given type that is not covered by the normal API entry points,
|
||||
// you can wrap it yourself inside a 1 line function which can take typed argument as value instead of void*,
|
||||
// and then pass their address to the generic function. For example:
|
||||
@ -2099,7 +2099,7 @@ static void ShowDemoWindowWidgets()
|
||||
ImGui::Text("Drags:");
|
||||
ImGui::Checkbox("Clamp integers to 0..50", &drag_clamp);
|
||||
ImGui::SameLine(); HelpMarker(
|
||||
"As with every widgets in dear imgui, we never modify values unless there is a user interaction.\n"
|
||||
"As with every widget in dear imgui, we never modify values unless there is a user interaction.\n"
|
||||
"You can override the clamping limits by using CTRL+Click to input a value.");
|
||||
ImGui::DragScalar("drag s8", ImGuiDataType_S8, &s8_v, drag_speed, drag_clamp ? &s8_zero : NULL, drag_clamp ? &s8_fifty : NULL);
|
||||
ImGui::DragScalar("drag u8", ImGuiDataType_U8, &u8_v, drag_speed, drag_clamp ? &u8_zero : NULL, drag_clamp ? &u8_fifty : NULL, "%u ms");
|
||||
@ -2398,7 +2398,7 @@ static void ShowDemoWindowWidgets()
|
||||
HelpMarker("Testing how various types of items are interacting with the IsItemXXX functions. Note that the bool return value of most ImGui function is generally equivalent to calling ImGui::IsItemHovered().");
|
||||
ImGui::Checkbox("Item Disabled", &item_disabled);
|
||||
|
||||
// Submit selected item item so we can query their status in the code following it.
|
||||
// Submit selected items so we can query their status in the code following it.
|
||||
bool ret = false;
|
||||
static bool b = false;
|
||||
static float col4f[4] = { 1.0f, 0.5, 0.0f, 1.0f };
|
||||
@ -2591,6 +2591,26 @@ static void ShowDemoWindowWidgets()
|
||||
ImGui::SameLine(); HelpMarker("Demonstrate using BeginDisabled()/EndDisabled() across this section.");
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
IMGUI_DEMO_MARKER("Widgets/Text Filter");
|
||||
if (ImGui::TreeNode("Text Filter"))
|
||||
{
|
||||
// Helper class to easy setup a text filter.
|
||||
// You may want to implement a more feature-full filtering scheme in your own application.
|
||||
HelpMarker("Not a widget per-se, but ImGuiTextFilter is a helper to perform simple filtering on text strings.");
|
||||
static ImGuiTextFilter filter;
|
||||
ImGui::Text("Filter usage:\n"
|
||||
" \"\" display all lines\n"
|
||||
" \"xxx\" display lines containing \"xxx\"\n"
|
||||
" \"xxx,yyy\" display lines containing \"xxx\" or \"yyy\"\n"
|
||||
" \"-xxx\" hide lines containing \"xxx\"");
|
||||
filter.Draw();
|
||||
const char* lines[] = { "aaa1.c", "bbb1.c", "ccc1.c", "aaa2.cpp", "bbb2.cpp", "ccc2.cpp", "abc.h", "hello, world" };
|
||||
for (int i = 0; i < IM_ARRAYSIZE(lines); i++)
|
||||
if (filter.PassFilter(lines[i]))
|
||||
ImGui::BulletText("%s", lines[i]);
|
||||
ImGui::TreePop();
|
||||
}
|
||||
}
|
||||
|
||||
static void ShowDemoWindowLayout()
|
||||
@ -3505,7 +3525,7 @@ static void ShowDemoWindowPopups()
|
||||
// if (IsItemHovered() && IsMouseReleased(ImGuiMouseButton_Right))
|
||||
// OpenPopup(id);
|
||||
// return BeginPopup(id);
|
||||
// For advanced advanced uses you may want to replicate and customize this code.
|
||||
// For advanced uses you may want to replicate and customize this code.
|
||||
// See more details in BeginPopupContextItem().
|
||||
|
||||
// Example 1
|
||||
@ -3673,7 +3693,7 @@ static void ShowDemoWindowPopups()
|
||||
}
|
||||
|
||||
// Dummy data structure that we use for the Table demo.
|
||||
// (pre-C++11 doesn't allow us to instantiate ImVector<MyItem> template if this structure if defined inside the demo function)
|
||||
// (pre-C++11 doesn't allow us to instantiate ImVector<MyItem> template if this structure is defined inside the demo function)
|
||||
namespace
|
||||
{
|
||||
// We are passing our own identifier to TableSetupColumn() to facilitate identifying columns in the sorting code.
|
||||
@ -3892,7 +3912,7 @@ static void ShowDemoWindowTables()
|
||||
}
|
||||
|
||||
// [Method 2] Using TableNextColumn() called multiple times, instead of using a for loop + TableSetColumnIndex().
|
||||
// This is generally more convenient when you have code manually submitting the contents of each columns.
|
||||
// This is generally more convenient when you have code manually submitting the contents of each column.
|
||||
HelpMarker("Using TableNextRow() + calling TableNextColumn() _before_ each cell, manually.");
|
||||
if (ImGui::BeginTable("table2", 3))
|
||||
{
|
||||
@ -3913,7 +3933,7 @@ static void ShowDemoWindowTables()
|
||||
// as TableNextColumn() will automatically wrap around and create new rows as needed.
|
||||
// This is generally more convenient when your cells all contains the same type of data.
|
||||
HelpMarker(
|
||||
"Only using TableNextColumn(), which tends to be convenient for tables where every cells contains the same type of contents.\n"
|
||||
"Only using TableNextColumn(), which tends to be convenient for tables where every cell contains the same type of contents.\n"
|
||||
"This is also more similar to the old NextColumn() function of the Columns API, and provided to facilitate the Columns->Tables API transition.");
|
||||
if (ImGui::BeginTable("table3", 3))
|
||||
{
|
||||
@ -3965,7 +3985,7 @@ static void ShowDemoWindowTables()
|
||||
ImGui::SameLine(); ImGui::RadioButton("Text", &contents_type, CT_Text);
|
||||
ImGui::SameLine(); ImGui::RadioButton("FillButton", &contents_type, CT_FillButton);
|
||||
ImGui::Checkbox("Display headers", &display_headers);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBody", &flags, ImGuiTableFlags_NoBordersInBody); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body (borders will always appears in Headers");
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBody", &flags, ImGuiTableFlags_NoBordersInBody); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body (borders will always appear in Headers");
|
||||
PopStyleCompact();
|
||||
|
||||
if (ImGui::BeginTable("table1", 3, flags))
|
||||
@ -4004,8 +4024,8 @@ static void ShowDemoWindowTables()
|
||||
IMGUI_DEMO_MARKER("Tables/Resizable, stretch");
|
||||
if (ImGui::TreeNode("Resizable, stretch"))
|
||||
{
|
||||
// By default, if we don't enable ScrollX the sizing policy for each columns is "Stretch"
|
||||
// Each columns maintain a sizing weight, and they will occupy all available width.
|
||||
// By default, if we don't enable ScrollX the sizing policy for each column is "Stretch"
|
||||
// All columns maintain a sizing weight, and they will occupy all available width.
|
||||
static ImGuiTableFlags flags = ImGuiTableFlags_SizingStretchSame | ImGuiTableFlags_Resizable | ImGuiTableFlags_BordersOuter | ImGuiTableFlags_BordersV | ImGuiTableFlags_ContextMenuInBody;
|
||||
PushStyleCompact();
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_Resizable", &flags, ImGuiTableFlags_Resizable);
|
||||
@ -4127,7 +4147,7 @@ static void ShowDemoWindowTables()
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_Reorderable", &flags, ImGuiTableFlags_Reorderable);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_Hideable", &flags, ImGuiTableFlags_Hideable);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBody", &flags, ImGuiTableFlags_NoBordersInBody);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBodyUntilResize", &flags, ImGuiTableFlags_NoBordersInBodyUntilResize); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body until hovered for resize (borders will always appears in Headers)");
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBodyUntilResize", &flags, ImGuiTableFlags_NoBordersInBodyUntilResize); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body until hovered for resize (borders will always appear in Headers)");
|
||||
PopStyleCompact();
|
||||
|
||||
if (ImGui::BeginTable("table1", 3, flags))
|
||||
@ -4185,7 +4205,7 @@ static void ShowDemoWindowTables()
|
||||
"- any form of row selection\n"
|
||||
"Because of this, activating BorderOuterV sets the default to PadOuterX. Using PadOuterX or NoPadOuterX you can override the default.\n\n"
|
||||
"Actual padding values are using style.CellPadding.\n\n"
|
||||
"In this demo we don't show horizontal borders to emphasis how they don't affect default horizontal padding.");
|
||||
"In this demo we don't show horizontal borders to emphasize how they don't affect default horizontal padding.");
|
||||
|
||||
static ImGuiTableFlags flags1 = ImGuiTableFlags_BordersV;
|
||||
PushStyleCompact();
|
||||
@ -4654,7 +4674,7 @@ static void ShowDemoWindowTables()
|
||||
IMGUI_DEMO_MARKER("Tables/Nested tables");
|
||||
if (ImGui::TreeNode("Nested tables"))
|
||||
{
|
||||
HelpMarker("This demonstrate embedding a table into another table cell.");
|
||||
HelpMarker("This demonstrates embedding a table into another table cell.");
|
||||
|
||||
if (ImGui::BeginTable("table_nested1", 2, ImGuiTableFlags_Borders | ImGuiTableFlags_Resizable | ImGuiTableFlags_Reorderable | ImGuiTableFlags_Hideable))
|
||||
{
|
||||
@ -4699,7 +4719,7 @@ static void ShowDemoWindowTables()
|
||||
IMGUI_DEMO_MARKER("Tables/Row height");
|
||||
if (ImGui::TreeNode("Row height"))
|
||||
{
|
||||
HelpMarker("You can pass a 'min_row_height' to TableNextRow().\n\nRows are padded with 'style.CellPadding.y' on top and bottom, so effectively the minimum row height will always be >= 'style.CellPadding.y * 2.0f'.\n\nWe cannot honor a _maximum_ row height as that would requires a unique clipping rectangle per row.");
|
||||
HelpMarker("You can pass a 'min_row_height' to TableNextRow().\n\nRows are padded with 'style.CellPadding.y' on top and bottom, so effectively the minimum row height will always be >= 'style.CellPadding.y * 2.0f'.\n\nWe cannot honor a _maximum_ row height as that would require a unique clipping rectangle per row.");
|
||||
if (ImGui::BeginTable("table_row_height", 1, ImGuiTableFlags_BordersOuter | ImGuiTableFlags_BordersInnerV))
|
||||
{
|
||||
for (int row = 0; row < 10; row++)
|
||||
@ -5261,7 +5281,7 @@ static void ShowDemoWindowTables()
|
||||
static bool show_headers = true;
|
||||
static bool show_wrapped_text = false;
|
||||
//static ImGuiTextFilter filter;
|
||||
//ImGui::SetNextItemOpen(true, ImGuiCond_Once); // FIXME-TABLE: Enabling this results in initial clipped first pass on table which tend to affects column sizing
|
||||
//ImGui::SetNextItemOpen(true, ImGuiCond_Once); // FIXME-TABLE: Enabling this results in initial clipped first pass on table which tend to affect column sizing
|
||||
if (ImGui::TreeNode("Options"))
|
||||
{
|
||||
// Make the UI compact because there are so many fields
|
||||
@ -5288,8 +5308,8 @@ static void ShowDemoWindowTables()
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_BordersH", &flags, ImGuiTableFlags_BordersH);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_BordersOuterH", &flags, ImGuiTableFlags_BordersOuterH);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_BordersInnerH", &flags, ImGuiTableFlags_BordersInnerH);
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBody", &flags, ImGuiTableFlags_NoBordersInBody); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body (borders will always appears in Headers");
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBodyUntilResize", &flags, ImGuiTableFlags_NoBordersInBodyUntilResize); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body until hovered for resize (borders will always appears in Headers)");
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBody", &flags, ImGuiTableFlags_NoBordersInBody); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body (borders will always appear in Headers");
|
||||
ImGui::CheckboxFlags("ImGuiTableFlags_NoBordersInBodyUntilResize", &flags, ImGuiTableFlags_NoBordersInBodyUntilResize); ImGui::SameLine(); HelpMarker("Disable vertical borders in columns Body until hovered for resize (borders will always appear in Headers)");
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
@ -5352,7 +5372,7 @@ static void ShowDemoWindowTables()
|
||||
HelpMarker("If scrolling is disabled (ScrollX and ScrollY not set):\n"
|
||||
"- The table is output directly in the parent window.\n"
|
||||
"- OuterSize.x < 0.0f will right-align the table.\n"
|
||||
"- OuterSize.x = 0.0f will narrow fit the table unless there are any Stretch column.\n"
|
||||
"- OuterSize.x = 0.0f will narrow fit the table unless there are any Stretch columns.\n"
|
||||
"- OuterSize.y then becomes the minimum size for the table, which will extend vertically if there are more rows (unless NoHostExtendY is set).");
|
||||
|
||||
// From a user point of view we will tend to use 'inner_width' differently depending on whether our table is embedding scrolling.
|
||||
@ -5759,26 +5779,8 @@ static void ShowDemoWindowColumns()
|
||||
|
||||
namespace ImGui { extern ImGuiKeyData* GetKeyData(ImGuiKey key); }
|
||||
|
||||
static void ShowDemoWindowMisc()
|
||||
static void ShowDemoWindowInputs()
|
||||
{
|
||||
IMGUI_DEMO_MARKER("Filtering");
|
||||
if (ImGui::CollapsingHeader("Filtering"))
|
||||
{
|
||||
// Helper class to easy setup a text filter.
|
||||
// You may want to implement a more feature-full filtering scheme in your own application.
|
||||
static ImGuiTextFilter filter;
|
||||
ImGui::Text("Filter usage:\n"
|
||||
" \"\" display all lines\n"
|
||||
" \"xxx\" display lines containing \"xxx\"\n"
|
||||
" \"xxx,yyy\" display lines containing \"xxx\" or \"yyy\"\n"
|
||||
" \"-xxx\" hide lines containing \"xxx\"");
|
||||
filter.Draw();
|
||||
const char* lines[] = { "aaa1.c", "bbb1.c", "ccc1.c", "aaa2.cpp", "bbb2.cpp", "ccc2.cpp", "abc.h", "hello, world" };
|
||||
for (int i = 0; i < IM_ARRAYSIZE(lines); i++)
|
||||
if (filter.PassFilter(lines[i]))
|
||||
ImGui::BulletText("%s", lines[i]);
|
||||
}
|
||||
|
||||
IMGUI_DEMO_MARKER("Inputs, Navigation & Focus");
|
||||
if (ImGui::CollapsingHeader("Inputs, Navigation & Focus"))
|
||||
{
|
||||
@ -5817,11 +5819,40 @@ static void ShowDemoWindowMisc()
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
// Display mouse cursors
|
||||
IMGUI_DEMO_MARKER("Inputs, Navigation & Focus/Mouse Cursors");
|
||||
if (ImGui::TreeNode("Mouse Cursors"))
|
||||
{
|
||||
const char* mouse_cursors_names[] = { "Arrow", "TextInput", "ResizeAll", "ResizeNS", "ResizeEW", "ResizeNESW", "ResizeNWSE", "Hand", "NotAllowed" };
|
||||
IM_ASSERT(IM_ARRAYSIZE(mouse_cursors_names) == ImGuiMouseCursor_COUNT);
|
||||
|
||||
ImGuiMouseCursor current = ImGui::GetMouseCursor();
|
||||
ImGui::Text("Current mouse cursor = %d: %s", current, mouse_cursors_names[current]);
|
||||
ImGui::BeginDisabled(true);
|
||||
ImGui::CheckboxFlags("io.BackendFlags: HasMouseCursors", &io.BackendFlags, ImGuiBackendFlags_HasMouseCursors);
|
||||
ImGui::EndDisabled();
|
||||
|
||||
ImGui::Text("Hover to see mouse cursors:");
|
||||
ImGui::SameLine(); HelpMarker(
|
||||
"Your application can render a different mouse cursor based on what ImGui::GetMouseCursor() returns. "
|
||||
"If software cursor rendering (io.MouseDrawCursor) is set ImGui will draw the right cursor for you, "
|
||||
"otherwise your backend needs to handle it.");
|
||||
for (int i = 0; i < ImGuiMouseCursor_COUNT; i++)
|
||||
{
|
||||
char label[32];
|
||||
sprintf(label, "Mouse cursor %d: %s", i, mouse_cursors_names[i]);
|
||||
ImGui::Bullet(); ImGui::Selectable(label, false);
|
||||
if (ImGui::IsItemHovered())
|
||||
ImGui::SetMouseCursor(i);
|
||||
}
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
// Display Keyboard/Mouse state
|
||||
IMGUI_DEMO_MARKER("Inputs, Navigation & Focus/Keyboard, Gamepad & Navigation State");
|
||||
if (ImGui::TreeNode("Keyboard, Gamepad & Navigation State"))
|
||||
{
|
||||
// We iterate both legacy native range and named ImGuiKey ranges, which is a little odd but this allow displaying the data for old/new backends.
|
||||
// We iterate both legacy native range and named ImGuiKey ranges, which is a little odd but this allows displaying the data for old/new backends.
|
||||
// User code should never have to go through such hoops: old code may use native keycodes, new code may use ImGuiKey codes.
|
||||
#ifdef IMGUI_DISABLE_OBSOLETE_KEYIO
|
||||
struct funcs { static bool IsLegacyNativeDupe(ImGuiKey) { return false; } };
|
||||
@ -6009,30 +6040,6 @@ static void ShowDemoWindowMisc()
|
||||
ImGui::Text("io.MouseDelta: (%.1f, %.1f)", mouse_delta.x, mouse_delta.y);
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
IMGUI_DEMO_MARKER("Inputs, Navigation & Focus/Mouse cursors");
|
||||
if (ImGui::TreeNode("Mouse cursors"))
|
||||
{
|
||||
const char* mouse_cursors_names[] = { "Arrow", "TextInput", "ResizeAll", "ResizeNS", "ResizeEW", "ResizeNESW", "ResizeNWSE", "Hand", "NotAllowed" };
|
||||
IM_ASSERT(IM_ARRAYSIZE(mouse_cursors_names) == ImGuiMouseCursor_COUNT);
|
||||
|
||||
ImGuiMouseCursor current = ImGui::GetMouseCursor();
|
||||
ImGui::Text("Current mouse cursor = %d: %s", current, mouse_cursors_names[current]);
|
||||
ImGui::Text("Hover to see mouse cursors:");
|
||||
ImGui::SameLine(); HelpMarker(
|
||||
"Your application can render a different mouse cursor based on what ImGui::GetMouseCursor() returns. "
|
||||
"If software cursor rendering (io.MouseDrawCursor) is set ImGui will draw the right cursor for you, "
|
||||
"otherwise your backend needs to handle it.");
|
||||
for (int i = 0; i < ImGuiMouseCursor_COUNT; i++)
|
||||
{
|
||||
char label[32];
|
||||
sprintf(label, "Mouse cursor %d: %s", i, mouse_cursors_names[i]);
|
||||
ImGui::Bullet(); ImGui::Selectable(label, false);
|
||||
if (ImGui::IsItemHovered())
|
||||
ImGui::SetMouseCursor(i);
|
||||
}
|
||||
ImGui::TreePop();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
@ -7060,7 +7067,7 @@ struct ExampleAppLog
|
||||
if (Filter.IsActive())
|
||||
{
|
||||
// In this example we don't use the clipper when Filter is enabled.
|
||||
// This is because we don't have a random access on the result on our filter.
|
||||
// This is because we don't have random access to the result of our filter.
|
||||
// A real application processing logs with ten of thousands of entries may want to store the result of
|
||||
// search/filter.. especially if the filtering function is not trivial (e.g. reg-exp).
|
||||
for (int line_no = 0; line_no < LineOffsets.Size; line_no++)
|
||||
@ -7082,7 +7089,7 @@ struct ExampleAppLog
|
||||
// on your side is recommended. Using ImGuiListClipper requires
|
||||
// - A) random access into your data
|
||||
// - B) items all being the same height,
|
||||
// both of which we can handle since we an array pointing to the beginning of each line of text.
|
||||
// both of which we can handle since we have an array pointing to the beginning of each line of text.
|
||||
// When using the filter (in the block of code above) we don't have random access into the data to display
|
||||
// anymore, which is why we don't use the clipper. Storing or skimming through the search result would make
|
||||
// it possible (and would be recommended if you want to search through tens of thousands of entries).
|
||||
@ -7562,8 +7569,8 @@ static void ShowExampleAppFullscreen(bool* p_open)
|
||||
// [SECTION] Example App: Manipulating Window Titles / ShowExampleAppWindowTitles()
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
// Demonstrate using "##" and "###" in identifiers to manipulate ID generation.
|
||||
// This apply to all regular items as well.
|
||||
// Demonstrate the use of "##" and "###" in identifiers to manipulate ID generation.
|
||||
// This applies to all regular items as well.
|
||||
// Read FAQ section "How can I have multiple widgets with the same label?" for details.
|
||||
static void ShowExampleAppWindowTitles(bool*)
|
||||
{
|
||||
|
Loading…
Reference in New Issue
Block a user